本节重点
- 了解Python语言的基本特点
- 了解Python语法
- 练习几个练习题
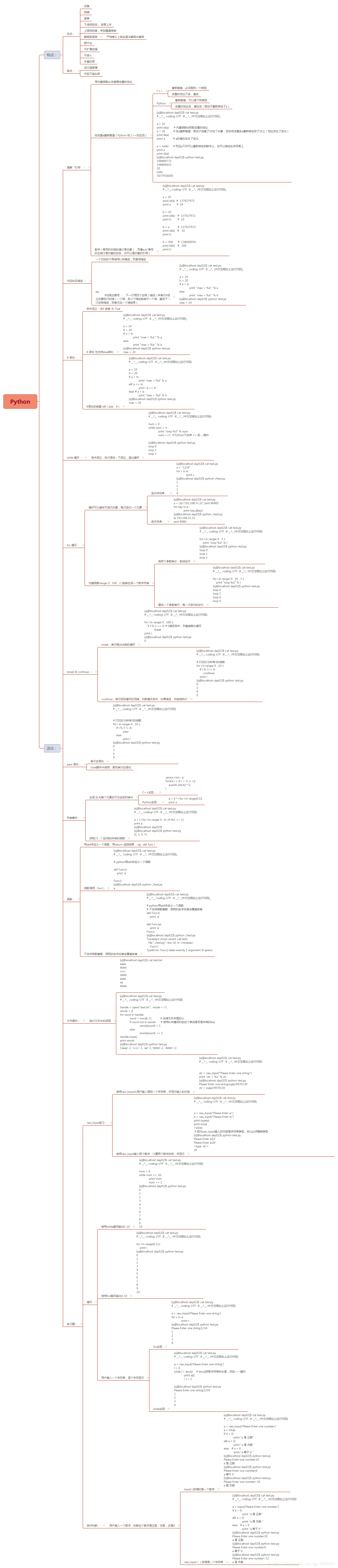
Python语言的基本特点
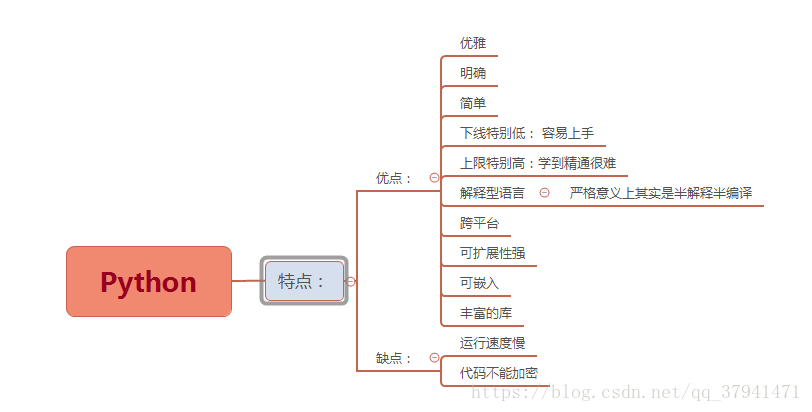
简单操作:
命令行输入:
1. 启动:python (解释器)
2. 退出:ctrl+D (EOF)
3. 写一个Python代码:文件以 .py 为后缀
4. 空格:
1. Python:变量赋值,等号两边空格有没有都可以
2. Shell : 变量赋值,等号两边必须没有空格
Python对于测试人员能做哪些事?
