版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/stuqbx/article/details/8524579
文笔不好,不废话,直接进入正题,不解释,相信大家都看得懂。
按百分比缩放图像:
public static Image ScaleByPercent(Image imgPhoto, int Percent) { float nPercent = ((float)Percent/100); int sourceWidth = imgPhoto.Width; int sourceHeight = imgPhoto.Height; int sourceX = 0; int sourceY = 0; int destX = 0; int destY = 0; int destWidth = (int)(sourceWidth * nPercent); int destHeight = (int)(sourceHeight * nPercent); Bitmap bmPhoto = new Bitmap(destWidth, destHeight, PixelFormat.Format24bppRgb); bmPhoto.SetResolution(imgPhoto.HorizontalResolution, imgPhoto.VerticalResolution); Graphics grPhoto = Graphics.FromImage(bmPhoto); grPhoto.InterpolationMode = InterpolationMode.HighQualityBicubic; grPhoto.DrawImage(imgPhoto, new Rectangle(destX,destY,destWidth,destHeight), new Rectangle(sourceX,sourceY,sourceWidth,sourceHeight), GraphicsUnit.Pixel); grPhoto.Dispose(); return bmPhoto; }
按指定大小缩放图像:
public static Image FixedSize(Image imgPhoto, int Width, int Height) { int sourceWidth = imgPhoto.Width; int sourceHeight = imgPhoto.Height; int sourceX = 0; int sourceY = 0; int destX = 0; int destY = 0; float nPercent = 0; float nPercentW = 0; float nPercentH = 0; nPercentW = ((float)Width/(float)sourceWidth); nPercentH = ((float)Height/(float)sourceHeight); if(nPercentH < nPercentW) { nPercent = nPercentH; destX = System.Convert.ToInt16((Width - (sourceWidth * nPercent))/2); } else { nPercent = nPercentW; destY = System.Convert.ToInt16((Height - (sourceHeight * nPercent))/2); } int destWidth = (int)(sourceWidth * nPercent); int destHeight = (int)(sourceHeight * nPercent); Bitmap bmPhoto = new Bitmap(Width, Height, PixelFormat.Format24bppRgb); bmPhoto.SetResolution(imgPhoto.HorizontalResolution, imgPhoto.VerticalResolution); Graphics grPhoto = Graphics.FromImage(bmPhoto); grPhoto.Clear(Color.Red); grPhoto.InterpolationMode = InterpolationMode.HighQualityBicubic; grPhoto.DrawImage(imgPhoto, new Rectangle(destX,destY,destWidth,destHeight), new Rectangle(sourceX,sourceY,sourceWidth,sourceHeight), GraphicsUnit.Pixel); grPhoto.Dispose(); return bmPhoto; }
按指定位置裁剪指定大小图像:
public static Image Crop(Image imgPhoto, int Width, int Height, AnchorPosition Anchor) { int sourceWidth = imgPhoto.Width; int sourceHeight = imgPhoto.Height; int sourceX = 0; int sourceY = 0; int destX = 0; int destY = 0; float nPercent = 0; float nPercentW = 0; float nPercentH = 0; nPercentW = ((float)Width/(float)sourceWidth); nPercentH = ((float)Height/(float)sourceHeight); if(nPercentH < nPercentW) { nPercent = nPercentW; switch(Anchor) { case AnchorPosition.Top: destY = 0; break; case AnchorPosition.Bottom: destY = (int) (Height - (sourceHeight * nPercent)); break; default: destY = (int) ((Height - (sourceHeight * nPercent))/2); break; } } else { nPercent = nPercentH; switch(Anchor) { case AnchorPosition.Left: destX = 0; break; case AnchorPosition.Right: destX = (int) (Width - (sourceWidth * nPercent)); break; default: destX = (int) ((Width - (sourceWidth * nPercent))/2); break; } } int destWidth = (int)(sourceWidth * nPercent); int destHeight = (int)(sourceHeight * nPercent); Bitmap bmPhoto = new Bitmap(Width, Height, PixelFormat.Format24bppRgb); bmPhoto.SetResolution(imgPhoto.HorizontalResolution, imgPhoto.VerticalResolution); Graphics grPhoto = Graphics.FromImage(bmPhoto); grPhoto.InterpolationMode = InterpolationMode.HighQualityBicubic; grPhoto.DrawImage(imgPhoto, new Rectangle(destX,destY,destWidth,destHeight), new Rectangle(sourceX,sourceY,sourceWidth,sourceHeight), GraphicsUnit.Pixel); grPhoto.Dispose(); return bmPhoto; }
转载请注明出处!!!
扫描二维码关注公众号,回复:
3036180 查看本文章
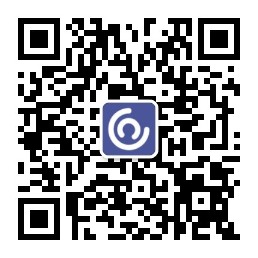