- Scanner
Scanner scanner = new Scanner(System.in);
String str = scanner.nextLine();//获取字符串
char c = scanner.nextLine().charAt(0);//获取字符
int a = scanner.nextInt();//获取数字
scanner.close();
- String
================编码转换
byte[] send = str.getBytes("gbk");
String不能改变自身长度和内容
赋值修改时是重新分配内存,指向了新的地址
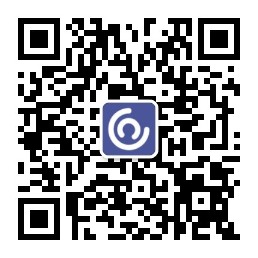
================构造
String str = "abcdef";
String str = new String();
String str = new String(char[]);
String str = new String(String);
================操作
比较操作一般不使用==
if(str.indexOf("bc") >= 0) {//字符串或字符查找}
lastIndexOf(str) 最后一次出现的索引位置
str.startsWith(" ab")//是否以某个字符序列开始
str += "xx";//添加字符
String substr = str.substring(1,4);//从下标1截取到下标3
str = str.toUpperCase();//转换为大写
str = str.replace("ab", "xx");//字符串替换
str = str.trim();//去掉首尾的空格
char[] s = str.toCharArray();//转换为字符数组
String[] s = str.split(" ");//字符串拆分
boolean b = str.contains("xx");//是否包含字符串
- StringBuffer
StringBuffer可以改变自身内容,属于线程安全
StringBuilder适合单线程使用
StringBuffer strb = new StringBuffer("abc");
strb.append("def");
strb.delete(0, 1);//删除第一个字符
strb.insert(3, "xx");//在下标为3的位置插入
strb.setCharAt(1, 'z');//替换下标为1的字符
strb.reverse();//逆序
strb.replace(1, 3, "mmm");//将下标1到2的字符替换
String str = strb.toString();
- System
System.exit(0);//终止当前java虚拟机
long start = System.currentTimeMillis();//返回以毫秒为单位的当前时间
System.gc(); //清理垃圾
获取当前系统的属性
import java.util.*;
Properties p = System.getProperties();
Enumeration pNames = p.propertyNames();
while(pNames.hasMoreElements())
{
String key = (String)pNames.nextElement();
String value = System.getProperty(key);
System.out.println(key+" "+value);
}
- Runtime
打开和关闭其他程序
Runtime tr = Runtime.getRuntime();
Process p = tr.exec("notepad.exe");
p.destroy();
- Thread
=============方式一
继承自 Thread,实现void run()函数,调用start()函数启动线程。
class T extends Thread
{
public void run(){}
}
调用:
new T().start();
new Thread(){
@Override
public void run() {
}
}.start();
=============方式二
类实现Runnable接口,实现函数run。调用时构造Thread类对象,传入该类,调用Thread对象的start函数。
class T implements Runnable {
public void run(){ }
}
调用:
new Thread(new T()).start();
可以在Thread类中传入同一个对象,让多个线程共享同一个对象的内容。
class T implements Runnable {
int count = 10;
public void run() {
while (count > 0) {
Thread t = Thread.currentThread();//获取运行该语句的线程
System.out.println(t.getName() + " count:" + count--);
}
}
}
调用:
T t = new T();
new Thread(t,"window1").start();
new Thread(t,"window2").start();
=============构造方法
Thread()
Thread(实现runnable的对象)
Thread(实现runnable的对象,窗口名)
=============后台线程
创建的线程默认是前台线程,即主线程结束后,线程未运行完也不会结束。在start前调用函数setDaemon可以让线程变成后台线程,当主线程运行结束后也停止。
Thread th = new Thread(new T());
th.setDaemon(true);//在start前调用该语句
th.start();
=============线程休眠
try
{
Thread.sleep(2000);//当前线程休眠2S
}catch(InterruptedException e){}
=============线程优先级
可以设置某个线程的优先级,作为提高程序效率的一种手段。
th.setPriority(Thread.MAX_PRIORITY);
=============线程让步
当某个线程执行到yield函数时,转换成就绪状态,只有与当前线程优先级相同或者更高的才能获得执行机会。
Thread.yield();
=============线程插队
当某个线程中调用其他线程的join方法后,调用的线程被阻塞,直到被调用的线程结束后它才会执行。
th.join();
=============线程同步
多个线程操作同一对象,在该对象类的同步代码块或同步方法中操作相同资源,可以进行互斥。
第一个线程进入synchronized(lock)后占用了锁,第二个线程到synchronized(lock)时阻塞,直到第一个线程结束synchronized块内语句或者其他激活条件时第二个线程才执行synchronized(lock)后一句
同步代码块:
static Object lock = new Object();
public void run()
{
。。。。。
synchronized(lock){ 同步块 }
。。。。。
}
同步方法:
同步方法的锁就是this对象,相当于进入函数后进入synchronized(this)语句块
synchronized void fun(){}
=============多线程通信
lock.wait() 使当前线程放弃同步锁lock,并进入等待,直到其他线程进入此同步锁,并调用notify或者notifyAll方法唤醒该线程为止。
notify唤醒此同步锁上等待的第一个调用wait方法的线程。
notify和wait需要使用锁对象调用,且调用位置在该锁对象由Syncoronized触发的代码块内。
线程1,2公用Stack对象,1线程调用push(),2线程调用pop()。两个线程依靠互斥对象和notiy、wait方法交替执行
Stack stack = new Stack();
new Thread(new Th1(stack)).start();
new Thread(new Th2(stack)).start();
class Stack {
//可以不使用lock对象,直接把push和pop定义成synchronized方法,则两个方法使用进入时使用的是this作为锁
