效果图
虽然说现在已经不流行jquery了,但是有时候操作dom,没有比他更简单的框架了,
一、先说一下功能和需求
1.我们有时候需要限制上传的个数,那么到底要设置最多上传多少个图片呢?这个教给产品经理,他想上传多少个,咱们就能上传多少个
2.有时候我们选择完图片,我发现我选错图片了,我又不想刷新界面,我想删除指定的被选择的图片,这要是不会实现就挺尴尬了。
3.其实还想做一下,图片的展示功能(就是点击一下图片,可以查看高清大图,你懂得),这里我没做给略过了。原理就是最原始的拼接字符串,一顿dom操作就完活。
4.提交图片的地址,想写在前端html里面,为以后改起来方便。
5.兼容移动端哦
二、实现方法和代码
扫描二维码关注公众号,回复:
3019978 查看本文章
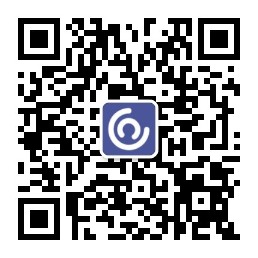
1.我用面向对象的方式编程,formdata,ajax提交到后台
2.使用工厂模式,把所有函数都放在一个对象里面
3.调用哪个函数,我就该对象.上谁(这块看不懂,一会代码里面会有介绍)
4.得,不多说了,直接开始写代码!!!
三、代码
1.项目目录结构
2.ad_img.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,minimum-scale=1.0,maximum-scale=1.0"/>
<title>上传广告图</title>
<link rel="stylesheet" href="css/index.css">
<script src="js/jquery-3.2.1.min.js"></script>
</head>
<body>
<div id="box" style="">
<!-- 上传广告图 -->
<form class="upBox">
<div class="inputBox">
<input type="file" title="请选择图片" id="file1" multiple accept="image/png,image/jpg,image/gif,image/JPEG"/><span class="goon">点击选择</span><strong>广告图</strong></div>
<div id="imgBox1"></div>
<a class="btn" id="btn1">上传</a>
</form>
</div>
<script src="js/upload.js" charset="utf-8"></script>
<script type="text/javascript">
var removeImg = move.removeImg;
// ad_img
move.imgUpload({
inputId:'file1', //input框id
imgBox:'imgBox1', //图片容器id
buttonId:'btn1', //提交按钮id
upUrl:'http://.......',//提交地址
data:'file1', //参数名
num:"2"//上传个数限制
});
</script>
</body>
</html>
3.index.css
*{
margin: 0;
padding: 0;
}
strong{
color: #f63300;
}
.upBox{
text-align: center;
width:70%;
padding: 20px;
border: 1px solid #666;
margin: auto;
margin-top: 30px;
position: relative;
border-radius: 10px;
}
.inputBox{
width: 120px;
height: 40px;
margin: auto;
border: 1px solid cornflowerblue;
color: cornflowerblue;
border-radius: 5px;
position: relative;
text-align: center;
line-height: 40px;
overflow: hidden;
font-size: 16px;
padding: 0 10px;
}
.inputBox input{
width: 70%;
height: 40px;
opacity: 0;
cursor: pointer;
position: absolute;
top: 0;
left: -14%;
}
#imgBox{
text-align: center;
}
.imgContainer{
display: inline-block;
width: 32%;
height: 150px;
margin-left: 1%;
border: 1px solid #666666;
position: relative;
margin-top: 30px;
box-sizing: border-box;
}
.imgContainer img{
width: 100%;
height: 150px;
cursor: pointer;
}
.imgContainer p{
position: absolute;
bottom: -1px;
left: 0;
width: 100%;
height: 30px;
background: red;
text-align: center;
line-height: 30px;
color: #fff;
font-size: 16px;
font-weight: bold;
cursor: pointer;
display: none;
}
.imgContainer:hover p{
display: block;
}
.btn{
display: inline-block;
text-align: center;
line-height: 30px;
outline: none;
width: 100px;
height: 30px;
background: cornflowerblue;
border: 1px solid cornflowerblue;
color: white;
cursor: pointer;
margin-top: 30px;
border-radius: 5px;
}
4.最关键的,也是最重要,最核心的大人物来了,
jquery.js//这个自己随便在网上下载就好使,我用的是-3.2.1版本
upload.js
// function move 开始
var move = {
imgSrc:[], //图片路径
imgFile:[],//文件流
imgName:[], //图片名字
