在项目中
有需求对后台传过来的List 对象,在jsp进行级联操作,写了几种实现的方式,
现保存实现步骤如下,以便日后使用。
=================================================
// 省list 省里有cities city里有areas
省 (id,name,cities) 市 (id,name,areas) 区(id,name)
========
一 、 异步获取:
=========================
JSP:
<div class="item"> <span><em>*</em>所在地区:</span> <span class="error-msg" id="areaNote"></span> <div class="fl"> <input type="hidden" id="provinceSet" value="${provinceList }"> <select id="provinceList" onchange="loadCity(this)" class="sele"> <option>请选择</option> <c:forEach var="province" items="${provinceList }"> <option value=${province.id }>${province.name }</option> </c:forEach> </select> <select id="cityList" onchange="loadArea()" class="sele"><option value="">请选择</option></select> <select id="areaList" name="deliveryaddress.area.id" class="sele"><option value="">请选择</option></select> </div> </div>js:
<script type="text/javascript"> /** * 获取二级列表 */ function loadCity() { var provinceId = $("#provinceList").find("option:selected").val(); jQuery.ajax( { type : "post", dataType : "json", url : "delivery_loadCity.action", data : "provinceId="+provinceId, cache : false, success : function(dataResult) { // 没有登录跳登录 if (isUserNotLogin(dataResult)) { goToLogin(); return; } var cityHtml = "<option value='0'>请选择</option>"; if(dataResult) { $.each(dataResult, function() { cityHtml += "<option value='" + this.id + "'>" + this.name + "</option>" ; } ); } $("#cityList").html(cityHtml); $("#areaList").html("<option value='0'>请选择</option>"); }, error : function(XMLHttpResponse) { return false; } }); } /** * 获取三级列表 */ function loadArea() { var cityId = $("#cityList").find("option:selected").val(); jQuery.ajax( { type : "post", dataType : "json", url : "delivery_loadArea.action", data : "cityId="+cityId, cache : false, success : function(dataResult) { // 没有登录跳登录 if (isUserNotLogin(dataResult)) { goToLogin(); return; } var areaHtml = "<option value='0'>请选择</option>"; if(dataResult) { $.each(dataResult, function() { areaHtml += "<option value='" + this.id + "'>" + this.name + "</option>" ; } ); } $("#areaList").html(areaHtml); }, error : function(XMLHttpResponse) { return false; } }); } </script>action:
public String loadCity() throws Exception { HttpServletRequest request = ServletActionContext.getRequest(); String provinceId = request.getParameter("provinceId"); Province province = provinceService.getById(Integer.valueOf(provinceId)); Set<City> citySet = province.getCities(); //把citySet集合转成Json传给页面 //只取得City的id和name属性 SimplePropertyPreFilter filter = new SimplePropertyPreFilter(); Set<String> set = filter.getIncludes(); set.add("id"); set.add("name"); writeHtml(JSONArray.toJSONString(citySet,filter)); return null; } public String loadArea() throws Exception { HttpServletRequest request = ServletActionContext.getRequest(); String cityId = request.getParameter("cityId"); City city = cityService.getById(Integer.valueOf(cityId)); Set<Area> areaSet = city.getAreas(); //把areaSet集合传给页面 //只选取Area的name和id和code属性 SimplePropertyPreFilter filter = new SimplePropertyPreFilter(); Set<String> set = filter.getIncludes(); set.add("id"); set.add("name"); writeHtml(JSONArray.toJSONString(areaSet,filter)); return null; } //返回strData结果给页面 protected void writeHtml(String strData) throws Exception { HttpServletResponse response = ServletActionContext.getResponse(); response.setContentType("application/json;charset=UTF-8"); response.setHeader("Cache-Control", "no-cache"); PrintWriter out = response.getWriter(); out.write(strData); out.flush(); out.close(); }
========
扫描二维码关注公众号,回复:
308300 查看本文章
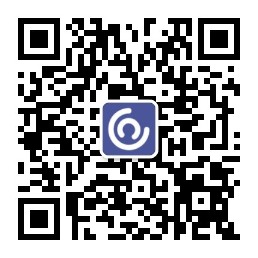
二 、 遍历Action的属性provinceList 获取:
=========================
