将获取token的方法封装到公共类
#java
package date811;
import io.restassured.response.Response;
import org.testng.annotations.Test;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertThat;
import static io.restassured.RestAssured.given;
public class WXUtil {
/*
公共类
*/
private static String corpID = "xxxxx";
private static String corpSecret = "xxxxxxxx";
private static String tokenURL = "https://qyapi.weixin.qq.com/cgi-bin/gettoken";
//获取access_token的方法
public static String getToken(){
Response res = given().param("corpid",corpID).
param("corpsecret",corpSecret).get(tokenURL).prettyPeek();
String token_text = res.getBody().jsonPath().getString("access_token");
assertNotNull(token_text);
return token_text;
}
}
将返回的body封装,便于修改和查看
#java
package date811;
import java.util.Map;
public class WXTextMessage {
/**
* {
* "touser" : "UserID1|UserID2|UserID3",
* "toparty" : "PartyID1|PartyID2",
* "totag" : "TagID1 | TagID2",
* "msgtype" : "text",
* "agentid" : 1,
* "text" : {
* "content" : "你的快递已到,请携带工卡前往邮件中心领取。\n出发前可查看<a href=\"http://work.weixin.qq.com\">邮件中心视频实况</a>,聪明避开排队。"
* },
* "safe":0
* }
*/
private String touser;
private String toparty;
private String totag;
private String msgtype;
private Integer agentid;
private Map<String,String> text;
private Integer safe;
/**
* 生成set和get方法
* 右键选择Generate或者control+enter,Getter and Setter全选
*/
public String getTouser() {
return touser;
}
public void setTouser(String touser) {
this.touser = touser;
}
public String getToparty() {
return toparty;
}
public void setToparty(String toparty) {
this.toparty = toparty;
}
public String getTotag() {
return totag;
}
public void setTotag(String totag) {
this.totag = totag;
}
public String getMsgtype() {
return msgtype;
}
public void setMsgtype(String msgtype) {
this.msgtype = msgtype;
}
public Integer getAgentid() {
return agentid;
}
public void setAgentid(Integer agentid) {
this.agentid = agentid;
}
public Map<String, String> getText() {
return text;
}
public void setText(Map<String, String> text) {
this.text = text;
}
public Integer getSafe() {
return safe;
}
public void setSafe(Integer safe) {
this.safe = safe;
}
}
执行类
#java
package date811;
import io.restassured.RestAssured.*;
import io.restassured.http.ContentType;
import io.restassured.matcher.RestAssuredMatchers.*;
import io.restassured.response.Response;
import org.hamcrest.Matchers.*;
import org.testng.annotations.Test;
import java.util.HashMap;
import java.util.Map;
import static io.restassured.RestAssured.given;
import static org.hamcrest.Matchers.equalTo;
//assertThat方法一定要静态导入
import static org.hamcrest.Matchers.notNullValue;
import static org.junit.Assert.assertThat;
public class GetToken {
@Test
public void post_message() {
String token_text = WXUtil.getToken();
String postURL = "https://qyapi.weixin.qq.com/cgi-bin/message/send";
WXTextMessage wx = new WXTextMessage();
wx.setAgentid(0);
wx.setSafe(0);
wx.setToparty("0");
wx.setMsgtype("text");
Map<String,String> text = new HashMap<>();
text.put("content","你的快递已到,请携带工卡前往邮件中心领取。\\n" +
"出发前可查看<a href=\\\"http://work.weixin.qq.com\\\">" +
"邮件中心视频实况</a>,聪明避开排队");
wx.setText(text);
Response res = given().contentType(ContentType.JSON).body(wx).queryParam("access_token",token_text)
.post(postURL).prettyPeek();
assertThat(res.getBody().jsonPath().getString("errmsg"),equalTo("ok"));
}
}
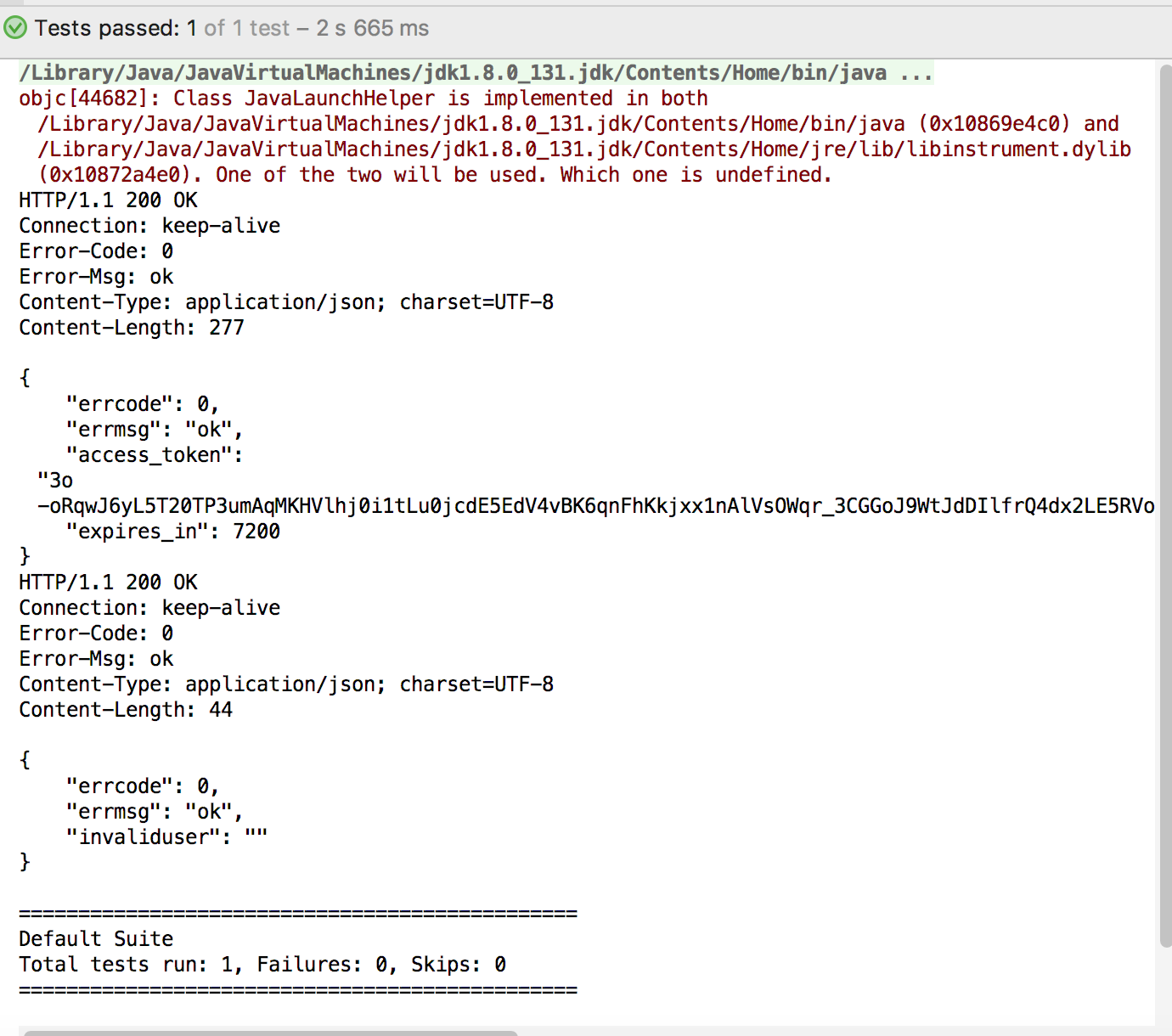