1、首先数据库部分:字段类型全字符串
2、部署spring、hibernate、struts2、导包建path,新建包,生成pojo及映射文件,目录如下
3、在tools包下编写分页工具bean类
package com.tools;
public class Paging {
private int pageNow;//当前页
private int pageSize;//每页显示数
private int totalRecords;//总记录数
private int totalPages;//总页数
private boolean hasFirst;//是否有首页
private boolean hasLast;//是否有尾页
private boolean hasPre;//是否有前一页
private boolean hasNext;//是否有后一页
public Paging(){}
public Paging(int pageNow,int pageSize,int totalRecords){
this.pageNow=pageNow;
this.pageSize=pageSize;
this.totalRecords=totalRecords; //构造器为变量赋值
}
public int getPageNow() {
return pageNow;
}
public void setPageNow(int pageNow) {
this.pageNow = pageNow;
}
public int getPageSize() {
return pageSize;
}
public void setPageSize(int pageSize) {
this.pageSize = pageSize;
}
public int getTotalRecords() {
return totalRecords;
}
public void setTotalRecords(int totalRecords) {
this.totalRecords = totalRecords;
}
public int getTotalPages() {
if(totalRecords%pageSize!=0)
return totalRecords/pageSize+1;
else
return totalRecords/pageSize;
//return (pageSize+totalRecords-1)/pageSize;//总页数计算
}
public void setTotalPages(int totalPages) {
this.totalPages = totalPages;
}
public boolean isHasFirst() {
if(pageNow==1) //如果当前页是1,则没有首页
return false;
else
return true;
}
public void setHasFirst(boolean hasFirst) {
this.hasFirst = hasFirst;
}
public boolean isHasLast() {
if(pageNow==this.getTotalPages()) //如果当前页是总页数,则没有尾页
return false;
else
return true;
}
public void setHasLast(boolean hasLast) {
this.hasLast = hasLast;
}
public boolean isHasPre() {
if(pageNow==1) //如果当前页是1,则没有前一页
return false;
else
return true;
}
public void setHasPre(boolean hasPre) {
this.hasPre = hasPre;
}
public boolean isHasNext() {
if(pageNow==this.getTotalPages()) //如果当前页是总页数,则没有后一页
return false;
else
return true;
}
public void setHasNext(boolean hasNext) {
this.hasNext = hasNext;
}
@Override
public String toString() {
return "Paging [pageNow=" + pageNow + ", pageSize=" + pageSize + ", totalRecords=" + totalRecords
+ ", totalPages=" + totalPages + ", hasFirst=" + hasFirst + ", hasLast=" + hasLast + ", hasPre="
+ hasPre + ", hasNext=" + hasNext + "]";
}
}
4、在service包下编写业务方法
package com.service;
import java.util.List;
import com.model.Studentsinfo;
public interface InterPagingService {
public int findTotalRecords(); //查找总记录条数业务方法
public List<Studentsinfo> findShowRecords(int pageNow,int pageSize); //查找需要显示的记录业务方法
}
package com.service;
import java.util.List;
import com.dao.InterPagingDao;
import com.model.Studentsinfo;
public class PagingServiceImpl implements InterPagingService {
private InterPagingDao pagingDaoImpl;
public InterPagingDao getPagingDaoImpl() {
return pagingDaoImpl;
}
public void setPagingDaoImpl(InterPagingDao pagingDaoImpl) {
this.pagingDaoImpl = pagingDaoImpl;
}
@Override
public int findTotalRecords() {
return pagingDaoImpl.findTotalRecordsDao();
}
public List<Studentsinfo> findShowRecords(int pageNow,int pageSize){
int skipRecords=(pageNow-1)*pageSize; //数据库查询跳过的记录数
return pagingDaoImpl.findShowRecordsDao(skipRecords,pageSize);
}
}
5、dao包下编写数据库的操作
package com.dao;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
public class BaseDao {
private SessionFactory sessionFactory;
private Session session;
public SessionFactory getSessionFactory() {
return sessionFactory;
}
public void setSessionFactory(SessionFactory sessionFactory) {
this.sessionFactory = sessionFactory;
}
public Session getSession(){
return sessionFactory.openSession();
}
}
package com.dao;
import java.util.List;
import com.model.Studentsinfo;
public interface InterPagingDao {
public int findTotalRecordsDao();
public List<Studentsinfo> findShowRecordsDao(int skipRecords,int pageSize);
}
package com.dao;
import java.util.List;
import org.hibernate.Query;
import org.hibernate.Session;
import org.hibernate.Transaction;
import com.model.Studentsinfo;
