- 中缀表达式 转 后缀表达式 举例示意图:
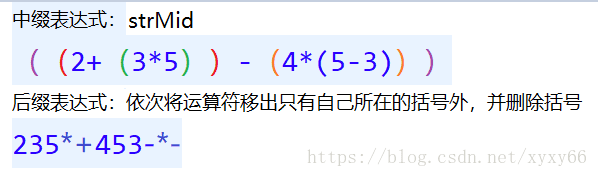
- 全部代码:
package ShunXuZhan;
import java.util.Arrays;
class Constant {
/**
* 表示加法
*/
public static final int OPERATORS_PRIO_PLUS_IN = 4;
public static final int OPERATORS_PRIO_SUB_IN = 4;
public static final int OPERATORS_PRIO_MULTY_IN = 2;
public static final int OPERATORS_PRIO_DIV_IN = 2 ;
public static final int OPERATORS_PRIO_LEFT_BRAK_IN = 10;
public static final int OPERATORS_PRIO_PLUS_OUT = 5 ;
public static final int OPERATORS_PRIO_SUB_OUT = 5;
public static final int OPERATORS_PRIO_MULTY_OUT = 3;
public static final int OPERATORS_PRIO_DIV_OUT = 3;
public static final int OPERATORS_PRIO_LEFT_BRAK_OUT = 1;
public static final int OPERATORS_PRIO_RIGHT_BRAK_OUT = 10;
public static final int OPERATORS_PRIO_ERROR = -1;
}
public class TestM11 {
public static int Get_Prio(char opera,boolean instack)//char类型的一个字符,布尔类型进栈
{
int prio = Constant.OPERATORS_PRIO_ERROR;
if(instack)
{
switch(opera)
{
case '+':
prio = Constant.OPERATORS_PRIO_PLUS_IN;
break;
case '-':
prio = Constant.OPERATORS_PRIO_SUB_IN;
break;
case '*':
prio = Constant.OPERATORS_PRIO_MULTY_IN;
break;
case '/':
prio = Constant.OPERATORS_PRIO_DIV_IN;
break;
case '(':
prio = Constant.OPERATORS_PRIO_LEFT_BRAK_IN;
break;
default:
prio = Constant.OPERATORS_PRIO_ERROR;
break;
}
}
else
{
switch(opera)
{
case '+':
prio = Constant.OPERATORS_PRIO_PLUS_OUT;
break;
case '-':
prio = Constant.OPERATORS_PRIO_SUB_OUT;
break;
case '*':
prio = Constant.OPERATORS_PRIO_MULTY_OUT;
break;
case '/':
prio = Constant.OPERATORS_PRIO_DIV_OUT;
break;
case '(':
prio = Constant.OPERATORS_PRIO_LEFT_BRAK_OUT;
break;
case ')':
prio = Constant.OPERATORS_PRIO_RIGHT_BRAK_OUT;
break;
default:
prio = Constant.OPERATORS_PRIO_ERROR;
break;
}
}
return prio;
}
public static void strMidToLast(String strMid,char[] strLast){
char[] stack=new char[strMid.length()];
int top=0;
int len=strMid.length();
int i=0;
int j=0;
int prioIn;
int prioOut;
while(i!=len){
if(Character.isDigit(strMid.charAt(i))){
strLast[j++]=strMid.charAt(i);
i++;
}else{
if(top==0){
stack[top++]=strMid.charAt(i);
i++;
}else{
prioIn=Get_Prio(stack[top-1],true);
prioOut=Get_Prio(strMid.charAt(i),false);
if(prioIn < prioOut){
strLast[j++]=stack[--top];
}else if(prioIn==prioOut){
top--;
i++;
}else{
stack[top++]=strMid.charAt(i);
i++;
}
}
}
}
while(top > 0){
strLast[j++]=stack[--top];
}
}
public void show(char[] strLast){
for(int i=0;i<strLast.length;i++){
System.out.print(strLast[i]+" ");
}
System.out.println();
}
public static void main(String[] args) {
String strMid="2+3*5-4*(5-3)";
char[] strLast=new char[strMid.length()];
strMidToLast(strMid,strLast);
System.out.println(Arrays.toString(strLast));
}
}
- 输出结果:

public static void strMidToLast(String strMid,char[] strLast){}
方法的逻辑图解释:
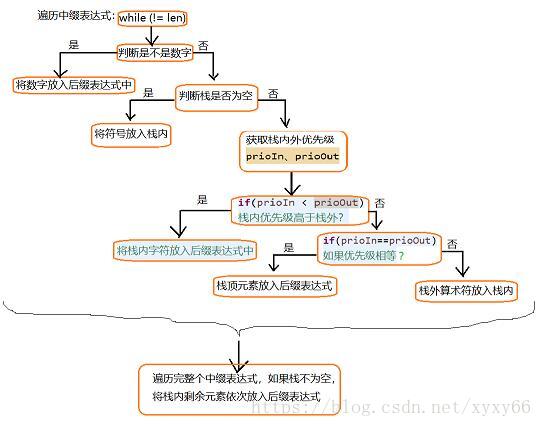