1. 搭建自动化测试框架
2. 搭建持续集成环境
3. 搭建bug状态跟踪平台
语法:
一. 变量赋值:
1. 空格的用法:
1. Python:变量赋值,等号两边空格有没有都可以(尽量加上)
2. Shell : 变量赋值,等号两边必须没有空格
2. 增量赋值:a = a * 10 等价于 a *= 10(和C语言相同)
eg1:
[xj@localhost day01]$ cat test1.py
a = 10
a = a * 10
print a
[xj@localhost day01]$ python test1.py
100
eg2:
[xj@localhost day01]$ cat test1.py
a = 10
a *= 10
print a
[xj@localhost day01]$ python test1.py
100
3. ++ && --
Python不支持++ 和 --
4. 同一个名字变量,可以赋值成不同的类型的值
eg:
[xj@localhost day01]$ cat test1.py
a = 10
a *= 10
print a
a = 'hehe'
print a
[xj@localhost day01]$ python test1.py
100
hehe
二. 变量命名规则:
1. 变量名必须是字母,数字,下划线,但是不能用数字开头( 规则和C语言一样 )
2. 变量名大小写敏感:区分大小写
3. 变量名要做到:"见名知意"
三. 内建函数type可以查看变量的类型
eg:
a = 1
b = 3.14
print type(a)
print type(b)
[xj@localhost day01]$ python ./test.py
<type 'int'>
<type 'float'>
另外,变量的取值范围并没有限制
eg:
[xj@localhost day01]$ cat test1.py
a = 1000000000 * 10000000 * 10000000
print a
[xj@localhost day01]$ python test1.py
100000000000000000000000
四. 还有一个"复数"类型
eg:
a = 10 + 5j
print a
[xj@localhost day01]$ python ./test.py
(10+5j)
五. 字符串
Python中可以用单引号,双引号,三引号 来表示字符串
(另外三引号可以用:三个单引号表示 或者 三个双引号来表示)
Shell 和 Python 的区别:
1. Shell :
1. 单引号:直接取字面值
2. 双引号:会对里面的特殊字符特殊处理或者命令等等
2. Python:两者都是直接取字面值
eg:
[xj@localhost day01]$ cat test2.py
a = 'hehe'
b = "hehe"
c = '''hehe'''
d = """hehe"""
print a
print b
print c
print d
[xj@localhost day01]$ python test2.py
hehe
hehe
hehe
hehe
六. 索引操作符[] & 切片操作符[:]
C语言: 下标如果是负数,其实在C语言中下标都是无符号整型的,所以下标其实值很大,但是编译会通过,结果有可能是乱码
Python: 下标如果为负数,表示的是倒数第几个
(即下标-1取的是数组最后一个元素)
索引规则: 第一个字符索引就是0,最后一个字符索引是len-1(这里指的是下标)
另外:
a[1:] 表示从下标为1的元素到数组最后一个元素
a[:3] 表示从数组第一个元素开始到下表为2(3-1)的元素
eg:
a = "abcd"
print a[0] ,a[1] ,a[2] ,a[3]
print a[-1]
print a[1:3]
print a[1:]
print a[:3]
print a[:-1]
print a[:100]
[xj@localhost day01]$ python ./test.py
a b c d
d
bc
bcd
abc
abc
abcd
七. 字符串拼接:+ & *(用于字符串重复)
eg:
[xj@localhost day01]$ cat test2.py
a = 'hehe'
b = 'haha'
c = a + b
print c
d = a * 4
print d
[xj@localhost day01]$ python test2.py
hehehaha
hehehehehehehehe
八. 没有字符的概念,单个字符也认为是字符串
eg :
[xj@localhost day01]$ cat test2.py
a = 'p'
print type(a)
[xj@localhost day01]$ python test2.py
<type 'str'>
九. 获取字符串长度 & 格式化字符串
1. 获取字符串长度
eg :
[xj@localhost day01]$ cat test2.py
a = 'haha'
print len(a)
[xj@localhost day01]$ python test2.py
4
2. 格式化字符串
eg :
[xj@localhost day01]$ cat test2.py
a = 100
pystr = "a = %d"
result = pystr % a
print result
[xj@localhost day01]$ python test2.py
a = 100
简化后:
[xj@localhost day01]$ cat test2.py
a = 100
print "a = %d" % a
[xj@localhost day01]$ python test2.py
a = 100
十. 布尔类型(bool) :True & False
eg:
a = True
print a
print type(a)
[xj@localhost day01]$ python ./test.py
True
<type 'bool'>
十一. 输入输出
1. 输出:print 可以作为函数 也可以作为语句
(将结果输出到标准输出(显示器)上)
2. 输入:raw_input函数从标准输入(键盘)中获取用户输入
eg :
[xj@localhost day01]$ cat test2.py
num = raw_input('Enter a number:')
print num
[xj@localhost day01]$ python test2.py
Enter a number:10
10
当然print num 如果将print 看做函数,也可以用print(num)输出num
[xj@localhost day01]$ cat test2.py
num = raw_input('Enter a number:')
print(num)
[xj@localhost day01]$ python test2.py
Enter a number:10
10
十二. 注释
1. 单行注释:
2. 中文注释:
Python中默认只支持ASCII码
如果要使用,在代码文件最开头加上
十三. 操作符:+ - * / %(这些都和C语言用法一样)
特殊的几个操作符:
1. ** 表示乘方运算
eg:
[xj@localhost day01]$ cat test2.py
a = 5
b = a ** a
print b
[xj@localhost day01]$ python test2.py
3125
2. "地板除"://
不管操作数为何种数值类型,总是会舍去小数部分,
返回数字序列中比真正的商小的最接近的数字。
下面通过例子来解释:
>>> 1.0/2
0.5
>>> 1.0//2
0.0
很明显地板除将小数点后面的舍去了
>>> -3.6/9
-0.40000000000000002
>>> -3.6//9
-1.0
3. 使用: from __future__ import division 将/变为精确除法(备注:两个_)
eg:
>>> 1/2
0
>>> from __future__ import division
>>> 1/2
0.5
十四. 逻辑运算符 :and or not
eg:
[xj@localhost day01]$ python
Python 2.6.6 (r266:84292, Nov 22 2013, 12:11:10)
[GCC 4.4.7 20120313 (Red Hat 4.4.7-4)] on linux2
Type "help", "copyright", "credits" or "license" for more information.