public class PagingDaoImpl extends BaseDao implements InterPagingDao {
@Override
public int findTotalRecordsDao() {
try{
Session session=getSession(); //继承中获取session
Transaction ts=session.beginTransaction();//开始事务
return session.createQuery("from Studentsinfo").list().size();//返回记录条数
}catch(Exception e){
e.printStackTrace();
return 0;
}
}
@Override
public List<Studentsinfo> findShowRecordsDao(int skipRecords, int pageSize) {
Session session=getSession();
Transaction ts=session.beginTransaction();
Query query=session.createQuery("from Studentsinfo");
query.setFirstResult(skipRecords);//指定从哪一条记录开始查
query.setMaxResults(pageSize); //设置要查找的记录数
List<Studentsinfo> list=query.list();
ts.commit();
session.close();
return list;
}
}
7、在action包下编写流程控制的action类
扫描二维码关注公众号,回复:
2582641 查看本文章
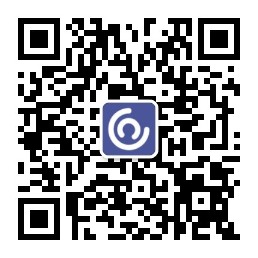
package com.action;
import java.util.List;
import java.util.Map;
import com.model.Studentsinfo;
import com.opensymphony.xwork2.ActionContext;
import com.opensymphony.xwork2.ActionSupport;
import com.service.InterPagingService;
import com.tools.Paging;
public class PagingAction extends ActionSupport{
private int pageNow=1; //初始化为当前页为1,通过请求可更改
private int pageSize=4; //每页显示数为4,同上
private InterPagingService pagingServiceImpl;
public String execute()throws Exception{
pageNow=getPageNow(); //获取要设置的当前页值
pageSize=getPageSize(); //获取要设置的每页记录数
int totalRecords=pagingServiceImpl.findTotalRecords();//获取查找到的总记录数
//判断用户可能输入的当前页是否超出范围,超出重置为1
if(pageNow<1 || pageNow>((pageSize+totalRecords-1)/pageSize))
pageNow=1;
Map request=(Map)ActionContext.getContext().get("request");
Paging paging=new Paging(pageNow,pageSize,totalRecords);//获取分页对象
List<Studentsinfo> list=pagingServiceImpl.findShowRecords(pageNow, pageSize);
//System.out.println(list);
//System.out.println(paging);
request.put("list",list);
request.put("paging", paging);
return SUCCESS;
}
public InterPagingService getPagingServiceImpl() {
return pagingServiceImpl;
}
public void setPagingServiceImpl(InterPagingService pagingServiceImpl) {
this.pagingServiceImpl = pagingServiceImpl;
}
public int getPageNow() {
return pageNow;
}
public void setPageNow(int pageNow) {
this.pageNow = pageNow;
}
public int getPageSize() {
return pageSize;
}
public void setPageSize(int pageSize) {
this.pageSize = pageSize;
}
}
6、配置文件web.xml、struts.xml、applicationContext.ml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1">
<filter>
<filter-name>struts2</filter-name>
<filter-class>org.apache.struts2.dispatcher.FilterDispatcher</filter-class>
</filter>
<filter-mapping>
<filter-name>struts2</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/classes/applicationContext.xml</param-value>
</context-param>
</web-app>
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<package name="default" extends="struts-default">
<!-- 分页action -->
<action name="paging" class="pagingAction">
<result name="success">/paging.jsp</result>
</action>
</package>
<!-- <constant name="struts.i18n.encoding" value="gb2312"></constant> -->
</struts>
<?xml version="1.0" encoding="UTF-8"?>
<beans
xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.1.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd" xmlns:tx="http://www.springframework.org/schema/tx">
<bean id="dataSource"
class="org.apache.commons.dbcp.BasicDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"></property>
<property name="url" value="jdbc:mysql://localhost:3306/"></property>
<property name="username" value="root"></property>
<property name="password" value="root123"></property>
</bean>
<bean id="sessionFactory"
class="org.springframework.orm.hibernate4.LocalSessionFactoryBean">
<property name="dataSource">
<ref bean="dataSource" />
</property>
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">
org.hibernate.dialect.MySQLDialect
</prop>
</props>
</property>
<property name="mappingResources">
<list>
