精通各种语言的 Hello World
int main(void)
{
std::cout<<"Hello world";
}
int main(void)
{
printf("\nhello world!"); \n 换行
return 0;
}
// 程序的入口
public static void main(String args[]){
// 向控制台输出信息
System.out.println("Hello World!");
}
}
echo "hello world!";
?>
import "fmt"
func main(){
fmt.Printf("Hello World!\n God Bless You!");
}
变量
- C++
int main(void)
{
std::cout<<"Hello world";
}
- C
int main(void)
{
printf("\nhello world!"); \n 换行
return 0;
}
- JAVA
// 程序的入口
public static void main(String args[]){
// 向控制台输出信息
System.out.println("Hello World!");
}
}
- PHP
echo "hello world!";
?>
- Ruby
- GO
import "fmt"
func main(){
fmt.Printf("Hello World!\n God Bless You!");
}
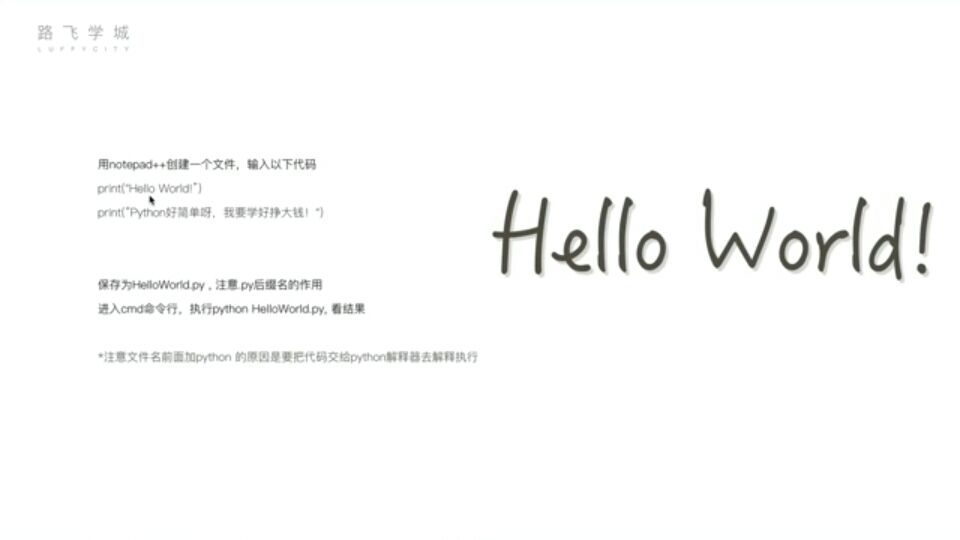
变量
计算机的主要作用之一:进行计算,用 Python进行数据运算非常容易,跟我们平常用计算机一样简单。
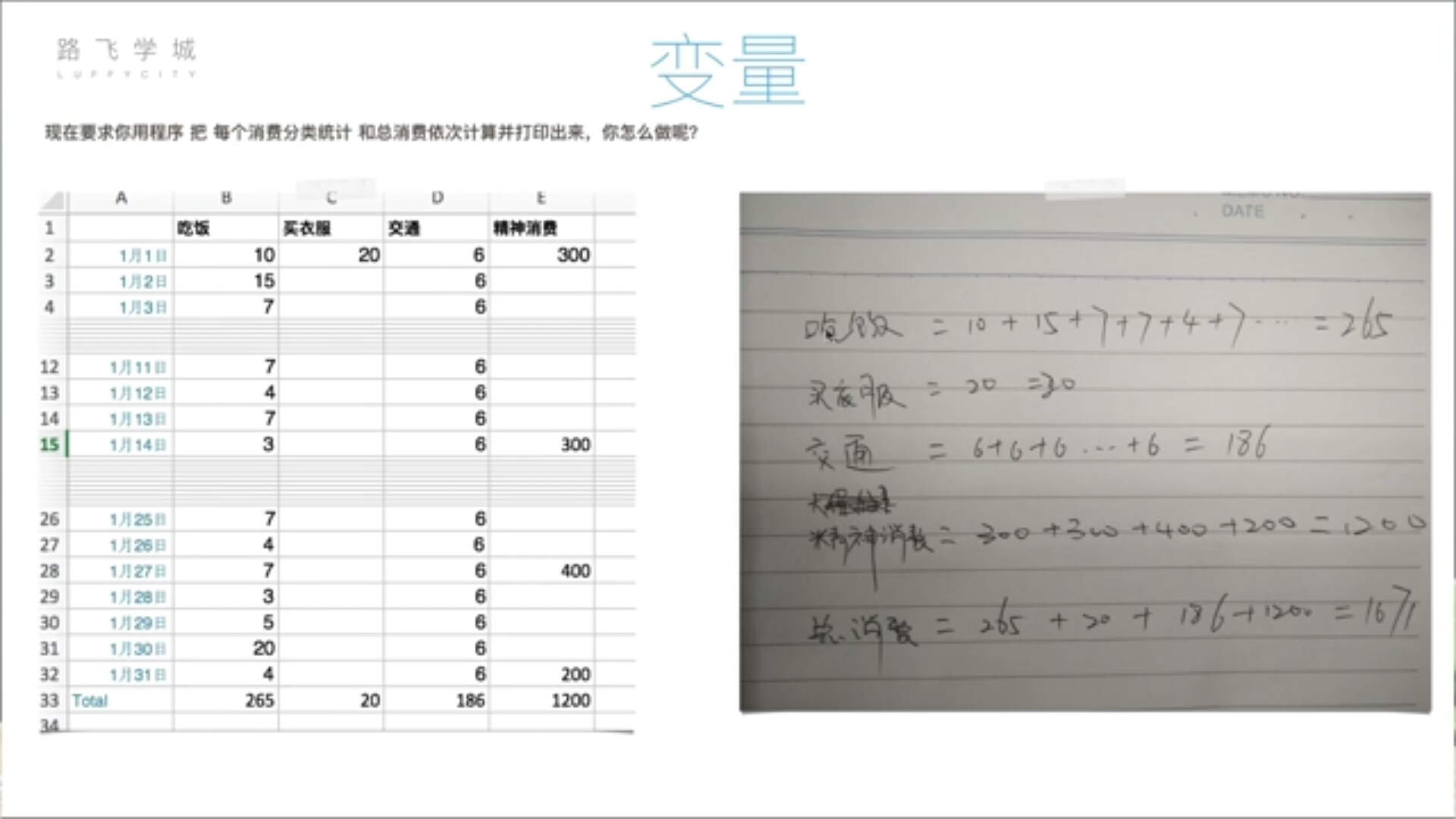
在算总消费的时候直接用的是之前已经算好的中间结果,这样是为了避免重新再算一遍所有的数据。
不正确的写法:
>>> print('eat',10+15+7+4+7+3)
eat 46
>>> print('cloth',20)
cloth 20
>>> print('traffic',6+6+6+6+6)
traffic 30
>>> print('精神',300+300+400+200)
精神 1200
>>>
>>>
>>> print('总消费', 46+20+30+1200)
总消费 1296
这么写之所以是不正确,是因为最后算总消费的时候 是人肉 把之前算出来的分类结果 填进去的, 但是我们把程序写在脚本里运行时, 计算机肯定不会预先知道吃饭、交通、买衣服3个分类的结果的,所以这个结果是我们自己动态算出来的,而不是计算机算出来的。
正确的写法是,直接把每个分类结果先起个名字存下来,然后计算总消费的时候,只需要把之前存下来的几个名字调用一下就可以了。
>>> eat = 10+15+7+4+7+3
>>> cloth = 20
>>> traffic = 6+6+6+6+6
>>> Spirit=300+300+200+400
>>>
>>> total = eat + cloth + traffic + Spirit
>>> print('总消费',total)
总消费 1296
变量名(标识符):name
变量值:Alex Li
②变量名的第一个字符不能是数字。
③下面的关键字不能声明为变量名:
