using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data;
using Aspose.Words;
using System.Configuration;
using System.Data.SqlClient;
using System.Reflection;
using Aspose.Words.Drawing;
using System.Text.RegularExpressions;
using System.IO;
using Aspose.Words.Saving;
using Aspose.Words.Tables;
using System.Text;
using System.Drawing.Printing;
using System.Collections;
namespace xxxxx{
public class Print{
#region 打印多个模板拼接成word
public Document printDocumentAllWordMore(List<PrintData> datalist)
{
int p = 0;
Document maindoc = new Document();
foreach (var page in datalist)
{
Document newdoc = new Document(page.wordpath);
DocumentBuilder builder = new DocumentBuilder(newdoc);
List<DataTable> dtinfos = page.dtinfos;
if (dtinfos != null)
{
for (int i = 0; i < dtinfos.Count; i++)
{
DataTable dtinfo = dtinfos[i];
if (dtinfo != null)
{
for (int n = 0; n < dtinfo.Columns.Count; n++)
{
string fieldname = dtinfo.Columns[n].ColumnName.ToLower();
try
{
string fieldvalue = dtinfo.Rows[0][fieldname].ToString();
newdoc.Range.Replace(WordTabChar + fieldname + WordTabChar, fieldvalue, false, false);
}
catch { }
}
}
}
}
Dictionary<string, string> dict = page.dict;
if (dict != null)
{
foreach (var key in dict.Keys)
{
try
{
newdoc.Range.Replace(WordTabChar + key + WordTabChar, dict[key], false, false);
}
catch { }
}
}
List<PositionProChildren> listpos = new List<PositionProChildren>();
List<DataTable> dtlist = page.dtlist;
int section = newdoc.GetChildNodes(NodeType.Section, true).Count;
int dataindex = 0;
for (int kk = 0; kk < section; kk++)
{
Aspose.Words.Section dtsection = (Aspose.Words.Section)newdoc.GetChild(NodeType.Section, kk, true);
int num = dtsection.Body.Tables.Count;
for (int mm = 0; mm < num; mm++)
{
Aspose.Words.Tables.Table dtdoc1 = dtsection.Body.Tables[mm];
for (int r = 0; r < dtdoc1.Rows.Count; r++)
{
for (int c = 0; c < dtdoc1.Rows[r].Cells.Count; c++)
{
if (dtdoc1.Rows[r].Cells[c].Range.Text.ToLower().Contains("#start#"))
{
PositionProChildren pos = new PositionProChildren();
pos.tableindex = mm;
pos.row_start = r;
pos.cell_start = c;
pos.row_end = r;
pos.cell_end = c;
listpos.Add(pos);
dtdoc1.Rows[r].Cells[c].Range.Replace("#START#", "", false, false);
}
if (dtdoc1.Rows[r].Cells[c].Range.Text.ToLower().Contains("#end#"))
{
PositionProChildren pos = listpos.Last<PositionProChildren>();
pos.row_end = r;
pos.cell_end = c;
dtdoc1.Rows[r].Cells[c].Range.Replace("#END#", "", false, false);
}
}
}
for (int i = 0; i < listpos.Count; i++)
{
PositionProChildren pos = new PositionProChildren();
if (listpos.Count > i)
