1.编写一个程序,调用一次printf()函数,把你的名和姓打印在一行。再调用一次printf()函数,把你的名和姓分别打印在两行。然后,再调用两次printf()函数,把你的名和姓打印在一行。输出应如下所示(当然要把示例的内容换成你的名字):
Gustav Mahler //第1次打印的内容
Gustav //第2次打印的内容
Mahler //第3次打印的内容
Gustav Mahler //第4次打印的内容
#include <stdio.h>
int main(void)
{ printf("Gustav Mahler\n");
printf("Gustav\nMahler\n");
printf("Gustav ");
printf("Mahler");
return 0;
}
Output:
3.编写一个程序把你的年龄转换成天数,并显示这两个值。这里不用考虑闰年的问题。
#include <stdio.h>
#define AGE 20
int main(void)
{ int day;
day=AGE*365;
printf("I am %d years old, it is equal to %d days\n",AGE,day);
return 0;
}
Output:
4.编写一个程序,生成以下输出:
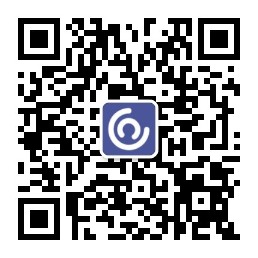
For he's a jolly good fellow!
For he's a jolly good fellow!
For he's a jolly good fellow!
Which nobody can deny!
除了main()函数以外,该程序还要调用两个自定义函数:一个名为jolly(),用于打印前3条消息,调用一次打印一条:另一个函数名为deny(),打印最后一条消息。
#include <stdio.h>
void jolly(void);
void deny(void);
int main(void)
{ jolly(); /*调用函数时括号内不用加void*/
jolly();
jolly();
deny();
return 0;
}
void jolly(void)
{
printf("For he's a jolly good fellow!\n");
}
void deny(void)
{
printf("Which nobody can deny!\n");
}
Output:
5.编写一个程序,生成以下输出:
Brazil, Russia, India, China
India, China,
Brazil, Russia
除了main()以外,该程序还要调用两个自定义函数:一个名为br(),调用一次打印一次"Brazil,Russia";另一个名为ic(),调用一次打印一次"India,China"。其他内容在main()函数中完成。
#include <stdio.h>
void br(void);
void ic(void);
int main(void)
{ br();
printf(", ");
ic();
printf("\n");
ic();
printf("\n");
br();
return 0;
}
void br(void)
{
printf("Brazil,Russia");
}
void ic(void)
{
printf("India,China");
}
Output:
6.编写一个程序,创建一个整型变量toes,并将toes设置为10.程序中还要计算toes的两倍和toes的平方。该程序应打印3个值,并分别描述以示区分。
#include <stdio.h>
int main(void)
{ int toes=10;
printf("toes=%d\n",toes);
printf("the twice of toes is %d\n",toes*2);
printf("the square of toes is %d\n",toes*toes);
return 0;
}
Output:
7.许多研究表明,微笑益处多多。编写一个程序,生成以下格式的输出:
Smile!Smile!Smile!
Smile!Smile!
Smile!
该程序要定义一个函数,该函数要被调用一次打印一次“Smile!”,根据程序的需要使用该函数。
//换行输出的另一种形式putchar('\n')
#include <stdio.h>
void sm(void);
int main(void)
{ sm(); sm(); sm();
putchar('\n');
sm(); sm();
putchar('\n');
sm();
return 0;
}
void sm(void)
{
printf("Smile!");
}
Output:
8.在C语言中,函数可以调用另一个函数。编写一个程序,调用一个名为one_three()的函数。该函数在一行打印单词"one",再调用第2个函数two(),然后在另一行打印单词“three”。two()函数在一行显示单词“two”。main()函数在调用one_three()函数前要打印短语“starting now:”,并在调用完毕后显示短语“done!”。因此,该程序的输出应如下所示:
starting now:
one
two
three
done!
#include <stdio.h>
void one_three(void);
void two(void);
int main(void)
{ printf("starting now:\n");
one_three();
printf("done!\n");
return 0;
}
void one_three(void)
{
printf("one\n");
two();
printf("three\n");
}
void two(void)
{
printf("two\n");
}
Output: