1.读取文件流在控制台显示的方法:
①创建字节输入流或者字符输入流 ②创建int[] 或者byte数组接收字符流或者字节流
read(arr)读入数组-->将数组写入字符串str=new String(arr,0,length)
例如:
public static void main(String[] args) throws Exception {
FileReader fr=new FileReader("F:\\F.txt");
BufferedReader bdr=new BufferedReader(new FileReader("F:\\F.txt"));
char[] arr=new char[10224];
int i=-1;
while((i=bdr.read(arr))!=-1) {
String st=new String(arr,0,i);
System.out.println(st);
}
bdr.close();
System.out.println("----------------------------------------------");
FileInputStream fos=new FileInputStream("F:\\F.txt");
byte[] bt=new byte[1024];
int i1=-1;
while((i1=fos.read(bt))!=-1) {
String str=new String(bt,0,i1);
System.out.println(str);
}
}
2.字节流可以处理所有类型数据,如:图片,MP3,AVI视频文件,而字符流只能处理字符数据。只要是处理纯文本数据,就要优先考虑使用字符流,除此之外都用字节流。
3.两种通过字节流复制文件的方法:
public static void main(String[] args) throws Exception{
// TODO Auto-generated method stub
FileInputStream fis=new FileInputStream("F:\\F.txt");
FileOutputStream fos=new FileOutputStream("F:\\J.txt");
//一个字节一个字节的写入
/*int i=-1;
while((i=fis.read())!=-1) {
fos.write(i);
}
fis.close();
fos.close();
*/
//先些如数组,再从数组写入文件内
byte[] bt=new byte[1024];
int i=0;
while((i=fis.read(bt))!=-1) {
fos.write(bt,0,i);
}
fis.close();
fos.close();
}
4.通过高校的BufferIputStream与BufferedOutputStream复制文件
public static void main(String[] args) throws Exception{
//读取文件
BufferedReader br=new BufferedReader(new FileReader("F:\\F.txt") );
//写入文件
BufferedWriter bw=new BufferedWriter(new FileWriter("F:\\j.txt"));
String str=null;
//读取方式为一行一行的读取,返回类型为字符串String
while((str=br.readLine())!=null) {
bw.write(str);
}
bw.flush();
bw.close();
br.close();
}
注: a.高效流不能直接对文件进行操作,必须通过普通流操作.高效流是从输入流中读取文本,缓冲各个字符,从而实现字符、数组和行的高效读取。 可以指定缓冲区的大小,或者可使用默认的大小。大多数情况下,默认值就足够大了 b.字节流在操作的时候本身是不会用到缓冲区(内存)的,是与文件本身直接操作的,而字符流在操作的时候是使用到缓冲区的,并且可以使用flush方法强制进行刷新缓冲区
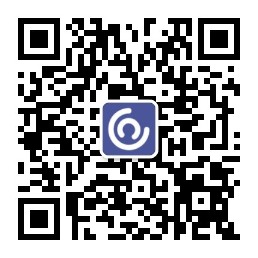
5.
public static void main(String[] args) throws Exception{
//读取键盘字节流,字节流转化为字符流,再转化为缓冲字符流
//使用字符缓冲流是为了实现可以一行一行的读取写入
InputStream is=System.in;
InputStreamReader isr=new InputStreamReader(is);
BufferedReader br=new BufferedReader(isr);
//写入文件数据
PrintWriter pw=new PrintWriter(new FileWriter("F:\\k.txt"),true);
String str=null;
while((str=br.readLine())!=null) {
if(str.equals("Over")) {
break;
}
pw.println(str);
}
pw.flush();
pw.close();
br.close();
}