{
pos = listpos[i];
}
DataTable dt = dtlist[i + dataindex];
Aspose.Words.Tables.Table dtdoc = dtsection.Body.Tables[mm];
List<string> celltabs = new List<string>();
for (int t = pos.cell_start; t < dtdoc.Rows[pos.row_start].Cells.Count; t++)
{
string colname = dtdoc.Rows[pos.row_start].Cells[t].Range.Text.Replace(WordTabChar, "").Replace("\a", "");
celltabs.Add(colname);
dtdoc.Rows[pos.row_start].Range.Replace(WordTabChar + colname + WordTabChar, "", false, false);
}
if (dt.Rows.Count > pos.rownum)
{
int addrow = dt.Rows.Count - pos.rownum;
for (int a = 0; a < addrow; a++)
{
Aspose.Words.Node newrow = dtdoc.Rows[pos.row_start + 1].Clone(true);//确认模板有第二行
//Aspose.Words.Node newrow = dtdoc.Rows[pos.row_start].Clone(true);
dtdoc.Rows.Insert(pos.row_start + 1, newrow);
if (i < listpos.Count - 1)
{
for (int l = i + 1; l < listpos.Count; l++)
{
PositionProChildren poscur = listpos[l - 1];
PositionProChildren posnext = listpos[l];
if (posnext.tableindex.Equals(poscur.tableindex))
{
posnext.row_start += 1;
posnext.row_end += 1;
}
else
break;
}
}
}
}
for (int m = 0; m < dt.Rows.Count; m++)
{
for (int n = 0; n < celltabs.Count; n++)
{
try
{
builder.MoveToSection(kk);
//builder.MoveTo(newdoc.Sections[kk].Body.Tables[mm].Rows[pos.row_start + m].Cells[pos.cell_start + n].Paragraphs[0].Runs[0]);
builder.MoveToCell(pos.tableindex, pos.row_start + m, pos.cell_start + n, 0);
builder.Write(dt.Rows[m][celltabs[n].ToString()].ToString());
}
catch { }
}
}
}
//dataindex = 0;
dataindex = dataindex + listpos.Count;
listpos.Clear();
}
}
if (!p.Equals(0))
{
newdoc.FirstSection.PageSetup.SectionStart = SectionStart.NewPage;
maindoc.AppendDocument(newdoc, ImportFormatMode.KeepSourceFormatting);
}
else
{
maindoc = newdoc;
}
p++;
}
return maindoc;
}
public void PrintMoreMb(List<List<PrintData>> datalist)
{
if (datalist.Count > 0)
{
Document maindoc = printDocumentAllWordMore(datalist[0]);
Document newdoc = new Document();
for (int i = 1; i < datalist.Count; i++)
{
newdoc = printDocumentAllWordMore(datalist[i]);
newdoc.FirstSection.PageSetup.SectionStart = SectionStart.NewPage;
maindoc.AppendDocument(newdoc, ImportFormatMode.KeepSourceFormatting);
}
maindoc.Protect(ProtectionType.AllowOnlyFormFields,"mima");
WaterMark(maindoc, HttpContext.Current.Session["LRXSMC_SH"].ToString());
//WaterMarkMore(maindoc, HttpContext.Current.Session["LRXSMC_SH"].ToString());
this.SaveDocument(maindoc);
}
}
//public Object apply(Shape watermark)
//{
// watermark.setRelativeHorizontalPosition(RelativeHorizontalPosition.MARGIN);