jsp:在隐藏域保存遍历list<div class="fl"> <select id="provinceList" class="sele"> <option>请选择</option> <s:iterator value="provinceList" var="province"> <option value="<s:property value='id'/>"><s:property value="name"/></option> </s:iterator> </select> <select id="cityList" onchange="loadArea()" class="sele"><option value="">请选择</option></select> <select id="areaList" name="deliveryaddress.area.id" class="sele"><option value="">请选择</option></select> </div> <s:iterator value="provinceList" var="province"> <s:iterator value="cities" var="city"> <input type="hidden" name ="province_<s:property value='#province.id'/>" value="<s:property value='#city.name'/>_<s:property value='#city.id'/>"/> <s:iterator value="#city.areas" var="area"> <input type="hidden" name ="city_<s:property value='#city.id'/>" value="<s:property value='#area.name'/>_<s:property value='#area.id'/>"/> </s:iterator> </s:iterator> </s:iterator>js:
$(function(){ $("#provinceList").change(function (){ var value = $(this).val(); $("#cityList").html("<option value='0'>请选择</option>"); $("input[name^='province_"+value+"']").each(function(){ var val = $(this).val(); $("#cityList").append("<option value='"+val.split("_")[1]+"'>"+val.split("_")[0]+"</option>"); }); $("#areaList").html("<option value='0'>请选择</option>"); }); $("#cityList").change(function (){ var value = $(this).val(); $("#areaList").html("<option value='0'>请选择</option>"); $("input[name^='city_"+value+"']").each(function(){ var val = $(this).val(); $("#areaList").append("<option value='"+val.split("_")[1]+"'>"+val.split("_")[0]+"</option>"); }); }); });
========
三 、 Action中将provinceList 转成JSON字符串传给页面保存:
=========================
========
三 、 Action中将provinceList 转成JSON字符串传给页面保存:
=========================
jsp:<div> <select id="provinceList" class="sele"><option>请选择</option></select> <select id="cityList" class="sele"><option value="">请选择</option></select> <select id="areaList" name="deliveryaddress.area.id" class="sele"><option value="">请选择</option></select> </div> <%-- 直接在js里保存后台传来JSON的字符串 页面无需保存 <s:hidden id="provinceJSONString" value="%{provinceJSONString}"></s:hidden> --%>js:将后台传来的字符串保存到js对象中
$(function(){ var provinceJSONString = $("#provinceJSONString").val();//将java的String对象转成js字符对象 var provinceJSON = eval('('+provinceJSONString+')');//转换为json对象 /** * 首次加载页面,填充province列表 * 加载一级列表 */ loadOptions(provinceJSON,$("#provinceList")); function loadOptions(data,item){ $.each(data, function(index,value){ item.append($("<option />").val(index).text(value.name)); }); } /** * 加载二级列表 */ $("#provinceList").change(function (){ var value = $(this).val(); $("#cityList").html("<option value='0'>请选择</option>"); loadOptions(provinceJSON[value].cities,$("#cityList")); $("#areaList").html("<option value='0'>请选择</option>"); });action:将provinceList转成JSON格式的字符串
/** * provinceList转成JSON字符串 * @param _provinceList * @return * @throws Exception */ public static String toProvinceJSONString(List<Province> _provinceList) throws Exception { if (_provinceList == null) return null; Iterator<Province> itrProvince = _provinceList.iterator(); StringBuffer strBuffer = new StringBuffer("["); while(itrProvince.hasNext()) { Province _province = itrProvince.next(); strBuffer.append("{\"id\":\""+_province.getId()+"\",\"name\":\""+_province.getName()+"\",\"cities\":["); Set<City> Cities = _province.getCities(); Iterator<City> itrCity = Cities.iterator(); while(itrCity.hasNext()) { City _city = itrCity.next(); strBuffer.append("{\"id\":"+_city.getId()+",\"name\":\""+_city.getName()+"\",\"areas\":["); Set<Area> Areas = _city.getAreas(); Iterator<Area> itrArea = Areas.iterator(); while(itrArea.hasNext()) { Area _area = itrArea.next(); strBuffer.append("{\"id\":\""+_area.getId()+"\",\"name\":\""+_area.getName()+"\"}"); if (itrArea.hasNext()) { strBuffer.append(","); } } strBuffer.append("]}"); if (itrCity.hasNext()) { strBuffer.append(","); } } strBuffer.append("]}"); if (itrProvince.hasNext()) { strBuffer.append(","); } } strBuffer.append("]"); return strBuffer.toString(); }