网上商城_数据库jar包的使用
0.导入数据库相关jar包
commons-dbutils-1.4.jar
c3p0-0.9.1.2.jar
1.配置C3P0-config.xml文件
-
<?xml version="1.0" encoding="UTF-8"?>
-
<c3p0-config>
-
<!-- 数据库连接池 -->
-
<default-config>
-
<property name="user">root</property>
-
<property name="password">admins</property>
-
<property name="driverClass">com.mysql.jdbc.Driver</property>
-
<property name="jdbcUrl">jdbc:mysql:///shop</property>
-
</default-config>
-
</c3p0-config>
2.然后写一个DataSourceUtils工具类

1 package com.shop.utils; 2 3 import java.sql.Connection; 4 import java.sql.ResultSet; 5 import java.sql.SQLException; 6 import java.sql.Statement; 7 8 import javax.sql.DataSource; 9 10 import com.mchange.v2.c3p0.ComboPooledDataSource; 11 12 /** 13 * 数据库连接工具 14 */ 15 public class DataSourceUtils { 16 17 private static DataSource dataSource = new ComboPooledDataSource(); 18 19 private static ThreadLocal<Connection> tl = new ThreadLocal<Connection>(); 20 21 // 直接可以获取一个连接池 22 public static DataSource getDataSource() { 23 return dataSource; 24 } 25 26 // 获取连接对象 27 public static Connection getConnection() throws SQLException { 28 29 Connection con = tl.get(); 30 if (con == null) { 31 con = dataSource.getConnection(); 32 tl.set(con); 33 } 34 return con; 35 } 36 37 // 开启事务 38 public static void startTransaction() throws SQLException { 39 Connection con = getConnection(); 40 if (con != null) { 41 con.setAutoCommit(false); 42 } 43 } 44 45 // 事务回滚 46 public static void rollback() throws SQLException { 47 Connection con = getConnection(); 48 if (con != null) { 49 con.rollback(); 50 } 51 } 52 53 // 提交并且 关闭资源及从ThreadLocall中释放 54 public static void commitAndRelease() throws SQLException { 55 Connection con = getConnection(); 56 if (con != null) { 57 con.commit(); // 事务提交 58 con.close();// 关闭资源 59 tl.remove();// 从线程绑定中移除 60 } 61 } 62 63 // 关闭资源方法 64 public static void closeConnection() throws SQLException { 65 Connection con = getConnection(); 66 if (con != null) { 67 con.close(); 68 } 69 } 70 71 public static void closeStatement(Statement st) throws SQLException { 72 if (st != null) { 73 st.close(); 74 } 75 } 76 77 public static void closeResultSet(ResultSet rs) throws SQLException { 78 if (rs != null) { 79 rs.close(); 80 } 81 } 82 83 }
3.最后在相关dao包就可以直接使用了
扫描二维码关注公众号,回复:
2928996 查看本文章
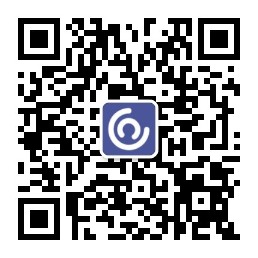
如:查询商品所有分类
-
//查询商品所有分类
-
public List<Category> findAllCategory() throws SQLException {
-
QueryRunner runner=new QueryRunner(DataSourceUtils.getDataSource());
-
String sql="select * from category";
-
return runner.query(sql,new BeanListHandler<Category>(Category.class));
-
}
如:添加商品分类
-
//添加商品分类
-
public void saveProductCategory(Category category) throws SQLException{
-
QueryRunner runner=new QueryRunner(DataSourceUtils.getDataSource());
-
String sql="insert into category values(?,?)";
-
runner.update(sql,category.getCid(),category.getCname());
-
}
这里查询是用query方法,增删改用update方法;
查询需要映射到一个实体类,而增删改往往需要预编译参数