项目GitHub地址 :
https://github.com/FrameReserve/TrainingBoot
https://github.com/FrameReserve/TrainingBoot/releases/tag/0.0.10
Spring Boot启动类,增加@EnableAsync注解配置:
src/main/java/com/training/SpringBootServlet.java
- package com.training;
- import org.springframework.boot.SpringApplication;
- import org.springframework.boot.autoconfigure.SpringBootApplication;
- import org.springframework.boot.builder.SpringApplicationBuilder;
- import org.springframework.boot.web.support.SpringBootServletInitializer;
- import org.springframework.scheduling.annotation.EnableAsync;
- @SpringBootApplication
- @EnableAsync
- public class SpringBootServlet extends SpringBootServletInitializer {
- // jar启动
- public static void main(String[] args) {
- SpringApplication.run(SpringBootServlet.class, args);
- }
- // tomcat war启动
- @Override
- protected SpringApplicationBuilder configure(SpringApplicationBuilder application) {
- return application.sources(SpringBootServlet.class);
- }
- }
扫描二维码关注公众号,回复:
2822655 查看本文章
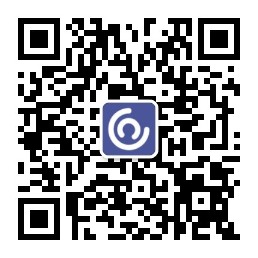
测试:
增加异步方法Service,线程休眠:
- package com.training.async.service.impl;
- import java.util.Random;
- import java.util.concurrent.Future;
- import org.springframework.scheduling.annotation.Async;
- import org.springframework.scheduling.annotation.AsyncResult;
- import org.springframework.stereotype.Service;
- import com.training.async.service.DemoAsyncService;
- @Service
- public class DemoAsyncServiceImpl implements DemoAsyncService {
- public static Random random =new Random();
- @Async
- public Future<String> doTaskOne() throws Exception {
- System.out.println("开始做任务一");
- long start = System.currentTimeMillis();
- Thread.sleep(random.nextInt(10000));
- long end = System.currentTimeMillis();
- System.out.println("完成任务一,耗时:" + (end - start) + "毫秒");
- return new AsyncResult<>("任务一完成");
- }
- @Async
- public Future<String> doTaskTwo() throws Exception {
- System.out.println("开始做任务二");
- long start = System.currentTimeMillis();
- Thread.sleep(random.nextInt(10000));
- long end = System.currentTimeMillis();
- System.out.println("完成任务二,耗时:" + (end - start) + "毫秒");
- return new AsyncResult<>("任务二完成");
- }
- @Async
- public Future<String> doTaskThree() throws Exception {
- System.out.println("开始做任务三");
- long start = System.currentTimeMillis();
- Thread.sleep(random.nextInt(10000));
- long end = System.currentTimeMillis();
- System.out.println("完成任务三,耗时:" + (end - start) + "毫秒");
- return new AsyncResult<>("任务三完成");
- }
- }
调用异步测试测试,查看控制台输出执行顺序:
- package com.training.async.controller;
- import io.swagger.annotations.ApiOperation;
- import java.util.concurrent.Future;
- import javax.annotation.Resource;
- import org.springframework.web.bind.annotation.RequestMapping;
- import org.springframework.web.bind.annotation.RequestMethod;
- import org.springframework.web.bind.annotation.ResponseBody;
- import org.springframework.web.bind.annotation.RestController;
- import com.training.async.service.DemoAsyncService;
- import com.training.core.dto.ResultDataDto;
- @RestController
- @RequestMapping(value="/async")
- public class DemoAsyncController {
- @Resource
- private DemoAsyncService demoAsyncService;
- /**
- * 测试异步方法调用顺序
- */
- @ApiOperation(value="测试异步方法调用顺序", notes="getEntityById")
- @RequestMapping(value = "/getTestDemoAsync", method = RequestMethod.GET)
- public @ResponseBody ResultDataDto getEntityById() throws Exception {
- long start = System.currentTimeMillis();
- Future<String> task1 = demoAsyncService.doTaskOne();
- Future<String> task2 = demoAsyncService.doTaskTwo();
- Future<String> task3 = demoAsyncService.doTaskThree();
- while(true) {
- if(task1.isDone() && task2.isDone() && task3.isDone()) {
- // 三个任务都调用完成,退出循环等待
- break;
- }
- Thread.sleep(1000);
- }
- long end = System.currentTimeMillis();
- System.out.println("任务全部完成,总耗时:" + (end - start) + "毫秒");