// watermark.setRelativeVerticalPosition(RelativeVerticalPosition.MARGIN);
// watermark.setWrapType(WrapType.NONE);
// // 我们需要自定义距离顶部的高度
// // watermark.setVerticalAlignment(VerticalAlignment.TOP);
// watermark.setHorizontalAlignment(HorizontalAlignment.CENTER);
// // 设置距离顶部的高度
// watermark.setTop(160);
// return null;
//}
/// <summary>
/// 插入多个水印
/// </summary>
/// <param name="mdoc">Document</param>
/// <param name="wmText">水印文字名</param>
/// <param name="left">左边距多少</param>
/// <param name="top">上边距多少</param>
/// <returns></returns>
public static Shape ShapeMore(Document mdoc, string wmText, double left, double top)
{
Shape waterShape = new Shape(mdoc, ShapeType.TextPlainText);
//设置该文本的水印
waterShape.TextPath.Text = wmText;
waterShape.TextPath.FontFamily = "宋体";
waterShape.Width = 50;
waterShape.Height = 50;
//文本将从左下角到右上角。
waterShape.Rotation = 0;
//绘制水印颜色
waterShape.Fill.Color = System.Drawing.Color.Gray;//浅灰色水印
waterShape.StrokeColor = System.Drawing.Color.Gray;
//将水印放置在页面中心
waterShape.Left = left;
waterShape.Top = top;
waterShape.WrapType = WrapType.None;
return waterShape;
}
/// <summary>
/// 插入多个水印
/// </summary>
/// <param name="mdoc">Document</param>
/// <param name="wmText">水印文字名</param>
public static void WaterMarkMore(Document mdoc, string wmText)
{
Paragraph watermarkPara = new Paragraph(mdoc);
for (int j = 20; j < 500; j = j + 100)
{
for (int i = 20; i < 700; i = i + 50)
{
Shape waterShape = ShapeMore(mdoc, wmText, j, i);
watermarkPara.AppendChild(waterShape);
}
}
// 在每个部分中,最多可以有三个不同的标题,因为我们想要出现在所有页面上的水印,插入到所有标题中。
foreach (Section sect in mdoc.Sections)
{
// 每个区段可能有多达三个不同的标题,因为我们希望所有页面上都有水印,将所有的头插入。
InsertWatermarkIntoHeader(watermarkPara, sect, HeaderFooterType.HeaderPrimary);
InsertWatermarkIntoHeader(watermarkPara, sect, HeaderFooterType.HeaderFirst);
InsertWatermarkIntoHeader(watermarkPara, sect, HeaderFooterType.HeaderEven);
}
}
/// <summary>
/// 插入水印
/// </summary>
/// <param name="mdoc">Document</param>
/// <param name="wmText">水印名称</param>
public static void WaterMark(Document mdoc, string wmText)
{
Shape waterShape = new Shape(mdoc, ShapeType.TextPlainText);
//设置该文本的水印
waterShape.TextPath.Text = wmText;
waterShape.TextPath.FontFamily = "宋体";
waterShape.Width = 200;
waterShape.Height = 100;
//文本将从左下角到右上角。
waterShape.Rotation = -40;
//绘制水印颜色
waterShape.Fill.Color = System.Drawing.Color.Gray;//浅灰色水印
waterShape.StrokeColor = System.Drawing.Color.Gray;
//waterShape.Fill.Color = System.Drawing.Color.FromName("#DFF1DF");//浅灰色水印
//waterShape.StrokeColor = System.Drawing.Color.Gray;
//将水印放置在页面中心
