/// <summary>
/// 批量导入学生核心代码
/// </summary>
/// <param name="path">excel表文件的绝对路径</param>
/// <param name="schoolKey">学校密钥</param>
/// <returns></returns>
public string ImportPhone(string path, string schoolKey)
{
string cs = ConfigurationManager.ConnectionStrings["NFineDbContext"].ToString();
SqlConnectionStringBuilder scsb = new SqlConnectionStringBuilder(cs);
scsb.MultipleActiveResultSets = true;
cs = scsb.ConnectionString;
var db = new SqlConnection(cs);
db.Open();
SqlTransaction tran = db.BeginTransaction();
OleDbConnection ExcelConn = null;
OleDbCommand comm = null;
OleDbDataReader thisReader = null;
string text = "";
string sql;
try
{
ExcelConn = new OleDbConnection(@"Provider=Microsoft.Ace.OleDb.12.0;Data Source=" + path + ";Extended Properties=Excel 12.0;");
ExcelConn.Open();
comm = new OleDbCommand();
comm.Connection = ExcelConn;
sql = "select 学生姓名,电子卡号,亲情号码,亲情关系 from [亲情号码$] ";//这个是从表格里找
comm.CommandText = sql;
thisReader = comm.ExecuteReader();
int rowIndex = 1; // 行号
while (thisReader.Read())
{
++rowIndex;
string textMsg = "";
OleDbCommand comm_ = new OleDbCommand();
comm_.Connection = ExcelConn;
if (thisReader["学生姓名"] == null
|| string.IsNullOrWhiteSpace(thisReader["学生姓名"].ToString().Trim())
)
{
textMsg += string.Format("<br />第{0}行,学生姓名不能为空", rowIndex);
}
if (thisReader["电子卡号"] == null
|| string.IsNullOrWhiteSpace(thisReader["电子卡号"].ToString().Trim())
)
{
textMsg += string.Format("<br />第{0}行,电子卡号不能为空", rowIndex);
}
else if (thisReader["电子卡号"].ToString().Trim().Length != 10)
{
textMsg += string.Format("<br />第{0}行,电子卡号必须是10位长度", rowIndex);
}
if (thisReader["亲情号码"] == null
|| string.IsNullOrWhiteSpace(thisReader["亲情号码"].ToString().Trim())
)
{
textMsg += string.Format("<br />第{0}行,亲情号码不能为空", rowIndex);
}
else if (thisReader["亲情号码"].ToString().Trim().Length != 11)
{
textMsg += string.Format("<br />第{0}行,亲情号码必须是11位手机号码", rowIndex);
}
if (thisReader["亲情关系"] == null
|| string.IsNullOrWhiteSpace(thisReader["亲情关系"].ToString().Trim())
)
{
textMsg += string.Format("<br />第{0}行,亲情关系不能为空", rowIndex);
}
else if (thisReader["亲情关系"].ToString().Trim().Length > 2)
{
textMsg += string.Format("<br />第{0}行,亲情关系最多只能两个字", rowIndex);
}
if (!string.IsNullOrWhiteSpace(textMsg))
{
text += textMsg;
continue;
}
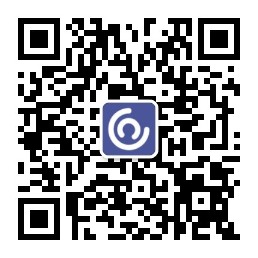
// 检查该电子卡号是否已绑定过该号码
string sqlText = string.Format(@"SELECT [ID]
FROM [FamilyPhone]
WHERE [Card] = '{0}' AND [FamilyPhone] = '{1}'", thisReader["电子卡号"], thisReader["亲情号码"]);
DataTable da = new DataTable();
using (var command = new SqlCommand(sqlText, db))
{
command.CommandType = CommandType.Text; // 设置command的CommandType为指定的CommandType
command.Transaction = tran;
using (SqlDataAdapter adapter = new SqlDataAdapter(command)) // 通过包含查询SQL的SqlCommand实例来实例化SqlDataAdapter
{
adapter.Fill(da); // 填充DataTable
}
}
if (da != null && da.Rows.Count > 0)
{ // 如果已存在,则不用重新绑定
continue;
}
else
{ // 新增操作
sql = string.Format(@"INSERT INTO [FamilyPhone] ([SchoolKey], [Student], [Card], [FamilyPhone], [Family])
VALUES ('{0}', '{1}', '{2}', '{3}', '{4}')", schoolKey.Trim(), thisReader["学生姓名"].ToString().Trim(), thisReader["电子卡号"].ToString().Trim(), thisReader["亲情号码"].ToString().Trim(), thisReader["亲情关系"].ToString().Trim());
SqlCommand cmand = tran.Connection.CreateCommand();