<value>com/model/Studentsinfo.hbm.xml</value></list>
</property></bean>
<bean id="transactionManager"
class="org.springframework.orm.hibernate4.HibernateTransactionManager">
<property name="sessionFactory" ref="sessionFactory" />
</bean>
<tx:annotation-driven transaction-manager="transactionManager" />
<bean id="baseDao" class="com.dao.BaseDao">
<property name="sessionFactory"><ref bean="sessionFactory"></ref></property>
</bean>
<bean id="pagingDaoImpl" class="com.dao.PagingDaoImpl" parent="baseDao"></bean>
<bean id="pagingServiceImpl" class="com.service.PagingServiceImpl">
<property name="pagingDaoImpl" ref="pagingDaoImpl"></property>
</bean>
<bean id="pagingAction" class="com.action.PagingAction">
<property name="pagingServiceImpl" ref="pagingServiceImpl"></property>
</bean>
<bean id="paging" class="com.tools.Paging"></bean>
</beans>
7、编写jsp页面输出
<%@ page language="java" import="java.util.*,com.tools.Paging" pageEncoding="utf-8"%>
<%@ taglib prefix="s" uri="/struts-tags" %>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>ssh2 paging</title>
</head>
<script src=js/jquery-3.0.0.min.js></script>
<script type="text/javascript">
function pageSizeChange(){
var v_pageSize=$("#pageSizeButt").val()
document.location.href="paging.action?pageSize="+v_pageSize+"&pageNow=1";
}
$(function(){
<%
Paging pageInfo=(Paging)request.getAttribute("paging");
int vPageSize=3;
if(pageInfo!=null)
vPageSize=pageInfo.getPageSize();
%>
$("#pageSizeButt").val("<%=vPageSize%>")
})
</script>
<style>
table{
height: 100px;
margin: 80px auto;
margin-bottom:20px;
text-align: center;
}
caption{
margin: 30px;
}
thead th,td{
width:100px;
}
ul{
position: fixed;
left: 27%;
top: auto;
text-decoration: none;
}
li{
margin:5px;
list-style: none;
display: inline;
}
#goButt{
width:30px;
}
#dropPages{
width: 65px;
height: 23px;
}
a{text-decoration:none;}
</style>
<body>
<s:form action="paging" theme="simple">
<s:submit value="sumbit"/>
</s:form>
<table style="border-bottom:gainsboro solid 1px;border-top:gainsboro solid 1px;">
<caption>学生信息表</caption>
<thead>
<th>学号</th>
<th>姓名</th>
<th>籍贯</th>
<th>专业</th>
<th>电话</th>
</thead>
<s:iterator value="#request.list" id="stu">
<tr>
<td><s:property value="#stu.sno" /></td>
<td><s:property value="#stu.name" /></td>
<td><s:property value="#stu.residence" /></td>
<td><s:property value="#stu.major" /></td>
<td><s:property value="#stu.phone" /></td>
</tr>
</s:iterator>
</table>
<s:form action="paging" method="post" theme="simple">
<ul>
<s:set name="page" value="#request.paging"/><!-- 获取分页信息 -->
<li>
<s:if test="#page.hasFirst">
<s:a href="paging.action?pageNow=1">
<input type="button" value="首页" />
</s:a>
</s:if>
</li>
<li>
<s:if test="#page.hasPre">
<a href="paging.action?pageNow=<s:property value="#page.pageNow-1"/>">
<input type="button" value="上一页" />
</a>
</s:if>
</li>
<li>
共<s:property value="#page.pageNow"/>/<s:property value="#page.totalPages"/>页
转到<s:textfield name="pageNow" size="1"/>页
<s:submit value="Go" id="goButt"/>
</li>
<li>每页
<select name="pageSize" onchange="pageSizeChange()" id="pageSizeButt">
<option value="3">3</option>
<option value="4">4</option>
<option value="5">5</option>
<option value="10">10</option>
</select>
条记录
</li>
<li>
<s:if test="#page.hasNext">
<a href="paging.action?pageNow=<s:property value="#page.pageNow+1"/>">
<input type="button" value="下一页" />
</a>
</s:if>
</li>
<li>
<s:if test="#page.hasLast">
<a href="paging.action?pageNow=<s:property value="#page.totalPages"/>">
<input type="button" value="尾页" />
</a>
</s:if>
</li>
</ul>
</s:form>
</body>
</html>
8、测试结果
起初访问jsp页面没有数据,后台也没有报错。调试发现action类没有执行。原因是jsp没有发送请求,可通过修改添加空表单,点击提交链接到action完成初始化(后来才想到其实可以直接访问action完成初始化)。
实现的跳转页功能也用了表单action属性。
实现设置每页最大显示数用了select标签的onchange()函数功能,select显示的值稍微多绕了些才知道可以通过嵌入Java代码获取page对象拿到pageSize属性值,完成当前记录数的显示。
跳转到第二页(其中Go是表单提交)
点击尾页
修改每页十条,当前页会设置为1
如果想下载代码查看,请戳这里