//waterShape.Left = 200;
//waterShape.Top = 600;
waterShape.RelativeHorizontalPosition = RelativeHorizontalPosition.Page;
waterShape.RelativeVerticalPosition = RelativeVerticalPosition.Page;
waterShape.WrapType = WrapType.None;
waterShape.VerticalAlignment = VerticalAlignment.Center;
waterShape.HorizontalAlignment = HorizontalAlignment.Center;
// 创建一个新段落并在该段中添加水印。
Paragraph watermarkPara = new Paragraph(mdoc);
watermarkPara.AppendChild(waterShape);
// 在每个部分中,最多可以有三个不同的标题,因为我们想要出现在所有页面上的水印,插入到所有标题中。
foreach (Section sect in mdoc.Sections)
{
// 每个区段可能有多达三个不同的标题,因为我们希望所有页面上都有水印,将所有的头插入。
InsertWatermarkIntoHeader(watermarkPara, sect, HeaderFooterType.HeaderPrimary);
InsertWatermarkIntoHeader(watermarkPara, sect, HeaderFooterType.HeaderFirst);
InsertWatermarkIntoHeader(watermarkPara, sect, HeaderFooterType.HeaderEven);
}
}
private static void InsertWatermarkIntoHeader(Paragraph watermarkPara, Section sect, HeaderFooterType headerType)
{
HeaderFooter header = sect.HeadersFooters[headerType];
if (header == null)
{
// 当前节中没有指定类型的头,创建它
header = new HeaderFooter(sect.Document, headerType);
sect.HeadersFooters.Add(header);
}
// 在头部插入一个水印的克隆
header.AppendChild(watermarkPara.Clone(true));
}
#endregion
private void SaveDocument(Document doc)
{
//SaveOptions saveop = SaveOptions.CreateSaveOptions(filename + ".doc");
//doc.Save(HttpContext.Current.Response, filename + ".doc", ContentDisposition.Attachment, saveop);
if (Dotype.Equals("word"))
{
SaveOptions saveop = SaveOptions.CreateSaveOptions(filename + ".doc");
doc.Save(HttpContext.Current.Response, filename + ".doc", ContentDisposition.Attachment, saveop);
}
else if (Dotype.Equals("pdf"))
{
filename = filename + ".pdf";
string dirpath = HttpContext.Current.Server.MapPath("~/FlexPaper/docs");
if (!Directory.Exists(dirpath))
{
Directory.CreateDirectory(dirpath);
}
string strpath = dirpath + "/" + filename;
if (File.Exists(strpath))
{
File.Delete(strpath);
}
doc.Save(strpath, SaveFormat.Pdf);
FileInfo fileInfo = new FileInfo(strpath);
HttpContext.Current.Response.Clear();
HttpContext.Current.Response.ClearContent();
HttpContext.Current.Response.ClearHeaders();
filename = System.Web.HttpUtility.UrlEncode(filename, System.Text.Encoding.UTF8);
HttpContext.Current.Response.AddHeader("Content-Disposition", "attachment;filename=" + filename);
HttpContext.Current.Response.AddHeader("Content-Length", fileInfo.Length.ToString());
HttpContext.Current.Response.AddHeader("Content-Transfer-Encoding", "binary");
HttpContext.Current.Response.ContentType = "application/octet-stream";
