项目环境:
idea 2017 + maven + jdk
一、新建项目
打开 idea 新建一个 Spring Initializr 项目。
二、测试项目
找到 “启动类” 如下图,右键,Run 就行了,由于 springboot 是内置 tomcat ,所以不需要自己配置 tomcat ,跑完之后在地址栏输入:http://localhost:8080/ 访问项目。会出现下面的错误页面,不用担心,这是正常的!!!因为我们都没有写实现类。
新建一个 HelloController,java 的类。
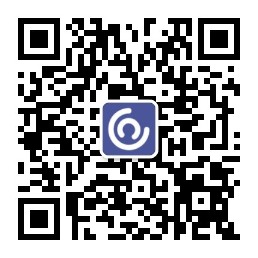
@RestController
public class HelloController {
@RequestMapping(value = "/say",method = RequestMethod.GET)
public String sayHi(){
return "Hello My First SpringBoot!";
}
}
访问成功了~~~~
三、配置 pom.xml 文件
在 dependencies 标签里面添加下面的代码,让 maven 自动导入相关的 jar 包。
<!-- 数据库需要用到的相关 jar 包 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
四、配置 application.yml 文件
由于 application.yml 文件的编程格式比 application.properties 的较简洁。所有我们采用 yml 后缀的文件来配置。
直接在 resource 文件中,把对象的文件删除掉,然后新建 application.yml 文件就行,或者直接改后缀名也行。
然后把下面的配置信息,直接复制粘贴到 application.yml 文件里面,然后修改自己的数据库名字和账号、密码。
server:
#配置端口号
port: 8081
servlet:
#项目访问前缀
context-path: /myspringboot
spring:
datasource:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3306/test?useSSL=false
username: root
password: root
jpa:
hibernate:
ddl-auto: update
show-sql: true
五、连接数据库
springboot 可以直接通过代码来新建表格,下面我们就来新建第一张表。
新建一个 Person.java 类。
就是一个 javabean ,把要生成的表中该有的字段给定义出来。
@Id :这个注解,是把 id 定义为主键
@GeneratedVlaue :这个注解是让 id 自增
@Entity
public class Person {
@Id
@GeneratedValue
private Integer id;
private String name;
private Integer age;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
}
运行启动类(不知道哪个是启动类,翻回去看看)。springboot 项目成功跑起来后,去数据库里面刷新下,就能发现 person 已经创建成功,并且是一张空表。数据库就连接上了,并且新建表成功。至于没有成功的,检查自己的数据库名字有没有写对什么之类的~~~
六、简单的增删查改例子
hibernate 是通过 JPA 来进行数据库的交互的。所以我们需要创建一个继承 JPA 的接口。
新建一个接口类:PersonRepository.java
public interface PersonRepository extends JpaRepository<Person,Integer>{
//自定义接口
//通过年龄来查询
public List<Person> findByAge(Integer age);
}
新建一个web层的类:PersonController.java
@RestController
public class PersonController {
@Autowired
private PersonRepository personRepository;
/**
* 添加一个人的信息
* @param name
* @param age
* @return
*/
@PostMapping(value = "/preson")
public Person personAdd(@PathVariable("id") Integer id,
@RequestParam("name") String name,
@RequestParam("age") Integer age){
Person person = new Person();
person.setId(id);
person.setName(name);
person.setAge(age);
return personRepository.save(person);
}
/**
* 查询所有人的数据
* @return
*/
@GetMapping(value = "/personlist")
public List<Person> personList(){
return personRepository.findAll();
}
/**
* 通过id查询某个人的信息
* @param id
* @return
*/
@GetMapping(value = "/person/{id}")
public Person personFindOne(@PathVariable("id") Integer id){
Person op = personRepository.findById(id).get();
return op;
}
/**
* 更新数据
* @param id
* @param name
* @param age
* @return
*/
@PutMapping(value = "/person/{id}")
public Person updateperson(@PathVariable("id") Integer id,
@RequestParam("name") String name,
@RequestParam("age") Integer age){
Person person = new Person();
person.setId(id);
person.setAge(age);
person.setName(name);
return personRepository.save(person);
}
/**
* 通过 id 删除数据
* @param id
*/
@DeleteMapping(value = "/person/{id}")
public void personDelete(@PathVariable("id") Integer id){
personRepository.deleteById(id);
}
/**
* 自定义通过年龄查询用户信息接口
* @param age
* @return
*/
@GetMapping(value = "/person/age/{age}")
public List<Person> personListByAge( @PathVariable("age") Integer age){
return personRepository.findByAge(age);
}
}
至于数据的测试,推荐使用 Postman 去测试。
看完有什么问题不懂的,可以在评论区提出来,大家讨论一下!!!~~~
附件:
@PathVariable 获取 url 中的数据
@RequestParam 获取请求参数的值
@GetMapping 组合注解
@RequestParam(value="",required=false,defaultValue="0")
这里值得注意的是,默认值为 0 时不能直接写 0 ,要加上引号。