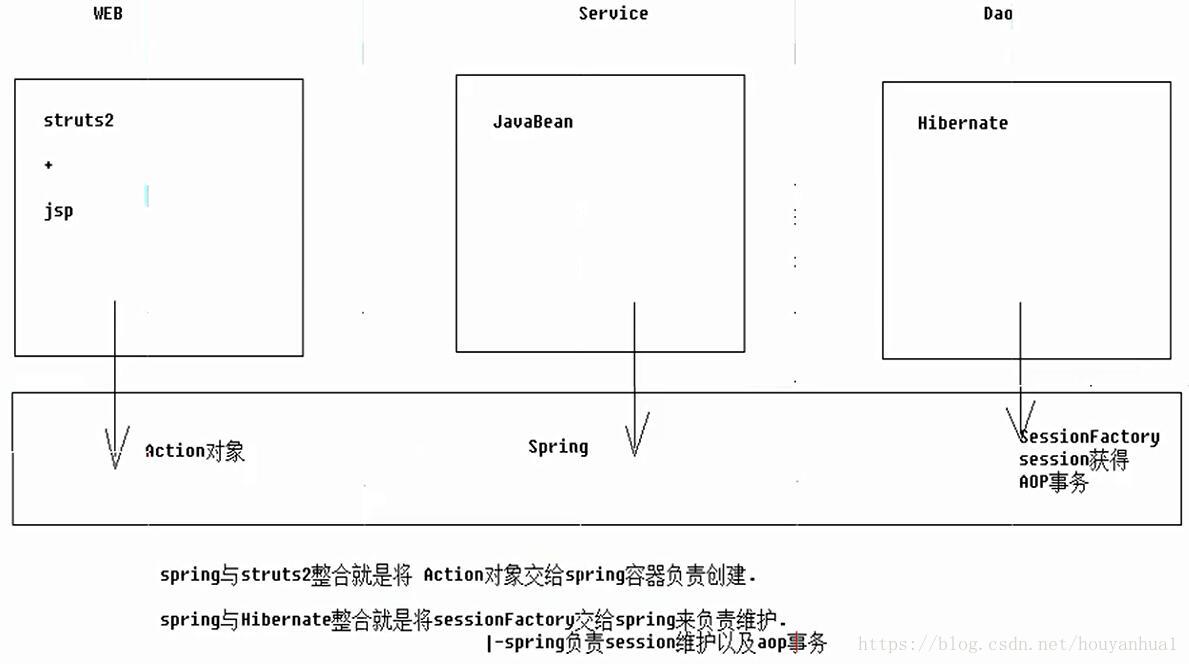
src/applicationContext.xml(Spring配置文件,配置SessionFactory对象,Spring加载Hibernate配置信息方案(推荐)):
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.2.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.2.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.2.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.2.xsd ">
<!-- 导入beans命名空间(约束) xmlns="http://www.springframework.org/schema/beans"
导入context命名空间(约束) (读取properties文件) xmlns:context="http://www.springframework.org/schema/context"
导入aop命名空间(约束) (通知织入目标对象) xmlns:aop="http://www.springframework.org/schema/aop"
导入tx命名空间(约束) (事务) xmlns:tx="http://www.springframework.org/schema/tx"
-->
<!-- 读取db.properties文件 -->
<context:property-placeholder location="classpath:db.properties" />
<!-- 配置c3p0连接池(连接池中的连接需要配置事务后才能正常提交) -->
<bean name="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource" >
<property name="jdbcUrl" value="${jdbc.jdbcUrl}" ></property>
<property name="driverClass" value="${jdbc.driverClass}" ></property>
<property name="user" value="${jdbc.user}" ></property>
<property name="password" value="${jdbc.password}" ></property>
</bean>
<!-- 核心事务管理器(不同的平台有不同的实现类) -->
<bean name="transactionManager" class="org.springframework.orm.hibernate5.HibernateTransactionManager" >
<property name="sessionFactory" ref="sessionFactory" ></property>
</bean>
<!-- 开启注解事务 -->
<tx:annotation-driven transaction-manager="transactionManager" />
<!-- 将SessionFactory配置到spring容器中(Spring整合Hibernate) -->
<!-- 加载Hibernate配置信息方案2(推荐):在spring配置中放置hibernate配置信息。不需要外部hibernate.cfg.xml配置文件了 -->
<!-- class要导入对应版本的Hibernate(例如:hibernate5) -->
<bean name="sessionFactory" class="org.springframework.orm.hibernate5.LocalSessionFactoryBean" >
<!-- 将连接池注入到sessionFactory,hibernate会通过连接池获得连接 -->
<property name="dataSource" ref="dataSource" ></property>
<!-- Spring中配置hibernate基本信息(可以从hibernate.cfg.xml配置文件中复制对应的键/值) -->
<property name="hibernateProperties">
<props>
<!-- 必选配置 -->
<!-- 如果SessionFactory对象不是通过连接池DataSource创建,那么需要加入以下的Hibernate配置信息
<prop key="hibernate.connection.driver_class" >com.mysql.jdbc.Driver</prop>
<prop key="hibernate.connection.url" >jdbc:mysql:///数据库名</prop>
<prop key="hibernate.connection.username" >root</prop>
<prop key="hibernate.connection.password" >1234</prop> -->
<prop key="hibernate.dialect" >org.hibernate.dialect.MySQLDialect</prop>
<!-- 可选配置 -->
<prop key="hibernate.show_sql" >true</prop>
<prop key="hibernate.format_sql" >true</prop>
<prop key="hibernate.hbm2ddl.auto" >update</prop>
</props>
</property>
<!-- 引入orm元数据,指定orm元数据所在的包路径,spring会自动读取包中的所有orm元数据配置(*.hbm.xml)。(包名中的"."换成"/") -->