- return ResultDataDto.addSuccess();
- }
- }
项目GitHub地址 :
https://github.com/FrameReserve/TrainingBoot
https://github.com/FrameReserve/TrainingBoot/releases/tag/0.0.10
Spring Boot启动类,增加@EnableAsync注解配置:
src/main/java/com/training/SpringBootServlet.java
- package com.training;
- import org.springframework.boot.SpringApplication;
- import org.springframework.boot.autoconfigure.SpringBootApplication;
- import org.springframework.boot.builder.SpringApplicationBuilder;
- import org.springframework.boot.web.support.SpringBootServletInitializer;
- import org.springframework.scheduling.annotation.EnableAsync;
- @SpringBootApplication
- @EnableAsync
- public class SpringBootServlet extends SpringBootServletInitializer {
- // jar启动
- public static void main(String[] args) {
- SpringApplication.run(SpringBootServlet.class, args);
- }
- // tomcat war启动
- @Override
- protected SpringApplicationBuilder configure(SpringApplicationBuilder application) {
- return application.sources(SpringBootServlet.class);
- }
- }
测试:
增加异步方法Service,线程休眠:
- package com.training.async.service.impl;
- import java.util.Random;
- import java.util.concurrent.Future;
- import org.springframework.scheduling.annotation.Async;
- import org.springframework.scheduling.annotation.AsyncResult;
- import org.springframework.stereotype.Service;
- import com.training.async.service.DemoAsyncService;
- @Service
- public class DemoAsyncServiceImpl implements DemoAsyncService {
- public static Random random =new Random();
- @Async
- public Future<String> doTaskOne() throws Exception {
- System.out.println("开始做任务一");
- long start = System.currentTimeMillis();
- Thread.sleep(random.nextInt(10000));
- long end = System.currentTimeMillis();
- System.out.println("完成任务一,耗时:" + (end - start) + "毫秒");
- return new AsyncResult<>("任务一完成");
- }
- @Async
- public Future<String> doTaskTwo() throws Exception {
- System.out.println("开始做任务二");
- long start = System.currentTimeMillis();
- Thread.sleep(random.nextInt(10000));
- long end = System.currentTimeMillis();
- System.out.println("完成任务二,耗时:" + (end - start) + "毫秒");
- return new AsyncResult<>("任务二完成");
- }
- @Async
- public Future<String> doTaskThree() throws Exception {
- System.out.println("开始做任务三");
- long start = System.currentTimeMillis();
- Thread.sleep(random.nextInt(10000));
- long end = System.currentTimeMillis();
- System.out.println("完成任务三,耗时:" + (end - start) + "毫秒");
- return new AsyncResult<>("任务三完成");
- }
- }
调用异步测试测试,查看控制台输出执行顺序:
- package com.training.async.controller;
- import io.swagger.annotations.ApiOperation;
- import java.util.concurrent.Future;
- import javax.annotation.Resource;
- import org.springframework.web.bind.annotation.RequestMapping;
- import org.springframework.web.bind.annotation.RequestMethod;
- import org.springframework.web.bind.annotation.ResponseBody;
- import org.springframework.web.bind.annotation.RestController;
- import com.training.async.service.DemoAsyncService;
- import com.training.core.dto.ResultDataDto;
- @RestController
- @RequestMapping(value="/async")
- public class DemoAsyncController {
- @Resource
- private DemoAsyncService demoAsyncService;
- /**
- * 测试异步方法调用顺序
- */
- @ApiOperation(value="测试异步方法调用顺序", notes="getEntityById")
- @RequestMapping(value = "/getTestDemoAsync", method = RequestMethod.GET)
- public @ResponseBody ResultDataDto getEntityById() throws Exception {
- long start = System.currentTimeMillis();
- Future<String> task1 = demoAsyncService.doTaskOne();
- Future<String> task2 = demoAsyncService.doTaskTwo();
- Future<String> task3 = demoAsyncService.doTaskThree();
- while(true) {
- if(task1.isDone() && task2.isDone() && task3.isDone()) {
- // 三个任务都调用完成,退出循环等待
- break;
- }
- Thread.sleep(1000);
- }
- long end = System.currentTimeMillis();
- System.out.println("任务全部完成,总耗时:" + (end - start) + "毫秒");
- return ResultDataDto.addSuccess();
- }
- }