刷题打卡:
In a certain course, you take n tests. If you get ai out of bi questions correct on test i, your cumulative average is defined to be
.
Given your test scores and a positive integer k, determine how high you can make your cumulative average if you are allowed to drop any k of your test scores.
Suppose you take 3 tests with scores of 5/5, 0/1, and 2/6. Without dropping any tests, your cumulative average is . However, if you drop the third test, your cumulative average becomes
.
Input
The input test file will contain multiple test cases, each containing exactly three lines. The first line contains two integers, 1 ≤ n ≤ 1000 and 0 ≤ k < n. The second line contains n integers indicating ai for all i. The third line contains npositive integers indicating bi for all i. It is guaranteed that 0 ≤ ai ≤ bi ≤ 1, 000, 000, 000. The end-of-file is marked by a test case with n = k = 0 and should not be processed.
Output
For each test case, write a single line with the highest cumulative average possible after dropping k of the given test scores. The average should be rounded to the nearest integer.
Sample Input
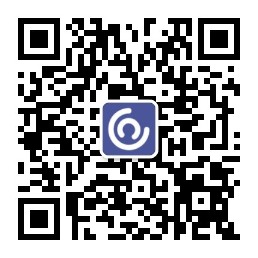
3 1
5 0 2
5 1 6
4 2
1 2 7 9
5 6 7 9
0 0
Sample Output
83
100
Hint
To avoid ambiguities due to rounding errors, the judge tests have been constructed so that all answers are at least 0.001 away from a decision boundary (i.e., you can assume that the average is never 83.4997).
分析:
这是一个很明显的01分数规划问题,具体知识点详见传送门
#include<iostream>
#include<stdio.h>
#include<string>
#include<string.h>
#include<math.h>
#include<stdlib.h>
#include<algorithm>
using namespace std;
typedef long long ll;
double a[1010],b[1010];
double d[1010];
bool cmp(int a,int b)
{
return a>b;
}
int main()
{
int n,k;
while(~scanf("%d%d",&n,&k))
{
if(n==0) break;
for(int i=0;i<n;i++)
scanf("%lf",&a[i]);
for(int i=0;i<n;i++)
scanf("%lf",&b[i]);
double l=0.0,r=1.0,mid;
while(r-l>1e-7)
{
double mid=(l+r)/2;
for(int i=0;i<n;i++)
d[i]=a[i]-mid*b[i];
sort(d,d+n,cmp);
double ans=0;
for(int i=0;i<n-k;i++){
ans+=d[i];
}
if(ans>0) l=mid;
else r=mid;
}
printf("%1.f\n",l*100);
}
return 0;
}
An array of size n ≤ 10 6 is given to you. There is a sliding window of size kwhich is moving from the very left of the array to the very right. You can only see the k numbers in the window. Each time the sliding window moves rightwards by one position. Following is an example:
The array is [1 3 -1 -3 5 3 6 7], and k is 3.
Window position | Minimum value | Maximum value |
---|---|---|
[1 3 -1] -3 5 3 6 7 | -1 | 3 |
1 [3 -1 -3] 5 3 6 7 | -3 | 3 |
1 3 [-1 -3 5] 3 6 7 | -3 | 5 |
1 3 -1 [-3 5 3] 6 7 | -3 | 5 |
1 3 -1 -3 [5 3 6] 7 | 3 | 6 |
1 3 -1 -3 5 [3 6 7] | 3 | 7 |
Your task is to determine the maximum and minimum values in the sliding window at each position.
Input
The input consists of two lines. The first line contains two integers n and k which are the lengths of the array and the sliding window. There are n integers in the second line.
Output
There are two lines in the output. The first line gives the minimum values in the window at each position, from left to right, respectively. The second line gives the maximum values.
Sample Input
8 3
1 3 -1 -3 5 3 6 7
Sample Output
-1 -3 -3 -3 3 3
3 3 5 5 6 7
分析:
单调队列用于求区间最值,使用两个单调队列从头扫到尾即可
#include <iostream>
#include <cstring>
#include <stdio.h>
using namespace std;
#define MXN 1000010
int n, m, rear = -1, front = 0;
int inp[MXN];
int indx[MXN];
int mx[MXN];
int mi[MXN];
int main()
{
while(scanf("%d %d",&n,&m)!=EOF)
{
for (int i = 0; i < n; i++)
scanf("%d",&inp[i]);
for (int i = 0; i < n; ++i)
{
while (rear >= front&&inp[i] <= inp[indx[rear]] )
--rear;
indx[++rear] = i;
if (i - indx[front] == m)
++front;
mi[i] = inp[indx[front]];
}
memset(indx,0,sizeof(indx));
rear=-1;
front=0;
for(int i=0; i<n; i++ )
{
while(rear>=front&&inp[i]>=inp[indx[rear]])--rear;
indx[++rear]=i;
if(i-indx[front]==m)++front;
mx[i]=inp[indx[front]];
}
for(int i=m-1; i<n; i++)
{
if(i!=n-1)
printf("%d ",mi[i]);
else
printf("%d\n",mi[i]);
}
for(int i=m-1; i<n; i++)
{
if(i!=n-1)
printf("%d ",mx[i]);
else
printf("%d\n",mx[i]);
}
}
return 0;
}