版权声明:本文为博主原创文章,商业转载请联系作者获得授权,非商业转载请注明出处。 https://blog.csdn.net/liitdar/article/details/81668478
本文主要介绍使用C++语言、利用libcurl编写HTTP客户端的方法。
1 概述
libcurl 属于 curl 的一部分,libcurl is a free and easy-to-use client-side URL transfer library.
从 git 上下载 curl 源码,编译安装之后,就可以使用 libcurl 了。当然,也可以直接使用 yum 安装 libcurl。
curl 的源码中,附带了一些 libcurl 的使用示例,示例位置如下:
我们可以参考 libcurl 提供的示例代码,编写 HTTP 客户端(或者其他HTTP程序)。
2 示例程序
这里将展示 GET 和 POST 两种请求方式的 HTTP 客户端示例程序。
2.1 GET请求类型HTTP客户端
示例代码(libcurl_http_client_test1.cpp)如下:
#include <iostream>
#include <curl/curl.h>
#include <string>
using namespace std;
int BufferWriterFunc(char * data, size_t size, size_t nmemb, string * buffer)
{
int result = 0;
if (buffer != NULL)
{
buffer->append(data, size * nmemb);
result = size * nmemb;
}
return result;
}
int main()
{
CURL *curl;
CURLcode res;
string strUrl = "http://example.com?param1=value1¶m2=value2";
string strResponse;
curl_global_init(CURL_GLOBAL_DEFAULT);
// get a curl handle
curl = curl_easy_init();
if (curl)
{
// set the URL with GET request
curl_easy_setopt(curl, CURLOPT_URL, strUrl.data());
// write response msg into strResponse
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, BufferWriterFunc);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &strResponse);
// perform the request, res will get the return code
res = curl_easy_perform(curl);
// check for errors
if (res != CURLE_OK)
{
cout << "curl_easy_perform() failed: " << curl_easy_strerror(res) << endl;
}
else
{
cout << "curl_easy_perform() success." << endl;
cout << "strResponse is: " << strResponse << endl;
}
// always cleanup
curl_easy_cleanup(curl);
}
curl_global_cleanup();
return 0;
}
2.2 POST请求类型HTTP客户端
示例代码(libcurl_http_client_test2.cpp)如下:
#include <iostream>
#include <curl/curl.h>
#include <string>
using namespace std;
int BufferWriterFunc(char * data, size_t size, size_t nmemb, string * buffer)
{
int result = 0;
if (buffer != NULL)
{
buffer->append(data, size * nmemb);
result = size * nmemb;
}
return result;
}
int main()
{
CURL *curl;
CURLcode res;
string strResponse;
curl_global_init(CURL_GLOBAL_DEFAULT);
// get a curl handle
curl = curl_easy_init();
if (curl)
{
// set the URL that is about to receive our POST
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
// specify the POST data
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "param1=value1¶m2=value2");
// write response msg into strResponse
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, BufferWriterFunc);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &strResponse);
// Perform the request, res will get the return code
res = curl_easy_perform(curl);
// Check for errors
if (res != CURLE_OK)
{
cout << "curl_easy_perform() failed: " << curl_easy_strerror(res) << endl;
}
else
{
cout << "curl_easy_perform() success." << endl;
cout << "strResponse is: " << strResponse << endl;
}
// always cleanup
curl_easy_cleanup(curl);
}
curl_global_cleanup();
return 0;
}
说明:在上述的两个代码中,都是通过使用“curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, BufferWriterFunc); 和 curl_easy_setopt(curl, CURLOPT_WRITEDATA, &strResponse);”,将HTTP服务器返回的消息写入了strResponse中。
扫描二维码关注公众号,回复:
2916688 查看本文章
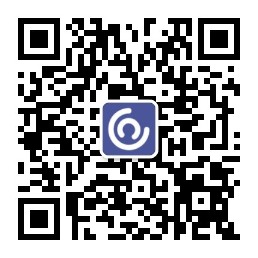