static Object lock = new Object();
void push() throws InterruptedException {
synchronized(lock)
{
int i = 0;
while (true) {
System.out.println("push" + i++);
Thread.sleep(1000);
if (i == 5) {
i = 0;
lock.notify();
lock.wait();
}
}
}
}
void pop() throws InterruptedException {
synchronized (lock) {
int i = 0;
while (true) {
System.out.println("pop...." + i++);
Thread.sleep(1000);
if (i == 5) {
i = 0;
lock.notify();
lock.wait();
}
}
}
}
}
- Math
Math.PI
Math.ceil(1.1)//向上取整 值为2
Math.floor(1.9)//向下取整 值为1
Math.abs(-1)//绝对值
Math.round(1.5)//四舍五入 值为2
Math.random()//生成大于等于0.1小于1的随机数
产生10个[0-100]的随机数
import java.util.Random;
Random r = new Random();
for(int x = 0; x < 10 ; ++x)
{
System.out.println(r.nextInt(100));
}
- Data
import java.util.*;
-
- 获取当前日期
Date cur = new Date();
-
- 格式化日期
SimpleDateFormat sdf=new SimpleDateFormat("yyyy-MM-dd"); //设置日期格式"yyyy-MM-dd HH:mm:ss"
String strCurTime = sdf.format(cur);//将日期按照格式转换字符串
Date da = sdf.parse("2016-02-26"); //解析出一个日期
-
- 日期运算
Calendar c = Calendar.getInstance();
//计算两个日期之间相差的天数
cal.setTime(date);
long time1 = cal.getTimeInMillis();
cal.setTime(dateNow);
long time2 = cal.getTimeInMillis();
long between_days=(time2-time1)/(1000*3600*24);
c.set(Calendar.HOUR, 3);//设置小时为3点
c.add(Calendar.HOUR, 1);//为小时加1
System.out.println(c.get(Calendar.HOUR));//获取小时值
- File
- 基本操作
File ff = new File("D:\\1.txt");
ff.exists();//判断文件或者目录是否存在
ff.isDirectory();//判断是否是文件夹
ff.isFile();//判断是否是文件
ff.createNewFile();//不存在则创建新文件
ff.delete();//删除文件
ff.renameTo(new File("D:\\xx.txt"));//移动文件,也可以修改文件夹名,文件夹必须存在
ff.mkdirs();//创建目录,目录可以多层级创建
ff.mkdir();//创建目录,目录只能是一层新目录
ff.delete();//删除目录,不能删除有子目录的目录
-
- 遍历文件夹和子文件夹
void ShowFile(File ff)
{
if(!ff.isDirectory()) return;
File[] ffList = ff.listFiles();
for(File f : ffList)
{
if (f.isFile()){
String fileName = f.getName();
if(fileName.endsWith(".txt"))
System.out.println(f.getName() + " " + f.getPath());
}
else ShowFile(f);
}
}
-
- 读文件
File ff = new File("D:\\1.txt");
FileInputStream fis = new FileInputStream(ff);
InputStreamReader isr = new InputStreamReader(fis,"UTF-8");
BufferedReader bfr = new BufferedReader(isr);
String line;
while((line = bfr.readLine()) != null)
{
System.out.println(line);
}
bfr.close();
isr.close();
fis.close();
-
- 写文件
File ff = new File("D:\\2.txt");
FileOutputStream fos = new FileOutputStream(ff);
OutputStreamWriter osw = new OutputStreamWriter(fos, "utf-8");
BufferedWriter bfw = new BufferedWriter(osw);
bfw.write("aaaa\r\n");
bfw.write("bbbb\r\n");
bfw.write("ccc");
bfw.close();
osw.close();
fos.close();
- 序列化
//实现序列化接口Serializable(包含serialVersionUID)
//序列化类中的成员必须要支持序列化 static,transient后的变量不能被序列化
//当一个父类实现序列化,子类自动实现序列化,不需要显式实现Serializable接口
class People implements Serializable
{
private static final long serialVersionUID = 1L;
int id;
String name;
People(int id,String name)
{
this.id = id;
this.name = name;
}
}
class PeopleList implements Serializable
{
private static final long serialVersionUID = 1L;//确保序列化和反序列化使用相同id
private ArrayList<People> list = new ArrayList<People>();
void Add(int id , String Name)
{
list.add(new People(id, Name));
}
void Show()
{
for(People p:list) System.out.println(p.id + " " + p.name);
}
}
============序列化
void Save() throws FileNotFoundException, IOException
{
PeopleList pList = new PeopleList();
pList.Add(1, "aa");
pList.Add(2, "bb");
pList.Add(3, "cc");
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("D:\\temp.bin"));
oos.writeObject(pList);
oos.flush();
oos.close();
}
==========反序列化
void Read() throws FileNotFoundException, IOException, ClassNotFoundException
{
ObjectInputStream oin = new ObjectInputStream(new FileInputStream("D:\\temp.bin"));
PeopleList list = (PeopleList ) oin.readObject();
list.Show();
}