//选择图片
// 函数闭包,让外部引入调用
imgUpload :function(obj){
var oInput = '#' + obj.inputId;
var imgBox = '#' + obj.imgBox;
var btn = '#' + obj.buttonId;
$(oInput).on("change", ()=> {
var fileImg = $(oInput)[0];
var fileList = fileImg.files;
for(var i = 0; i < fileList.length; i++) {
var imgSrcI = this.getObjectURL(fileList[i]);
this.imgName.push(fileList[i].name);
this.imgSrc.push(imgSrcI);
this.imgFile.push(fileList[i]);
}
this.addNewContent(obj.imgBox);
// console.log(obj);
})//change
$(btn).on('click',()=> {
if(!this.limitNum(obj.num)){
alert("图片数量最多"+obj.num+"个");
return false;
}
else if(this.imgFile.length==0){
alert('请选择图片!');
}
else if(this.imgFile.length>0&&this.limitNum(obj.num)){
//用formDate对象上传
alert('图片上传中请稍后');
var fd = new FormData($('.upBox')[0]);
for(var i=0;i<this.imgFile.length;i++){
fd.append(obj.data+"[]",this.imgFile[i]);
}
// console.log(fd); //FormData 对象
move.submitPicture(obj,obj.upUrl, fd);
}
});//click
},//return
//图片展示
addNewContent:function (obj) {
// console.log(obj);
console.log(this.imgSrc.length);
$('#'+obj).html("");
for(var a = 0; a < this.imgSrc.length; a++) {
var oldBox = $('#'+obj).html();
$('#'+obj).html(oldBox + '<div class="imgContainer"><img title=' + this.imgName[a] + ' alt=' + this.imgName[a] + ' src=' + this.imgSrc[a] + ' onclick="imgDisplay(this)"><p onclick="removeImg(this,' + a + ')" class="imgDelete">删除</p></div>');
}
alert('您有'+this.imgSrc.length+'张图片预先加载完毕');
},
//删除
removeImg:function(obj,index){
// console.log(move.imgSrc)
// console.log(obj);//obj为p标签
// 删除原来数组中的,并且返回被删除的项目
move.imgSrc.splice(index,1);
move.imgFile.splice(index,1);
move.imgName.splice(index,1);
var boxId = $(obj).parent('.imgContainer').parent().attr("id");
move.addNewContent(boxId);
},
//限制图片个数
limitNum:function (num){
if(!num){
return true;
}else if(this.imgFile.length>num){
return false;
}else{
return true;
}
},
//上传(将文件流数组传到后台)
submitPicture :function(obj,url,data){
// for (var p of data) {
// console.log(p);
// // p就是文件流
// }
console.log($('#'+obj.imgBox))
if(url&&data){
$.ajax({
type: "post",
url: url,
async: true,
data: data,
processData: false,
contentType: false,
success: (dat)=> {
// alert(dat.msg);
alert('你成功上传了'+this.imgFile.length+'张图片');
// console.log(this);
this.imgSrc=[]; //图片路径
this.imgFile=[];//文件流
this.imgName=[];
// 移除当前的图片
$('#'+obj.imgBox).find('.imgContainer').remove();
$('.goon').html('继续上传');
// console.log(dat.msg);
},
error:function(dat){
alert('图片上传失败!');
console.log(dat);
}
});
}else{
alert('请打开控制台查看传递参数!');
}
},
//图片预览路径
getObjectURL:function (file) {
var url = null;
if(window.createObjectURL != undefined) { // basic
url = window.createObjectURL(file);
} else if(window.URL != undefined) { // mozilla(firefox)
url = window.URL.createObjectURL(file);
} else if(window.webkitURL != undefined) { // webkit or chrome
url = window.webkitURL.createObjectURL(file);
}
return url;
}
}
四、如果你想尝试的话,直接复制粘贴代码就可以了,希望可以帮到你。