cmand.CommandType = CommandType.Text;
cmand.CommandText = sql;
cmand.Transaction = tran;
cmand.ExecuteNonQuery();
}
}
tran.Commit();
return text;
}
catch (Exception ex)
{
if (tran != null) tran.Rollback();
throw ex;
}
finally
{
db.Close();
thisReader.Dispose();
ExcelConn.Close();
}
}
调用:
/// <summary>
/// 批量导入亲情号码
/// </summary>
/// <param name="SchoolKey">学校密钥</param>
/// <returns></returns>
public ActionResult ImportPhone(string SchoolKey)
{
string msg = "";
string rootPath = HttpContext.Server.MapPath("/");
string afterFileName = "";
rootPath = rootPath.Replace("\\", "/");
try
{
string savePath = rootPath + "UploadFiles/";
if (!Directory.Exists(savePath)) // 判断文件夹是否存在
{
Directory.CreateDirectory(savePath);
}
HttpPostedFileBase file = HttpContext.Request.Files[0]; // 获得指定表单文件域的上传文件
if (file != null)
{
string fileName = file.FileName; // 获得上传上文件的名称,完整名称,如: aa.txt
fileName = fileName.Replace("\\", "/");
int index = fileName.LastIndexOf("/");
fileName = fileName.Substring(index + 1, fileName.Length - (index + 1));
index = fileName.LastIndexOf(".");
string extensionString = fileName.Substring(index, fileName.Length - (index)).ToLower();
// 限制文件格式
if (extensionString.IndexOf(".xlsx") < 0 && extensionString.IndexOf(".xls") < 0)
{
return Success("操作完成", new { Tag = 0, Content = "只支持Excel文件格式" });
}
DateTime dt = DateTime.Now;
afterFileName = string.Format("导入结果_{0}年{1}月{2}日{3}时{4}分{5}秒_{6}", dt.Year, dt.Month, dt.Day, dt.Hour, dt.Minute, dt.Second, System.IO.Path.GetExtension(fileName));
file.SaveAs(savePath + afterFileName);
msg = new FamilyPhoneApp().ImportPhone(savePath + afterFileName, SchoolKey);
}
if (msg.Trim().Equals(""))
{
return Success("操作完成", new { Tag = 1, Content = "导入完成" });
}
else
{
return Success("操作完成", new { Tag = 0, Content = msg });
}
}
catch (Exception ex)
{
return Success("操作完成", new { Tag = 0, Content = ex.Message });
}
}
前端调用:
<script>
function SubmitForm() {
var schoolKey = $.request("pagekey");
$.loading(true, '正在批量导入,时间可能会较长');
$.ajaxFileUpload({
url: "/SchoolPhone/FamilyPhone/ImportPhone?SchoolKey=" + schoolKey,
secureuri: false,
fileElementId: ["ImportData"],
dataType: 'json',
success: function (returnValue) {
$.loading(false);
if (returnValue.data.Tag == 1) {
$.modalAlert(returnValue.data.Content, 'success');
$.currentWindow().$("#gridList").trigger("reloadGrid");
} else {
$("#divError").html(returnValue.data.Content).show();
}
}
});
}
// 下载亲情号码模板
function DownLoadFimlyPhoneTempletFile() {
var f = $('<form action="/SchoolPhone/FamilyPhone/DownLoadFimlyPhoneTempletFile" method="post" id="ExportFimlyPhoneTempletFile"></form>');
f.appendTo(document.body).submit();
try {
document.body.removeChild(f);
} catch (e) { }
}
</script>
<form id="form1">
<div style="padding-top: 20px; margin-right: 20px;">
<table class="form" style="width:100%">
<tr>
<th class="formTitle">选择Excel:</th>
<td>
<input type='file' name='ImportData' id='ImportData' />
</td>
<td>
<a class="btn btn-primary dropdown-text" onclick="SubmitForm()">开始导入数据</a>
<a style="color: blue; text-decoration:underline; cursor: pointer" onclick="DownLoadFimlyPhoneTempletFile(); return false;"><i class="fa fa-file-excel-o"></i>模板文件下载</a>
</td>
</tr>
</table>
</div>
</form>