1、线程的调度:
线程的调度有两种模式,分别为:
a.分时调度:即所有线程轮流获得CPU的使用权,平均分配每个线程占用的CPU的时间片;
b.抢占式调度:即让优先级高的线程优先占用CPU,相同优先级则先随机选择一个线程占用CPU。Java虚拟机默认采用抢占式调度模型。
2、线程的优先级
线程的优先级用整数1~10表示,数字越大,优先级越高;默认优先级为5.
3、线程的控制
a.线程休眠(sleep());
b.线程让步(yield());
c.线程插队(join());
d.后台线程或守护线程(setDaemon(true));
扫描二维码关注公众号,回复:
2553427 查看本文章
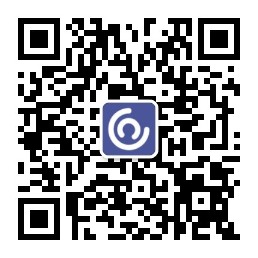
e.线程终止(interrupt(),stop());(其中stop()方法太暴力了,不安全,已过时,不过还能用哦)。
4、多线程之小球运动控制
功能实现:
a.通过设置两个布尔常量控制两个线程对象的开始、暂停与重启;
b.两种方式控制线程对象的关闭(布尔变量与调用interrupt()方法)
c.try{}catch(){}及内部类的简单应用。
效果图:
代码实现:
主界面:
package com.Liao.ball0704v1;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.Graphics;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
public class MainUI extends JFrame {
private static final long serialVersionUID = 1L;
private JButton jbu0, jbu1, jbu2;
private ThreadBall tb1,tb2;
private Thread t1,t2;
public static void main(String[] args) {
MainUI ui = new MainUI();
ui.init();
}
public void init() {
this.setTitle("小球运动");
this.setSize(700, 700);
this.setLocationRelativeTo(null);
this.setDefaultCloseOperation(3);
this.setLayout(new FlowLayout());
//设置背景颜色
this.getContentPane().setBackground(Color.WHITE);
this.setResizable(false);
// 线程的启动与关闭
jbu0 = new JButton("运行线程");
jbu0.setPreferredSize(new Dimension(120, 40));
this.add(jbu0);
// 控制线程1
jbu1 = new JButton("启动线程1");
jbu1.setPreferredSize(new Dimension(120, 40));
this.add(jbu1);
// 控制线程2
jbu2 = new JButton("启动线程2");
jbu2.setPreferredSize(new Dimension(120, 40));
this.add(jbu2);
this.setVisible(true);
Graphics g = this.getGraphics();
//创建Ball对象
Ball b1=new Ball(300,300,g);
Ball b2=new Ball(200,400,g);
//创建ThreadBall对象
tb1=new ThreadBall(b1);
tb2=new ThreadBall(b2);
//创建线程1
t1=new Thread(tb1,"线程1");
t2=new Thread(tb2,"线程2");
// 创建监听器
ActionListener al = new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("点击按钮了");
String str = e.getActionCommand();
control(str);
}
};
jbu0.addActionListener(al);
jbu1.addActionListener(al);
jbu2.addActionListener(al);
}
//控制线程
private void control(String str) {
if ("运行线程".equals(str)) {
jbu0.setText("结束线程");
tb1.setIsstop(false);
tb1.setIspaused(true);
tb2.setIsstop(false);
tb2.setIspaused(true);
//启动线程
t1.start();
t2.start();
}
if("结束线程".equals(str)){
//通过布尔变量控制第一个线程的结束
tb1.setIspaused(true);
tb1.setIsstop(true);
//通过调用interrupt()方法结束第二个线程
t2.interrupt();
//关闭窗口及相关组件
//this.dispose();
}
if("启动线程1".equals(str)){
jbu1.setText("暂停线程1");
tb1.setIspaused(false);
}
if("暂停线程1".equals(str)){
jbu1.setText("启动线程1");
tb1.setIspaused(true);
}
if("启动线程2".equals(str)){
jbu2.setText("暂停线程2");
tb2.setIspaused(false);
}
if("暂停线程2".equals(str)){
jbu2.setText("启动线程2");
tb2.setIspaused(true);
}
}
}
小球线程:
package com.Liao.ball0704v1;
public class ThreadBall implements Runnable {
private Boolean ispaused, isstop;
private Ball ball;
public ThreadBall(Ball ball) {
this.ball = ball;
}
public void setIspaused(Boolean ispaused) {
this.ispaused = ispaused;
}
public void setIsstop(Boolean isstop) {
this.isstop = isstop;
}
public void run() {
while (!isstop) {
// 输出线程是否暂停
System.out.println(Thread.currentThread().getName() + "ispaused:" + ispaused);
while (!ispaused) {
// 延时
try {
Thread.sleep(20);
} catch (InterruptedException ie) {
//出现ie异常执行下面语句
System.out.println(Thread.currentThread().getName() + "中断");
return;
}
ball.draw();
}
}
}
}
球类(小球的绘制及移动):
package com.Liao.ball0704v1;
import java.awt.Color;
import java.awt.Graphics;
public class Ball {
private int x, y, diaMeter = 50, dx = 5, dy = 5;
private Graphics g;
public Ball(int x, int y, Graphics g) {
this.x = x;
this.y = y;
this.g = g;
}
public void draw() {
g.drawLine(100, 100, 500, 500);
g.setColor(Color.WHITE);
g.fillOval(x, y, diaMeter, diaMeter);
// 小球运动
x += dx;
y += dy;
g.setColor(Color.black);
g.fillOval(x, y, diaMeter, diaMeter);
// 控制小球在窗体内运动
if (x >= 650 || x <= 0) {
dx = -dx;
}
if (y >= 650 || y <= 0) {
dy = -dy;
}
}
}
至此,多线程的两种创建方式及基本控制的学习已经结束了,接下来将正式步入线程的殿堂了。