'and'、 'as'、 'assert'、 'break'、'class' 、 'continue'、'def'、 'del'、'elif'、'else'、'except'、 'exec'、'finally'、 'for'、 'from'、 'global'、 'if'、 'import'、 'in'、 'is'、
>>> print('eat',10+15+7+4+7+3)
eat 46
>>> print('cloth',20)
cloth 20
>>> print('traffic',6+6+6+6+6)
traffic 30
>>> print('精神',300+300+400+200)
精神 1200
>>>
>>>
>>> print('总消费', 46+20+30+1200)
总消费 1296
这么写之所以是不正确,是因为最后算总消费的时候 是人肉 把之前算出来的分类结果 填进去的, 但是我们把程序写在脚本里运行时, 计算机肯定不会预先知道吃饭、交通、买衣服3个分类的结果的,所以这个结果是我们自己动态算出来的,而不是计算机算出来的。
正确的写法是,直接把每个分类结果先起个名字存下来,然后计算总消费的时候,只需要把之前存下来的几个名字调用一下就可以了。
>>> eat = 10+15+7+4+7+3
>>> cloth = 20
>>> traffic = 6+6+6+6+6
>>> Spirit=300+300+200+400
>>>
>>> total = eat + cloth + traffic + Spirit
>>> print('总消费',total)
总消费 1296
- 变量:eat、cloth、traffic、Spirit、total 这几个的作用就是把程序运算的中间结果临时存到内存里,可以让后面的代码继续调用,这几个的学名就叫做“变量”。
- 变量的作用 :
- 声明变量:
变量名(标识符):name
变量值:Alex Li
- 定义变量的规则:
②变量名的第一个字符不能是数字。
③下面的关键字不能声明为变量名:
'and'、 'as'、 'assert'、 'break'、'class' 、 'continue'、'def'、 'del'、'elif'、'else'、'except'、 'exec'、'finally'、 'for'、 'from'、 'global'、 'if'、 'import'、 'in'、 'is'、
'lambda'、'not'、'or'、 'pass'、 'print'、'raise'、'return'、'try'、 'while'、 'with'、'yield'
- 变量命名习惯:
AgeOfOldboy = 56
NumberOfStudents = 80
②下划线
age_of_oldboy = 56
number_of_students = 80
官方推荐使用第二种命名习惯,看起来更加的清晰。
- 定义变量的LOW 方式:
②变量名过长。
③变量名词不达意。
- 举例:
oldboy_gf_name = 'Lisa'
- 修改变量的值:
>>>oldboy_gf_age = 54 #老男孩女朋友的年龄为54
>>>age_of_oldboy + oldboy_gf_age # 老男孩的年龄与老男孩女朋友的年龄为110
110 结果
>>>oldboy_gf_age = 55 #修改老男孩女朋友的年龄为55
>>>age_of_oldboy + oldboy_gf_age #修改后老男孩的年龄与老男孩女朋友的年龄为111
111 修改后的结果
常量
- 常量:
常量即指不变的量,例如,pai=3.141592653..., 或在程序运行过程中不会改变的量。在Python中没有一个专门的语法代表常量,约定俗成用变量名全部大写代表常量。例如:
