介绍
在前后端分离的开发场景下,不可避免的会有前后端联调。在联调阶段,经常会遇到各式各样的问题,比如乱码问题、前端传的数据(字符串、数组、Json对象)后端无法正常解析等问题。
本文希望从源头着手,理清问题的根本原因,快速定位出现问题的位置,让前后端联调得心应手,让甩锅不再那么容易......
HTTP协议
之所以这里会介绍一下HTTP协议,是因为前后端联调离不开HTTP。了解了HTTP协议,有助于更好的理解数据传输的流程,以及更好的分析出到底是在哪个环节出了问题,方便排查。
1. 简介
首先,http是一个无状态的协议,即每次客户端和服务端交互都是无状态的,通常使用cookie来保持状态。
下图为http请求与响应的大致结构(本部分配图均来自于《HTTP权威指南》):
说明:
从上图中可以看出,HTTP请求大致分为三个部分:起始行、首部、主体。在请求起始行里,表面了请求方法、请求地址以及http协议的版本。另外,首部即是我们常说的http header。
2. HTTP method
下面是常用的HTTP请求方法以及介绍:
说明:
- 我们常用的一般为get于post。
- 是否包含主体的意思为请求内容是否带主体。例如,在get方式下由于不带主体,只能使用url的方式传参。
3. Content-type
HTTP传输的内容类型与编码是由Content-Type来控制的,客户端与服务端通过它来识别与解析传输内容。
常见的Content-Type:
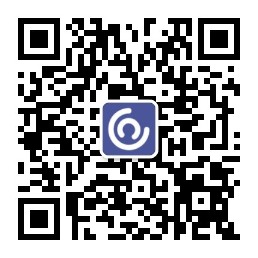
类型 | 说明 |
---|---|
text/html | html类型 |
text/css | css文件 |
text/javascript | js文件 |
text/plain | 文本文件 |
application/json | json类型 |
application/xml | xml类型 |
application/x-www-form-urlencoded | 表单,表单提交时的默认类型 |
multipart/form-data | 附件类型,一般为表单文件上传 |
前面六个为常见的文件类型,后面两个为表单数据提交时类型。我们ajax提交数据时一般为Content-Type:application/x-www-form-urlencoded;charset=utf-8
,以此声明了本次请求的数据格式与数据编码方式。需要额外说明的是,application/x-www-form-urlencoded此种类型比较特殊,数据发送时会把表单数据拼接成类似于a=1&b=2&c=3
的格式,如果数据中存在空格或特殊字符,会进行转换,标准文档在这里,更详细的在[RFC1738]可见。
相关资料:
- Content-type对照表:tool.oschina.net/commons
- Form content types:www.w3.org/TR/html4/in…
- 字符解码时加号解码为空格问题探究:muchstudy.com/2017/12/06/…
- 理解HTTP之Content-Type:homeway.me/2015/07/19/…
4. 字符集与编码
前后端联调之所以需要了解这部分,是因为在前后端的数据交互中,经常会碰到乱码的问题,了解了这块内容,对于解决乱码问题就手到擒来了。
一图胜千言:
在图中,charset的值为iso-8859-6
,详细介绍了一个文字从编码到解码,再到显示的完整过程。
相关资料:
- 字符集列表:www.iana.org/assignments…
- 字符编码详解:muchstudy.com/2016/08/26/…
前端部分
前端部分负责发起HTTP请求,前端常用的HTTP请求工具类有jquery
、axios
、fetch
。实际上jquery与axios的底层都是使用XMLHttpRequest
来发起http请求的,fetch属于浏览器内置的发起http请求方法。
前端ajax请求样例:
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/qs/6.5.1/qs.min.js"></script>
<title>前端发起HTTP请求样例</title>
</head>
<body>
<h2>使用XMLHttpRequest</h2>
<button onclick="xhrGet()">XHR Get</button>
<button onclick="xhrPost()">XHR Post</button>
<h2>使用axios</h2>
<button onclick="axiosGet()">Axios Get</button>
<button onclick="axiosPost()">Axios Post</button>
<h2>使用fetch</h2>
<button onclick="fetchGet()">Fetch Get</button>
<button onclick="fetchPost()">Fetch Post</button>
<script>
// 封装XMLHttpRequest发起ajax请求
let Axios = function({url, method, data}) {
return new Promise((resolve, reject) => {
var xhr = new XMLHttpRequest();
xhr.open(method, url, true);
xhr.onreadystatechange = function() {
// readyState == 4说明请求已完成
if (xhr.readyState == 4 && xhr.status == 200) {
// 从服务器获得数据
resolve(xhr.responseText)
}
};
if(data){
xhr.setRequestHeader('Content-type', 'application/x-www-form-urlencoded;charset=utf-8');
xhr.send(Qs.stringify(data));
//xhr.send("a=1&b=2");
//xhr.send(JSON.stringify(data));
}else{
xhr.send();
}
})
}
// 需要post提交的数据
let postData = {
firstName: 'Fred',
lastName: 'Flintstone',
fullName: '姓 名',
arr:[1,2,3]
}
// 请求地址
let url = 'DemoServlet';
function xhrGet(){
Axios({
url: url+'?a=1',
method: 'GET'
}).then(function (response) {
console.log(response);
})
}
function xhrPost(){
Axios({
url: url,
method: 'POST',
data: postData
}).then(function (response) {
console.log(response);
})
}
function axiosGet(){
// 默认Content-Type = null
axios.get(url, {
params: {
ID: '12345'
}
}).then(function (response) {
console.log(response);
}).catch(function (error) {
console.log(error);
});
}
function axiosPost(){
// 默认Content-Type = application/json;charset=UTF-8
axios.post(url, postData).then(function (response) {
console.log(response);
}).catch(function (error) {
console.log(error);
});
// 默认Content-Type = application/x-www-form-urlencoded
axios({
method: 'post',
url: url,
data: Qs.stringify(postData)
}).then(function (response) {
console.log(response);
});
}
function fetchGet(){
fetch(url+'?id=1').then(res => res.text()).then(data => {
console.log(data)
})
}
function fetchPost(){
fetch(url, {
