源码地址:https://github.com/cachecats/sell
学习了Spring
、SpringMVC
和 SpringBoot
之后,是时候开启一个实战项目了。
一、技术栈
项目采用前后端分离的方式,前端由 Vue
构建,后端用 SpringBoot
,后端页面采用 Bootstrap + FreeMarker + JQuery
实现。前后端通过 RESTful
风格接口相连。
其中 SpringBoot
涉及到如下技术和知识点:
二、环境搭建
开发工具: IDEA
,Java版本:1.8
,数据库版本:MySql 5.7
1. 打开IDEA,依次点击 File -> New -> Project
,然后最左侧项目类型选择 Spring Initializr
。Project SDK
选择 java1.8,其他的不用动,点击 Next
进入下一步。
填写
Group
和Artifact
,打包方式Packaging
选择 jar,点击Next
进入下一步。
选择依赖。本项目是个web工程,所以最左侧栏目选
Web
,中间栏勾选Web
,点击Next
进入下一步。其他的依赖边开发边添加。
最后选择 项目名和项目地址 ,然后点
Finish
即可
三、pom.xml文件设置
直接给出 pom.xml
代码吧,都是写依赖没啥好说的,直接复制到你项目中就好。
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.solo</groupId>
<artifactId>takeout</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>takeout</name>
<description>微信外卖点餐系统</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.3.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
</dependency>
<dependency>
<groupId>com.github.binarywang</groupId>
<artifactId>weixin-java-mp</artifactId>
<version>2.7.0</version>
</dependency>
<dependency>
<groupId>cn.springboot</groupId>
<artifactId>best-pay-sdk</artifactId>
<version>1.1.0</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
如果你是第一次运行 SpringBoot
项目,下载依赖会耗费很长时间。可以设置阿里的 maven 镜像,但依赖太多还是会花一些时间。
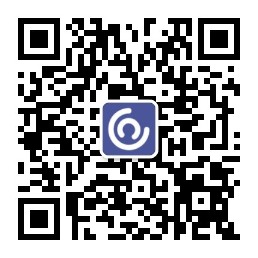
四、数据库设计
1. 项目设计分析
先讲一下项目设计
角色分为两种,买家(手机端)和卖家(PC端)。总体来说就是买家创建订单,并能进行修改等管理操作,卖家可以对订单管理,也可以对商品进行管理。如下图:
关系图如下:
按照角色划分和功能分析,项目中应该有五张表,它们之间的关系如下:
2. 数据表设计
先创建商品和订单相关的4张表,直接贴代码啦
create table `product_info`(
`product_id` varchar(32) not null,
`product_name` varchar(64) not null comment '商品名称',
`product_price` decimal(8,2) not null comment '单价',
`product_stock` int not null comment '库存',
`product_description` varchar(64) comment '描述',
`product_icon` varchar(512) comment '小图',
`category_type` int not null comment '类目编号',
`create_time` timestamp not null default current_timestamp comment '创建时间',
`update_time` timestamp not null default current_timestamp on update current_timestamp comment '修改时间',
primary key (`product_id`)
) comment '商品表';
create table `product_category`(
`category_id` int not null auto_increment,
`category_name` varchar(64) not null comment '类目名字',
`category_type` int not null comment '类目编号',
`create_time` timestamp not null default current_timestamp comment '创建时间',
`update_time` timestamp not null default current_timestamp on update current_timestamp comment '修改时间',
primary key (`category_id`),
unique key `uqe_category_type` (`category_type`)
) comment '类目表';
create table `order_master`(
`order_id` varchar(32) not null,
`buyer_name` varchar(32) not null comment '买家名字',
`buyer_phone` varchar(32) not null comment '买家电话',
`buyer_address` varchar(128) not null comment '买家地址',
`buyer_openid` varchar(64) not null comment '买家微信openid',
`order_amount` decimal(8,2) not null comment '订单总金额',
`order_status` tinyint(3) not null default '0' comment '订单状态,默认0新下单',
`pay_status` tinyint(3) not null default '0'comment '支付状态,默认0未支付',
`create_time` timestamp not null default current_timestamp comment '创建时间',
`update_time` timestamp not null default current_timestamp on update current_timestamp comment '修改时间',
primary key (`order_id`),
key `idx_buyer_openid` (`buyer_openid`)
) comment '订单主表';
create table `order_detail` (
`detail_id` varchar(32) not null,
`order_id` varchar(32) not null,
`product_id` varchar(32) not null,
`product_name` varchar(64) not null comment '商品名称',
`product_price` decimal(8,2) not null comment '商品价格',
`product_quantity` int not null comment '商品数量',
`product_icon` varchar(512) not null comment '商品小图',
`create_time` timestamp not null default current_timestamp comment '创建时间',
`update_time` timestamp not null default current_timestamp on update current_timestamp comment '修改时间',
primary key (`detail_id`),
key `idx_order_id` (`order_id`)
) comment '订单详情表';
以上就是数据库设计,直接执行 Sql 语句即可。
环境搭建和数据库设计就到这里,下篇文章见