//Response.ContentEncoding = System.Text.Encoding.UTF8;
HttpContext.Current.Response.WriteFile(fileInfo.FullName);
HttpContext.Current.Response.Flush();
HttpContext.Current.Response.End();
}
}
}
public class ReplaceText : IReplacingCallback
{
/// <summary>
/// 替换文本格式
/// </summary>
public string Text { get; set; }
public ReplaceText(string Text)
{
this.Text = Text;
}
public ReplaceAction Replacing(ReplacingArgs e)
{
//获取当前节点
var node = e.MatchNode;
Document doc = node.Document as Document;
DocumentBuilder builder = new DocumentBuilder(doc);
builder.MoveTo(node);
builder.Write(Text);
return ReplaceAction.Replace;
}
}
public class ReplaceHtml : IReplacingCallback
{
/// <summary>
/// 替换为html格式
/// </summary>
public string Text { get; set; }
public ReplaceHtml(string Text)
{
this.Text = Text;
}
public ReplaceAction Replacing(ReplacingArgs e)
{
//获取当前节点
var node = e.MatchNode;
Document doc = node.Document as Document;
DocumentBuilder builder = new DocumentBuilder(doc);
builder.MoveTo(node);
builder.InsertHtml(Text);
return ReplaceAction.Replace;
}
}
public class ReplaceImage : IReplacingCallback
{
/// <summary>
/// 替换图片
/// </summary>
public string ImageUrl { get; set; }
//
public ReplaceImage(string url)
{
this.ImageUrl = url;
}
public ReplaceAction Replacing(ReplacingArgs e)
{
//获取当前节点
if (!string.IsNullOrEmpty(ImageUrl))
{
var node = e.MatchNode;
Document doc = node.Document as Document;
DocumentBuilder builder = new DocumentBuilder(doc);
builder.MoveTo(node);
//builder.Write(Text);
Shape shape = new Shape(doc, ShapeType.Image);
shape.ImageData.SetImage(ImageUrl);
shape.Top = 0;
shape.Width = 80;
shape.Height = 104;
shape.HorizontalAlignment = HorizontalAlignment.Center;
CompositeNode node1 = shape.ParentNode;
builder.InsertNode(shape);
}
return ReplaceAction.Replace;
}
}
public class PositionPro
{
public string key { get; set; }
public int row_start { get; set; }
public int row_end { get; set; }
public int cell_start { get; set; }
public int cell_end { get; set; }
public int rownum
{
get
{
return row_end - row_start + 1;
}
}
}
public class PrintData
{
public List<DataTable> dtinfos;
public Dictionary<string, string> dict;
public List<DataTable> dtlist;
public string wordpath;
}
public class PositionProChildren
{
public int tableindex { get; set; }
public string key { get; set; }
public int row_start { get; set; }
public int row_end { get; set; }
public int cell_start { get; set; }
public int cell_end { get; set; }
public int rownum
{
get
{
return row_end - row_start + 1;
}
}
}
}
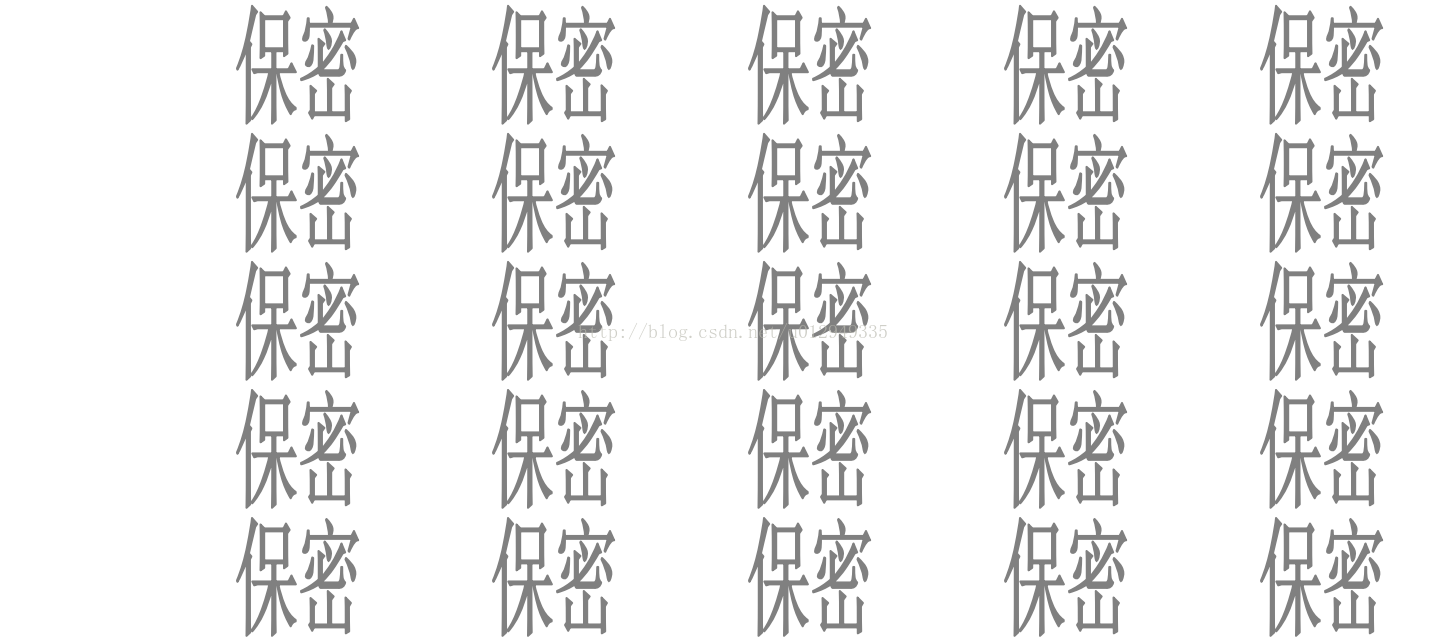