>>> 2 < 4 and 2 == 4
False
>>> 2 < 4 or 2 == 4
True
>>> not 2 == 4
True
>>>
十五. 列表 / 元组 / 字典
1. 列表 & 元组的区别:
列表:元素值可以修改
eg:
>>> a = [1,2,3,4]
>>> print a
[1, 2, 3, 4]
>>> print a[0]
1
>>> a[0] = 10
>>> print a
[10, 2, 3, 4]
>>>
元组:元素值不可以修改
eg:
>>> a = (1,2,3,4)
>>> print a
(1, 2, 3, 4)
>>> a[0] = 10
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'tuple' object does not support item assignment
>>>
2. 字典
基于哈希表构建(因为增删查改时间复杂度为O(1))
1. 字典和字典之间用逗号,隔开
2. 键值和value用冒号:隔开
eg:
>>> a = {'ip':'127.0.0.1','port':8080}
>>> print a
{'ip': '127.0.0.1', 'port': 8080}
修改vulue:
>>> a['ip'] = '192.168.31.32'
>>> print a
{'ip': '192.168.31.32', 'port': 8080}
插入元素:
>>> a['mask'] = '255.255.255.0'
>>> print a
{'ip': '192.168.31.32', 'mask': '255.255.255.0', 'port': 8080}
for循环
[xj@localhost day02]$ cat test.py
a = "1234"
for c in a:
print c
[xj@localhost day02]$ python ./test.py
1
2
3
4
[xj@localhost day02]$
[xj@localhost day02]$ cat test.py
a = {'ip':'192.168.31.32','port':8080}
for key in a:
print key,a[key]
[xj@localhost day02]$ python ./test.py
ip 192.168.31.32
port 8080
break & continue
breake:
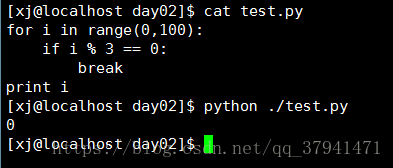
continue:
[xj@localhost day02]$ cat test.py
for i in range(0,100):
if i % 3 != 0:
continue
print i
[xj@localhost day02]$ python ./test.py
0
3
6
9
12
15
18
21
24
27
30
33
36
39
42
45
48
51
54
57
60
63
66
69
72
75
78
81
84
87
90
93
96
99
列表解析
# __*__ coding: UTF -8 __*_ (中文注释加上这行代码)_
# 生成 [0,4]每个元素的平方放到列表中
# C++
# std :: vector<int> a;
# for(int i = 0; i < 5; i++){
# a.push_back(i * i);
#}
#Python
a = [i * i for i in range(0,5)]
print a
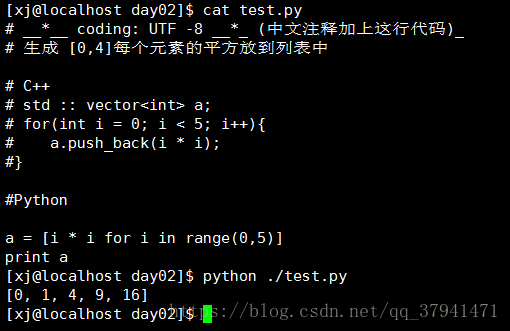
函数
def Add(x,y):
return x + y
print Add(10,20)
print Add("hello","world")
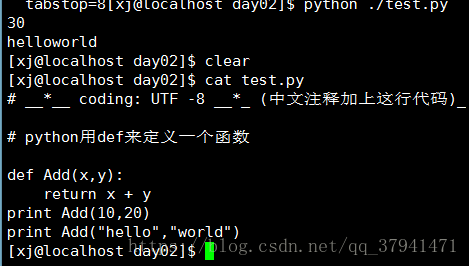
[xj@localhost day02]$ cat test.py
def Func():
print 'a'
Func()
[xj@localhost day02]$ python ./test.py
a
[xj@localhost day02]$ cat test.py
def Func():
print 'a'
def Func(a):
print 'a'
Func()
[xj@localhost day02]$ python ./test.py
Traceback (most recent call last):
File "./test.py", line 10, in <module>
Func()
TypeError: Func() takes exactly 1 argument (0 given)