AGE_OF_OLDBOY = 56
在c语言中有专门的常量定义语法,const int count = 60; 一旦定义为常量,更改即会报错。
用户交互
name = input("What is your name:")
print("Hello " + name )
执行脚本后,发现程序会等输入姓名后才能往下继续走。
AGE_OF_OLDBOY = 56
在c语言中有专门的常量定义语法,const int count = 60; 一旦定义为常量,更改即会报错。
用户交互
name = input("What is your name:")
print("Hello " + name )
执行脚本后,发现程序会等输入姓名后才能往下继续走。
让用户输入多个信息:
name = input("What is your name:")age = input("How old are you:")
hometown = input("Where is your hometown:")
print("Hello ",name , "your are ", age , "years old, you came from",hometown)
执行输出:
What is your name:Wu qianqian
How old are you:21
Where is your hometown:ShiJiaZhuang
Hello Wu qianqian your are 21 years old, you came from ShiJiaZhuang
注释
代码注释分为单行和多行注释。 单行注释用#,多行注释可以用三对双引号""" """。
例:
def subclass_exception(name, parents, module, attached_to=None):
"""
Create exception subclass. Used by ModelBase below.
If 'attached_to' is supplied, the exception will be created in a way that
allows it to be pickled, assuming the returned exception class will be added
as an attribute to the 'attached_to' class.
"""
class_dict = {'__module__': module}
if attached_to is not None:
def __reduce__(self):
# Exceptions are special - they've got state that isn't
# in self.__dict__. We assume it is all in self.args.
return (unpickle_inner_exception, (attached_to, name), self.args)
def __setstate__(self, args):
self.args = args
class_dict['__reduce__'] = __reduce__
class_dict['__setstate__'] = __setstate__
return type(name, parents, class_dict)
- 代码注释的原则:
②注释可以用中文或英文,但绝对不要用拼音。