method: 'post',
body: postData
})
.then(res => res.text())
.then(function (data) {
console.log(data);
})
.catch(function (error) {
console.log('Request failed', error);
});
}
</script>
</body>
</html>
复制代码
相关资料:
- XMLHttpRequest Standard:xhr.spec.whatwg.org/
- Fetch Standard:fetch.spec.whatwg.org/
- XMLHttpRequest介绍:developer.mozilla.org/zh-CN/docs/…
- fetch介绍:developers.google.com/web/updates…
- fetch 简介: 新一代 Ajax API: juejin.im/entry/57451…
- axios源码:github.com/axios/axios
后端部分
这里使用Java平台为样例来介绍后端是如何接收HTTP请求的。在J2EE体系下,数据的接收与返回实际上都是通过Servlet
来完成的。
Servlet接收与返回数据样例:
package com.demo.servlet;
import java.io.BufferedReader;
import java.io.IOException;
import java.util.Enumeration;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class DemoServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public DemoServlet() {
super();
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
System.out.println("-----------------start----------------");
System.out.println("Content-Type:" + request.getContentType());
// 打印请求参数
System.out.println("=========请求参数========");
Enumeration<String> em = request.getParameterNames();
while (em.hasMoreElements()) {
String name = (String) em.nextElement();
String value = request.getParameter(name);
System.out.println(name + " = " + value);
response.getWriter().append(name + " = " + value);
}
// 从inputStream中获取
System.out.println("===========inputStream===========");
StringBuffer sb = new StringBuffer();
String line = null;
try {
BufferedReader reader = request.getReader();
while ((line = reader.readLine()) != null)
sb.append(line);
} catch (Exception e) {
/* report an error */
}
System.out.println(sb.toString());
System.out.println("-----------------end----------------");
response.getWriter().append(sb.toString());
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doGet(request, response);
}
}
复制代码
相关资料:
- servlet的本质是什么,它是如何工作的?:www.zhihu.com/question/21…
- tomcat是如何处理http请求的?:blog.csdn.net/qq_38182963…
结果汇总
请求方式 | method | 请求Content-Type | 数据格式 | 后端收到的Content-Type | 能否通过getParameter获取数据 | 能否通过inputStream获取数据 | 后端接收类型 |
---|---|---|---|---|---|---|---|
XHR | Get | 未设置 | url传参 | null | 能 | 否 | 键值对 |
XHR | Post | 未设置 | json字符串 | text/plain;charset=UTF-8 | 否 | 能 | 字符串 |
XHR | Post | 未设置 | a=1&b=2格式字符串 | text/plain;charset=UTF-8 | 否 | 能 | 字符串 |
XHR | Post | application/x-www-form-urlencoded | a=1&b=2格式字符串 | application/x-www-form-urlencoded | 能 | 否 | 后端收到key为a和b,值为1和2的键值对 |
XHR | Post | application/x-www-form-urlencoded | json字符串 | application/x-www-form-urlencoded | 能 | 否 | 后端收到一个key为json数据,值为空的键值对 |
axios | Get | 未设置 | url传参 | null | 能 | 否 | 键值对 |
axios | Post | 未设置 | json对象 | application/json;charset=UTF-8 | 否 | 能 | json字符串 |
axios | Post | 未设置 | 数组 | application/json;charset=UTF-8 | 否 | 能 | 数组字符串 |
axios | Post | 未设置 | a=1&b=2格式字符串 | application/x-www-form-urlencoded | 能 | 否 | 键值对 |
fetch | Get | 未设置 | url传参 | null | 能 | 否 | 键值对 |
fetch | Post | 未设置 | a=1&b=2格式字符串 | text/plain;charset=UTF-8 | 否 | 能 | a=1&b=2字符串 |
fetch | Post | 未设置 | json对象 | text/plain;charset=UTF-8 | 否 | 能 | 后端收到[object Object]字符串 |
fetch | Post | application/x-www-form-urlencoded;charset=UTF-8 | a=1&b=2格式字符串 | application/x-www-form-urlencoded;charset=UTF-8 | 能 | 否 | 键值对 |
通过上面的表格内容可以发现,凡是使用get或者content-type为application/x-www-form-urlencoded
发送数据,在后端servlet都会默认把数据转换为键值对。否则,需要从输入流中获取前端发送过来的字符串数据,再使用fastJSON等后端工具类转换为Java实体类或集合对象。
联调工具Postman
可以在chrome的应用商店中下载Postman插件,在浏览器中模拟HTTP请求。Postman的界面如下:
说明:
- 发送get请求直接在url上带上参数,接着点击send即可
- 发送post请求,数据有三种传输方式;
form-data
、x-www-form-urlencoded
、raw
(未经加工的)
类型 | Content-Type | 说明 |
---|---|---|
form-data | Content-Type: multipart/form-data | form表单的附件提交方式 |
x-www-form-urlencoded | Content-Type: application/x-www-form-urlencoded | form表单的post提交方式 |
raw | Content-Type: text/plain;charset=UTF-8 | 文本的提交方式 |
原文地址:HTTP协议与前后端联调