目录
字符串处理
string模块提供了常用的常量,比如
string.ascii_letters、string.ascii_lowercase、string.ascii_uppercase
a、常用方法
b、字符串常量
以上可参考
https://blog.csdn.net/github_36601823/article/details/77815013
struct模块
提供了一些函数,可用于将数字、bool变量以及字符串打包打包为字节对象(二进制形式),或逆操作
可参考
https://blog.csdn.net/lis_12/article/details/52777983
difflib模块
提供了用于对序列(比如字符串)进行比较的类与方法,并可以产生以”diff”格式与HTML格式表示的输出信息
可参加
https://blog.csdn.net/lockey23/article/details/77913855
命令行解析模块
Python 有两个内建的模块用于处理命令行参数
一个是 getopt只能简单处理 命令行参数
另一个是 optparse,它功能强大,而且易于使用,可以方便地生成标准的、符合Unix/Posix 规范的命令行说明。会自动帮你负责-h帮助选项
optparse
#!/usr/bin/env python
# encoding: utf-8
from optparse import OptionParser
parser = OptionParser()
parser.add_option("-f", "--file", dest="file_name", help="write report to FILE")
parser.add_option("-q", "--quiet",
action="store_false", dest="verbose", default=True,
help="don't print status messages to stdout")
#options,它是一个对象,保存有命令行参数值。只要知道命令行参数名,如 input,就可以访问其对应的值:options.input
#args ,它是没被解析的命令行参数的列表。
(options, args) = parser.parse_args()#python .\1、hello.py -f outfile -q eee
print(options.file_name)#outfile
print(options.verbose)#False
print(args)#['eee']
更多可以参考
https://www.jianshu.com/p/bec089061742
http://www.jb51.net/article/59296.htm
getop
比较少用,功能也比较弱一些,具体可以参考链接
https://www.cnblogs.com/kex1n/p/5975722.html
数学与数字
Python中有三个可用的数值型标准库
math,用于标准的数学函数;
cmath,用于复数数学函数;
random,提供很多随机数生成函数
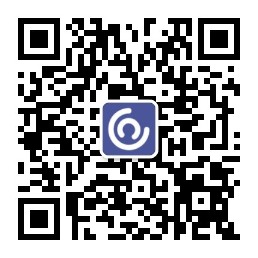
Python的数值抽象基类是在numbers模块中定义的,该基类在检测某个对象的具体类型时很有用,比如可以这样检测
#!/usr/bin/env python
# encoding: utf-8
import numbers
age = 24
print(isinstance(age, numbers.Integral))
print(isinstance(age, numbers.Rational))
print(isinstance(age, numbers.ABCMeta))
时间与日期模块
time模块可以处理时间戳,格式化时间
#!/usr/bin/env python
# encoding: utf-8
import time
#time.struct_time(tm_year=2018, tm_mon=6, tm_mday=7, tm_hour=16, tm_min=9, tm_sec=53, tm_wday=3, tm_yday=158, tm_isdst=0)
print(time.localtime())
print("当前时间戳:%s" % time.time())
print("当前时间戳:%s" % int(time.time()))
#生成自定义格式的时间
print(time.strftime("%Y-%m-%d", time.localtime()))#2018-06-07
datetime模块提供了用于处理日期与时间的函数
得到今天、前N天的日期是比较常见的功能
#!/usr/bin/env python
# encoding: utf-8
import datetime
#获取今天的信息
print(datetime.datetime.now())#2018-06-07 16:14:39.123364
print(datetime.date.today())#2018-06-07
#获取前两天的信息
print(datetime.date.today() - datetime.timedelta(days=2))#2018-06-05
#把昨天的信息格式化成2018***06***05
two_day_ago = datetime.date.today() - datetime.timedelta(days=2)
print(two_day_ago.strftime("%Y***%m***%d"))#2018***06***05
calendar模块主要用在日历相关
#!/usr/bin/env python
# encoding: utf-8
import calendar
# 获取某个月的日历,返回字符串类型
cal = calendar.month(2018, 12)
print(cal)
calendar.setfirstweekday(calendar.SUNDAY) # 设置日历的第一天
cal = calendar.month(2018, 12)
print(cal)
# 获取一年的日历
cal = calendar.calendar(2018)
print(cal)
cal = calendar.HTMLCalendar(calendar.MONDAY)
print(cal.formatmonth(2018, 12))
#判断是否闰年
print(calendar.isleap(2018))
#两个年份之间闰年的个数
print(calendar.leapdays(2010, 2018))
参考:
https://www.cnblogs.com/tkqasn/p/6001134.html
https://www.cnblogs.com/guigujun/p/6149770.html
http://www.jb51.net/article/77971.htm
https://www.cnblogs.com/BaiYiShaoNian/p/5084517.html
文件格式、编码
base64模块
对一行字符串进行编码与解码,如果文本里面遇到空格,会非常方便的进行压缩发送
#!/usr/bin/env python
# encoding: utf-8
import base64
str = u'zengraoli'
#py3对string与bytes做了区分,请参考https://www.cnblogs.com/abclife/p/7445222.html
str_bytes = str.encode('utf-8')
encode_str = base64.b64encode(str_bytes)
print(encode_str)#b'emVuZ3Jhb2xp'
decode_str = base64.b64decode(encode_str)
print(decode_str)#b'zengraoli'
print(decode_str.decode('utf-8'))#zengraoli
tarfile模块
该模块用于创建并拆分.tar与.tar.gz存放文件
使用方法可参考
https://www.jianshu.com/p/5609d67d8ab2
Shutil模块
Shutil模块提供了用于文件与目录处理的高层函数,能对文件以及整个文件夹进行拷贝(分别为shutil.copy()与shutil.copytree()), 用于移动文件的shutil.move()、移动文件夹的shutil.rmtree()
使用方法可参考
https://www.cnblogs.com/wang-yc/p/5625046.html
os模块
os模块封装了操作系统功能的访问接口
os.environ变量存放的是映射对象,存放环境变量名及其值;
os.getcwd()可以获取程序的工作目录
os.chdir()可以修改当前程序的工作目录
os.access()可用于确定某个文件是否存在,或者文件是否可读写
os.listdir()函数可以返回给定文件夹中的文件列表
os.stat()函数返回关于文件与目录的各种信息项,比如模式、访问时间与大小等
os.mkdir()可以创建目录
os.rmdir()移除空目录
os.walk()函数可以在整理文件夹上做迭代,依次取回每个文件与目录的名称
os.path模块则提供了路径操作函数,便于操纵文件系统的函数的混合
os.path.abspath()函数可以返回其参数的决定路径
os.path.split()函数返回一个二元组,其中第一项包含路径,第二项则是文件名,这两项可以直接用os.path.basename()与os.path.dirname()获取
使用实例课参考
https://www.cnblogs.com/znyyy/p/7716644.html