配置基本html,main.js
html代码如下:只要引入main.js即可
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Webpack App</title>
<link rel="stylesheet" type="text/css" href="/dist/main.css">
</head>
<body>
<div id="app">
</div>
<script type="text/javascript" src="/dist/main.js"></script>
</body>
</html>
js部分做到渲染:
import Vue from 'vue';
import App from './app.vue';
import './style.css';
new Vue({
el: '#app',
render: h => {
return h(App)
}
});
然后就是vue组件开发:
首先对知乎日报进行分析:日报是单页应用,由三部分组成,菜单,文章列表,文章内容以及评论
因为内容来自知乎,所以设置一个代理API来获取知乎内容,通过 NPM install request --save-dev来代理,
代理文件为proxy.js。
代码如下:
const http=require('http');
const request=require('request');
const hostname='127.0.0.1';//代理主机号,此处是本机
const port=8010;//接口端口
const imgPort=8011;//图片端口
//创建一个API代理服务
const apiServer=http.createServer((req,res)=>{
const url='http://news-at.zhihu.com/api/4' +req.url;//获取服务的url
const options={
url:url};
function callback(error,reponse,body){
if(!error&&response.statusCode===200){
//设置编码类型
res.setHeader('Conten-Type','text/plain;charset=UFT-8');
//设置所有域允许跨域
res.setHeader('Access-Control-Allow-Origin','*');
//返回代理后的内容
res.end(body);
}
}
request.get(options,callback);
}};
//监听8010
apiServer.listen(port, hostname, () => {
console.log(`接口代理运行在 http://${hostname}:${port}/`);
});
// 创建一个图片代理服务
const imgServer = http.createServer((req, res) => {
const url = req.url.split('/img/')[1];
const options = {
url: url,
encoding: null
};
function callback (error, response, body) {
if (!error && response.statusCode === 200) {
const contentType = response.headers['content-type'];
res.setHeader('Content-Type', contentType);
res.setHeader('Access-Control-Allow-Origin', '*');
res.end(body);
}
}
request.get(options, callback);
});
// 监听 8011 端口
imgServer.listen(imgPort, hostname, () => {
console.log(`图片代理运行在 http://${hostname}:${imgPort}/`);
});
这里使用axios 模块来做AJAX获取数据
Util.js
import axios from 'axios';
const Util = {
imgPath: 'http://127.0.0.1:8011/img/',
apiPath: 'http://127.0.0.1:8010/'
};
// 获取今天时间戳
Util.getTodayTime = function () {
const date = new Date();
date.setHours(0);
date.setMinutes(0);
date.setSeconds(0);
date.setMilliseconds(0);
return date.getTime();
};
// 获取上一天日期
Util.prevDay = function (timestamp = (new Date()).getTime()) {
const date = new Date(timestamp);
const year = date.getFullYear();
const month = date.getMonth() + 1 < 10
? '0' + (date.getMonth() + 1)
: date.getMonth() + 1;
const day = date.getDate() < 10
? '0' + date.getDate()
: date.getDate();
return year + '' + month + '' + day;
};
// Ajax 通用配置
Util.ajax = axios.create({
baseURL: Util.apiPath
});
// 添加响应拦截器
Util.ajax.interceptors.response.use(res => {
return res.data;
});
export default Util;
首先菜单组件:
扫描二维码关注公众号,回复:
2891781 查看本文章
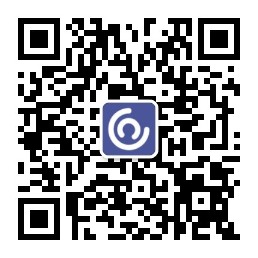
<div class="daily-menu">
<div class="daily-menu-item"
@click="handleToRecommend"
:class="{ on: type === 'recommend' }">每日推荐</div>
<div class="daily-menu-item"
:class="{ on: type === 'daily' }"
@click="showThemes = !showThemes">主题日报</div>
<ul v-show="showThemes">
<li v-for="item in themes" :key="item.id">
<a
:class="{ on: item.id === themeId && type === 'daily' }"
@click="handleToTheme(item.id)">{{ item.name }}</a>
</li>
</ul>
</div>
<script>
import $ from '../libs/Util.js'
handleToRecommend () {
this.type = 'recommend';
this.recommendList = [];
this.dailyTime = $.getTodayTime();
this.getRecommendList();
},
handleToTheme (id) {
this.type = 'daily';
this.themeId = id;
this.list = [];
$.ajax.get('theme/' + id).then(res => {
this.list = res.stories
.filter(item => item.type !== 1);
})
}
</script>