1、三种映射关系
drop table if exists husband;
drop table if exists wife;
create table wife(
id int auto_increment primary key,
name varchar(100)
);
create table husband(
id int auto_increment primary key,
name varchar(100),
wid int unique,
foreign key(wid) references wife(id)
);
create table dept(
id int auto_increment primary key,
name varchar(100)
);
create table emp(
id int auto_increment primary key,
name varchar(100),
did int,
foreign key(did) references dept(id) on delete cascade
);
create table student(
id int auto_increment primary key,
name varchar(100)
);
create table subject(
id int auto_increment primary key,
name varchar(100)
);
create table student_subject(
id int auto_increment primary key,
stuid int,
subid int,
foreign key(stuid) references student(id),
foreign key(subid) references subject(id)
);
2、连接查询
笛卡尔积
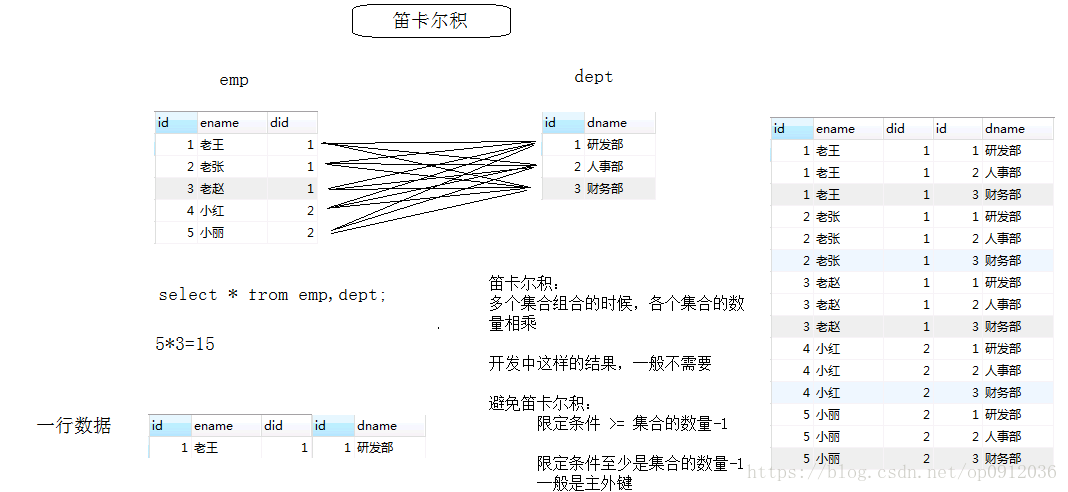
内连接
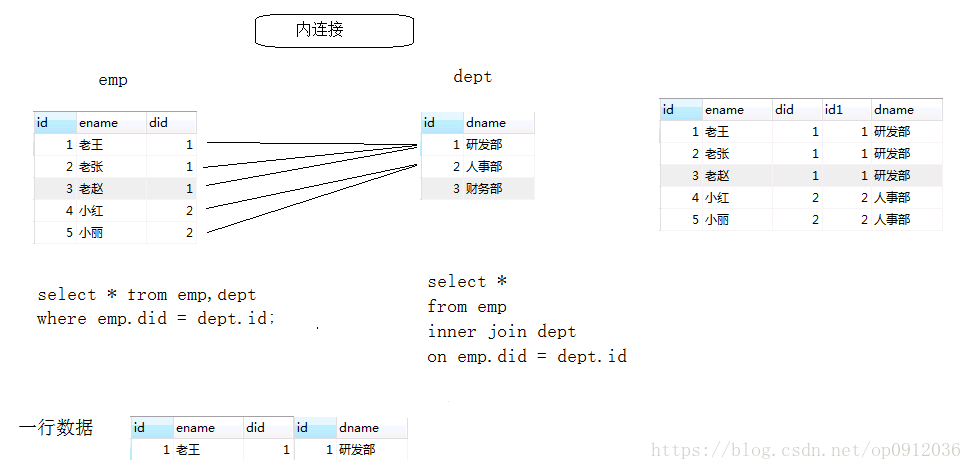
select * from DEPT,EMP
where DEPT.DEPTNO = EMP.DEPTNO;
select
EMP.EMPNO,EMP.ENAME,DEPT.DNAME
from
DEPT
inner join
EMP
on
DEPT.DEPTNO = EMP.DEPTNO;
select
EMP.EMPNO,EMP.ENAME,DEPT.DNAME
from
DEPT,EMP
where
DEPT.DEPTNO = EMP.DEPTNO;
select
EMP.EMPNO,EMP.ENAME,DEPT.DNAME
