Solitaire is a game played on a chessboard 8x8. The rows and columns of the chessboard are numbered from 1 to 8, from the top to the bottom and from left to right respectively.
There are four identical pieces on the board. In one move it is allowed to:
move a piece to an empty neighboring field (up, down, left or right),
jump over one neighboring piece to an empty field (up, down, left or right).
There are 4 moves allowed for each piece in the configuration shown above. As an example let’s consider a piece placed in the row 4, column 4. It can be moved one row up, two rows down, one column left or two columns right.
Write a program that:
reads two chessboard configurations from the standard input,
verifies whether the second one is reachable from the first one in at most 8 moves,
writes the result to the standard output.
Input
Each of two input lines contains 8 integers a1, a2, …, a8 separated by single spaces and describes one configuration of pieces on the chessboard. Integers a2j-1 and a2j (1 <= j <= 4) describe the position of one piece - the row number and the column number respectively. Process to the end of file.
Output
The output should contain one word for each test case - YES if a configuration described in the second input line is reachable from the configuration described in the first input line in at most 8 moves, or one word NO otherwise.
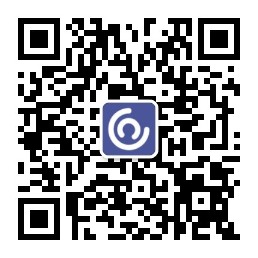
Sample Input
4 4 4 5 5 4 6 5
2 4 3 3 3 6 4 6
Sample Output
YES
Source
Southwestern Europe 2002
唉,还是太不仔细,要注意细节,有sort的时候很多东西都不能像以前那样写,下标会变,所以赋值和判断要紧跟着别的操作,可以用一个char数组记录所有的状态,int的话会mle。然后每次都sort之前判断一下,之后判断一下,就好了,注意一些细节,。
#include<bits/stdc++.h>
using namespace std;
char vis[8][8][8][8][8][8][8][8];
struct point
{
int x,y;
bool operator< (const point& a)const
{
if(x==a.x)
return y<a.y;
return x<a.x;
}
};
struct node
{
point p[5];
int step,id;
}sta,fin;
int xmov[]={1,0,-1,0};
int ymov[]={0,1,0,-1};
queue<node>Q;
int n=8,m=8;
int bfs()
{
while(!Q.empty())
{
node v,ne;
v=Q.front();
Q.pop();
for(int i=1;i<=4;i++)
{
for(int j=0;j<4;j++)
{
ne=v;
ne.p[i].x=v.p[i].x+xmov[j];
ne.p[i].y=v.p[i].y+ymov[j];
for(int k=1;k<=4;k++)
{
if(k==i)
continue;
if(ne.p[i].x==ne.p[k].x&&ne.p[i].y==ne.p[k].y)
{
ne.p[i].x=ne.p[i].x+xmov[j];
ne.p[i].y=ne.p[i].y+ymov[j];
break;
}
}
int flag=0;
if(ne.p[i].x<0||ne.p[i].x>=n||ne.p[i].y<0||ne.p[i].y>=m)
continue;
sort(ne.p+1,ne.p+5);
for(int k=2;k<=4;k++)
if(ne.p[k].x==ne.p[k-1].x&&ne.p[k].y==ne.p[k-1].y)
{
flag=1;
break;
}
if(flag)
continue;
if(vis[ne.p[1].x][ne.p[1].y][ne.p[2].x][ne.p[2].y][ne.p[3].x][ne.p[3].y][ne.p[4].x][ne.p[4].y]==ne.id)
continue;
if(vis[ne.p[1].x][ne.p[1].y][ne.p[2].x][ne.p[2].y][ne.p[3].x][ne.p[3].y][ne.p[4].x][ne.p[4].y]!=0)
{
return 1;
}
//printf("%d %d\n***\n",vis[ne.x[1]][ne.y[1]][ne.x[2]][ne.y[2]][ne.x[3]][ne.y[3]][ne.x[4]][ne.y[4]],ne.id);
vis[ne.p[1].x][ne.p[1].y][ne.p[2].x][ne.p[2].y][ne.p[3].x][ne.p[3].y][ne.p[4].x][ne.p[4].y]=ne.id;
ne.step=v.step+1;
if(ne.step<4)
{
Q.push(ne);
/*if(ne.id==1)
{for(int i=1;i<=4;i++)
printf("%d %d ",ne.p[i].x+1,ne.p[i].y+1);
printf("%d\n",ne.step);}*/
}
}
}
}
return 0;
}
int main()
{
while(scanf("%d%d",&sta.p[1].x,&sta.p[1].y)!=EOF)
{
sta.p[1].x-=1;
sta.p[1].y-=1;
memset(vis,0,sizeof(vis));
while(!Q.empty())
Q.pop();
for(int i=2;i<=4;i++)
{
scanf("%d%d",&sta.p[i].x,&sta.p[i].y);
sta.p[i].x-=1;
sta.p[i].y-=1;
}
sort(sta.p+1,sta.p+5);
sta.step=0;
vis[sta.p[1].x][sta.p[1].y][sta.p[2].x][sta.p[2].y][sta.p[3].x][sta.p[3].y][sta.p[4].x][sta.p[4].y]=1;
sta.id=1;
Q.push(sta);
for(int i=1;i<=4;i++)
{
scanf("%d%d",&fin.p[i].x,&fin.p[i].y);
fin.p[i].x-=1;
fin.p[i].y-=1;
}
sort(fin.p+1,fin.p+5);
fin.step=0;
vis[fin.p[1].x][fin.p[1].y][fin.p[2].x][fin.p[2].y][fin.p[3].x][fin.p[3].y][fin.p[4].x][fin.p[4].y]=2;
fin.id=2;
Q.push(fin);
int flag=0;
for(int i=1;i<=4;i++)
{
if(fin.p[i].x!=sta.p[i].x||fin.p[i].y!=sta.p[i].y)
{
flag=1;
break;
}
}
//printf("%d ",Q.size());
if(flag==0)
printf("YES\n");
else if(bfs()==1)
printf("YES\n");
else
printf("NO\n");
}
return 0;
}