注解不支持所有的功能一般开发使用xml配置文件
查询直接返回Student 直接用@select
public interface StudentMapper {
@Insert("insert into t_student values(null,#{name},#{age})")
public int insertStudent(Student student);
@Update("update t_student set name=#{name},age=#{age} where id=#{id}")
public int updateStudent(Student student);
@Delete("delete from t_student where id=#{id}")
public int deleteStudent(int id);
@Select("select * from t_student where id=#{id}")
public Student getStudentById(Integer id);
}
返回集合
@Select("select * from t_student")
@Results(
{
@Result(id=true,column="id",property="id"),
@Result(column="name",property="name"),
@Result(column="age",property="age")
}
)
public List<Student> findStudents();
一对一关联查询
定义结果集
@Select("select * from t_student where id=#{id}")
@Results(
{
@Result(id=true,column="id",property="id"),
@Result(column="name",property="name"),
@Result(column="age",property="age"),
@Result(column="addressId",property="address",one=@One(select="com.java1234.mappers.AddressMapper.findById"))
}
)
public Student selectStudentWithAddress(int id);
public interface AddressMapper {
@Select("select * from t_address where id=#{id}")
public Address findById(Integer id);
}
一对多关联查询
public class Student {
private Integer id;
private String name;
private Integer age;
private Grade grade;
}
public class Grade {
private Integer id;
private String gradeName;
private List<Student> students;
}
@Select("select * from t_student where gradeId=#{gradeId}")
@Results(
{
@Result(id=true,column="id",property="id"),
@Result(column="name",property="name"),
@Result(column="age",property="age"),
@Result(column="addressId",property="address",one=@One(select="com.java1234.mappers.AddressMapper.findById"))
}
)
public Student selectStudentByGradeId(int gradeId);
@Select("select * from t_student where id=#{id}")
@Results(
{
@Result(id=true,column="id",property="id"),
@Result(column="name",property="name"),
@Result(column="age",property="age"),
@Result(column="addressId",property="address",one=@One(select="com.java1234.mappers.AddressMapper.findById")),
@Result(column="gradeId",property="grade",one=@One(select="com.java1234.mappers.GradeMapper.findById"))
}
)
public Student selectStudentWithAddressAndGrade(int id);
}
public interface GradeMapper {
@Select("select * from t_grade where id=#{id}")
@Results(
{
@Result(id=true,column="id",property="id"),
@Result(column="gradeName",property="gradeName"),
@Result(column="id",property="students",many=@Many(select="com.java1234.mappers.StudentMapper.selectStudentByGradeId"))
}
)
public Grade findById(Integer id);
}
动态sql
public class StudentDynaSqlProvider {
public String insertStudent(final Student student){
return new SQL(){
{
INSERT_INTO("t_student");
if(student.getName()!=null){
VALUES("name", "#{name}");
}
if(student.getAge()!=null){
VALUES("age", "#{age}");
}
}
}.toString();
}
}
public interface StudentMapper {
@InsertProvider(type=StudentDynaSqlProvider.class,method="insertStudent")
public int insertStudent(Student student);
}
public String updateStudent(final Student student){
return new SQL(){
{
UPDATE("t_student");
if(student.getName()!=null){
SET("name=#{name}");
}
if(student.getAge()!=null){
SET("age=#{age}");
}
WHERE("id=#{id}");
}
}.toString();
}
}
@UpdateProvider(type=StudentDynaSqlProvider.class,method="updateStudent")
public int updateStudent(Student student);
public String deleteStudent(){
return new SQL(){
{
DELETE_FROM("t_student");
WHERE("id=#{id}");
}
}.toString();
}
@DeleteProvider(type=StudentDynaSqlProvider.class,method="deleteStudent")
public int deleteStudent(int id);
public String getStudentById(){
return new SQL(){
{
SELECT("*");
FROM("t_student");
WHERE("id=#{id}");
}
}.toString();
}
@SelectProvider(type=StudentDynaSqlProvider.class,method="getStudentById")
public Student getStudentById(Integer id);
public String findStudents(final Map<String,Object> map){
return new SQL(){
{
SELECT("*");
FROM("t_student");
StringBuffer sb=new StringBuffer();
if(map.get("name")!=null){
sb.append(" and name like '"+map.get("name")+"'");
}
if(map.get("age")!=null){
sb.append(" and age="+map.get("age"));
}
if(!sb.toString().equals("")){
WHERE(sb.toString().replaceFirst("and", ""));
}
}
}.toString();
}
@SelectProvider(type=StudentDynaSqlProvider.class,method="findStudents")
public List<Student> findStudents(Map<String,Object> map);
扫描二维码关注公众号,回复:
2886057 查看本文章
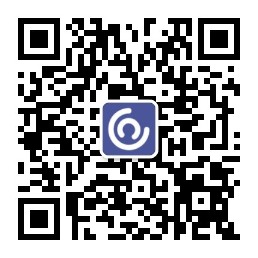