from
DEPT,EMP
where
DEPT.DEPTNO = EMP.DEPTNO
and
DEPT.DEPTNO = 10;
外连接
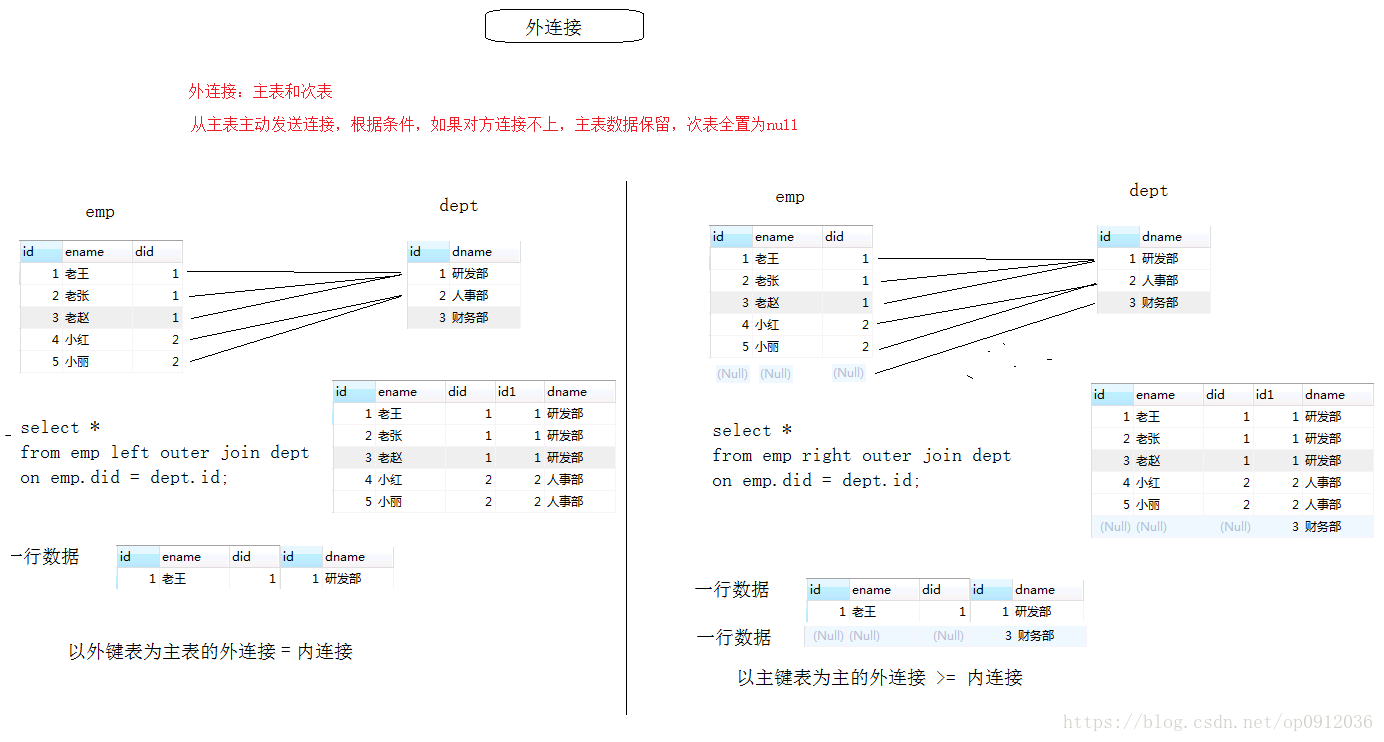
select
t2.EMPNO,t2.ENAME,t1.DEPTNO,t1.DNAME
from
DEPT t1
left join
EMP t2
on
t1.DEPTNO = t2.DEPTNO;
自连接
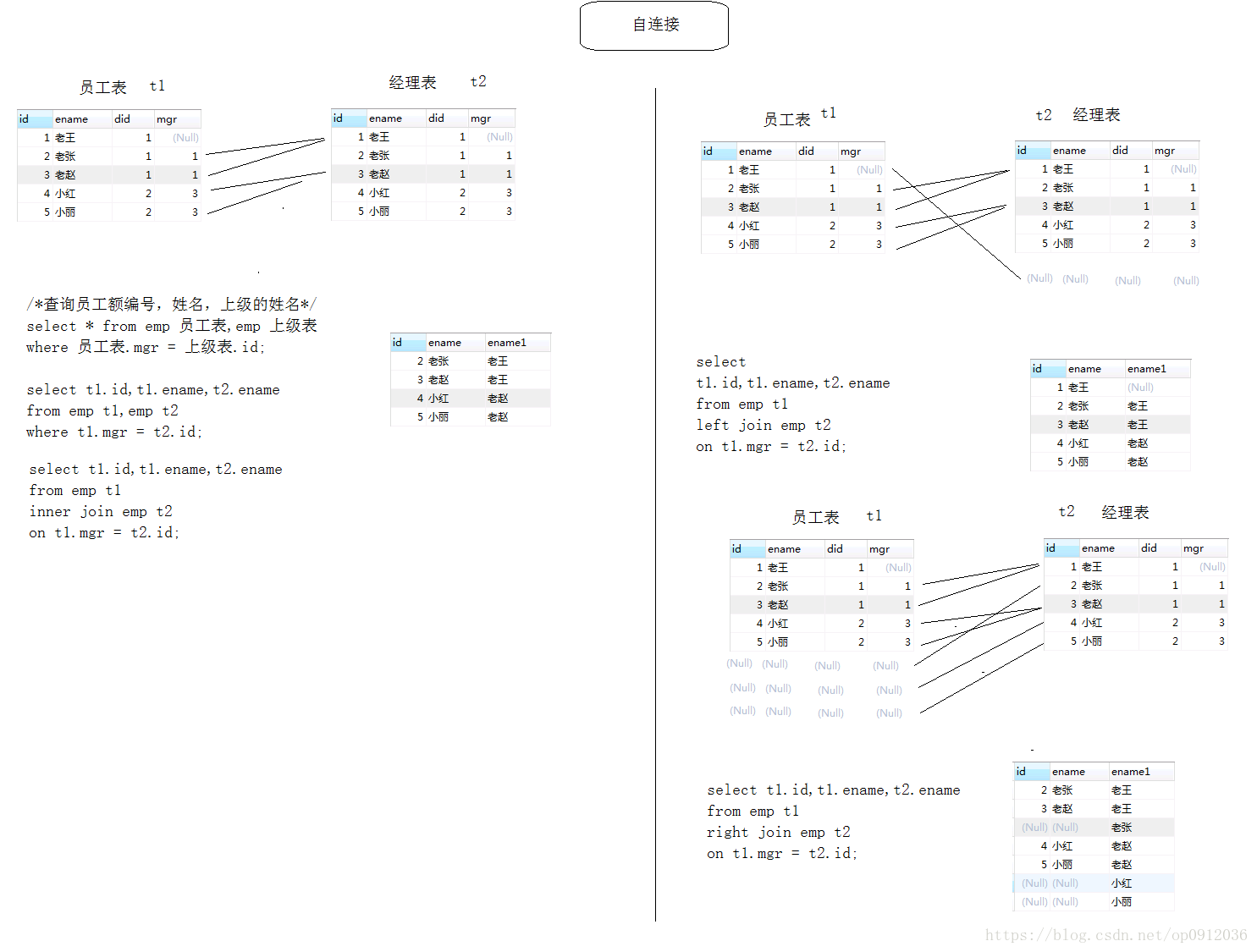
create table EMP2(
id int auto_increment primary key,
name varchar(100),
mgr int foreign key(mgr) references EMP2(id)
);
select t1.empno 员工的编号,t1.ename 员工的姓名,t2.ename 上级的姓名
from EMP t1,EMP t2
where t1.mgr = t2.empno
select t1.empno 员工的编号 ,t1.ename 员工的姓名,t2.ename 上级的姓名
from EMP t1 left join EMP t2
on t1.mgr = t2.empno;