<property name="mappingDirectoryLocations" value="classpath:cn/xxx/domain" ></property>
</bean>
<!-- Action配置(Spring整合Struts2) -->
<!-- 注意:Action对象作用范围一定是多例的(每个请求(线程)都对应一个Action对象;servlet是多线程的).这样才符合struts2架构 -->
<bean name="userAction" class="cn.xxx.web.action.UserAction" scope="prototype" >
<!-- 手动配置注入Action的依赖属性 -->
<property name="userService" ref="userService" ></property>
</bean>
<!-- service -->
<bean name="userService" class="cn.xxx.service.impl.UserServiceImpl" >
<property name="ud" ref="userDao" ></property>
</bean>
<!-- dao -->
<bean name="userDao" class="cn.xxx.dao.impl.UserDaoImpl" >
<!-- 注入sessionFactory -->
<property name="sessionFactory" ref="sessionFactory" ></property>
</bean>
</beans>
WEB-INF/web.xml(web项目配置,配置Spring监听器):
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5">
<display-name>MyWeb</display-name>
<!-- 通过监听器Listener,可以让spring容器随web项目的启动而创建,随项目的关闭而销毁.(可以保证Spring容器是单例的) -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<!-- 配置spring配置文件位置参数 -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:applicationContext.xml</param-value>
</context-param>
<!-- 扩大Hibernate中与数据库的连接会话session的作用范围 (避免Hibernate中懒加载,出现no-session的问题)
注意: 任何filter一定要在struts的filter之前调用
-->
<filter>
<filter-name>openSessionInView</filter-name>
<filter-class>org.springframework.orm.hibernate5.support.OpenSessionInViewFilter</filter-class>
</filter>
<!-- struts2核心过滤器 -->
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>openSessionInView</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
</web-app>
Test.java(测试类):
package cn.xxx.test;
import javax.annotation.Resource;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.Transaction;
import org.hibernate.cfg.Configuration;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import cn.xxx.domain.User;
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration("classpath:applicationContext.xml")
public class Test {
@Resource(name="sessionFactory")
//注入Spring容器中的SessionFactory对象
private SessionFactory sf ;
@Test
//测试spring管理sessionFactory
public void fun1(){
/* 单独使用Hibernate时,SessionFactory对象需要手动根据Hibernate配置信息创建
Configuration conf = new Configuration().configure(); //加载Hibernate配置信息
SessionFactory sf = conf.buildSessionFactory(); //手动创建SessionFactory对象
*/
Session session = sf.openSession(); //直接使用Spring容器注入的SessionFactory对象
Transaction tx = session.beginTransaction(); //事务可以交给Spring管理
//-------------------------------------------------
User u = new User();
u.setUser_code("rose");
u.setUser_name("肉丝");
u.setUser_password("1234");
session.save(u);
//-------------------------------------------------
tx.commit(); //事务可以交给Spring管理
session.close();
//sf.close(); //由Spring容器管理SessionFactory对象的生命周期,不需要手动关闭。
}
}