There's a beach in the first quadrant. And from time to time, there are sea waves. A wave ( xx , yy ) means the wave is a rectangle whose vertexes are ( 00 , 00 ), ( xx , 00 ), ( 00 , yy ), ( xx , yy ). Every time the wave will wash out the trace of former wave in its range and remain its own trace of ( xx , 00 ) -> ( xx , yy ) and ( 00 , yy ) -> ( xx , yy ). Now the toad on the coast wants to know the total length of trace on the coast after n waves. It's guaranteed that a wave will not cover the other completely.
Input
The first line is the number of waves n(n \le 50000)n(n≤50000).
The next nn lines,each contains two numbers xx yy ,( 0 < x0<x , y \le 10000000y≤10000000 ),the ii-th line means the ii-th second there comes a wave of ( xx , yy ), it's guaranteed that when 1 \le i1≤i , j \le nj≤n ,x_i \le x_jxi≤xj and y_i \le y_jyi≤yj don't set up at the same time.
Output
An Integer stands for the answer.
Hint:
As for the sample input, the answer is 3+3+1+1+1+1=103+3+1+1+1+1=10
样例输入
3 1 4 4 1 3 3
样例输出
10
第一眼扫描线 想想突然感觉不太对,扫描线不会写
这题仔细分析一下 这题倒推更加容易写
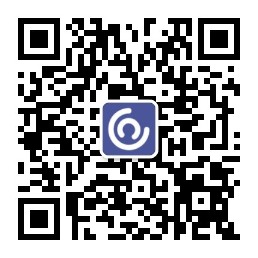
这题倒推的话只要更新比这个矩形更大的区域就行了
离散化一下
注意两个点
int ny = query( 1, L[i], tot, 1 );
int nx = query( 2, R[i], tot, 1 );
因为他给的是一个矩形所以我们看这条边能不能算就是看这个点的左边的最大值 所以query (L[I]->tot)
因为你只要左边有一条边 ,这是一个矩形 所以右边也一定有
第二个点
update( 1, L[i], sum[R[i] - 1], 1 );
update( 2, R[i], sum[L[i] - 1], 1 );
L[i] 表示X轴上的点 他的值对应的是 sum[R[i] - 1]
1 #include <cstdio> 2 #include <cstring> 3 #include <queue> 4 #include <cmath> 5 #include <algorithm> 6 #include <set> 7 #include <iostream> 8 #include <map> 9 #include <stack> 10 #include <string> 11 #include <vector> 12 #define pi acos(-1.0) 13 #define eps 1e-6 14 #define fi first 15 #define se second 16 #define lson l,m,rt<<1 17 #define rson m+1,r,rt<<1|1 18 #define rtl rt<<1 19 #define rtr rt<<1|1 20 #define bug printf("******\n") 21 #define mem(a,b) memset(a,b,sizeof(a)) 22 #define name2str(x) #x 23 #define fuck(x) cout<<#x" = "<<x<<endl 24 #define f(a) a*a 25 #define sf(n) scanf("%d", &n) 26 #define sff(a,b) scanf("%d %d", &a, &b) 27 #define sfff(a,b,c) scanf("%d %d %d", &a, &b, &c) 28 #define sffff(a,b,c,d) scanf("%d %d %d %d", &a, &b, &c, &d) 29 #define pf printf 30 #define FRE(i,a,b) for(i = a; i <= b; i++) 31 #define FREE(i,a,b) for(i = a; i >= b; i--) 32 #define FRL(i,a,b) for(i = a; i < b; i++) 33 #define FRLL(i,a,b) for(i = a; i > b; i--) 34 #define FIN freopen("in.txt","r",stdin) 35 #define gcd(a,b) __gcd(a,b) 36 #define lowbit(x) x&-x 37 using namespace std; 38 typedef long long LL; 39 typedef unsigned long long ULL; 40 const int INF = 0x7fffffff; 41 const int mod = 1e9 + 7; 42 const int maxn = 1e6 + 10; 43 struct node { 44 int l, r, sumx, sumy; 45 int mid() { 46 return ( l + r ) >> 1 ; 47 } 48 } tree[maxn << 2]; 49 void build( int l, int r, int rt ) { 50 tree[rt].l = l, tree[rt].r = r, tree[rt].sumx = tree[rt].sumy = 0; 51 if ( l == r ) return ; 52 int m = ( l + r ) >> 1; 53 build( l, m, rtl ); 54 build( m + 1, r, rtr ); 55 } 56 void update( int op, int pos, int key, int rt ) { 57 if ( tree[rt].l == tree[rt].r ) { 58 if ( op == 1 ) tree[rt].sumx = max( tree[rt].sumx, key ); 59 else tree[rt].sumy = max( tree[rt].sumy, key ); 60 return ; 61 } 62 int m = tree[rt].mid(); 63 if ( pos <= m ) update( op, pos, key, rtl ); 64 else update( op, pos, key, rtr ); 65 if ( op == 1 ) tree[rt].sumx = max( tree[rtl].sumx, tree[rtr].sumx ); 66 else tree[rt].sumy = max( tree[rtl].sumy, tree[rtr].sumy ); 67 } 68 int query( int op, int L, int R, int rt ) { 69 if ( L <= tree[rt].l && tree[rt].r <= R ) { 70 if ( op == 1 ) return tree[rt].sumx; 71 else return tree[rt].sumy; 72 } 73 int m = tree[rt].mid(); 74 if ( L > m ) return query( op, L, R, rtr ); 75 else if ( R <= m ) return query( op, L, R, rtl ); 76 else return max( query( op, L, m, rtl ), query( op, m + 1, R, rtr ) ); 77 } 78 int L[maxn], R[maxn], n, sum[maxn]; 79 int main() { 80 sf( n ); 81 int tot = 0; 82 for ( int i = 1 ; i <= n ; i++ ) { 83 sff( L[i], R[i] ); 84 sum[tot++] = L[i], sum[tot++] = R[i]; 85 } 86 sort( sum, sum + tot ); 87 tot = unique( sum, sum + tot ) - sum; 88 build( 1, tot, 1 ); 89 LL ans = 0; 90 for ( int i = n ; i >= 1 ; i-- ) { 91 int a = L[i], b = R[i]; 92 L[i] = lower_bound( sum, sum + tot, L[i] ) - sum + 1; 93 R[i] = lower_bound( sum, sum + tot, R[i] ) - sum + 1; 94 int ny = query( 1, L[i], tot, 1 ); 95 int nx = query( 2, R[i], tot, 1 ); 96 ans += b - ny; 97 ans += a - nx; 98 // fuck(nx),fuck(ny); 99 update( 1, L[i], sum[R[i] - 1], 1 ); 100 update( 2, R[i], sum[L[i] - 1], 1 ); 101 } 102 printf( "%lld\n", ans ); 103 return 0; 104 }