一、使用 @ ExceptionHandler 注解
使用该注解有一个不好的地方就是:进行异常处理的方法必须与抛异常的方法在同一个Controller里面。
/**
* 删除
*/
@Transactional(propagation=Propagation.REQUIRED)
@RequestMapping(value = "UserDel/{codes}", method = { RequestMethod.GET,
RequestMethod.POST }, produces = "application/json; charset=UTF-8")
@ResponseBody
public ResultUtil usersDel(HttpSession session, HttpServletRequest request, @PathVariable("codes") String codes) {
try {
userService.deleteByPrimaryKey(codes);
return ResultUtil.ok("操作成功!");
} catch (Exception e) {
e.printStackTrace();
throw new CustomException("删除出错了!!!");
}
}
/*** 异常处理
*/
@ExceptionHandler(CustomException.class)
@ResponseBody
public ResultUtil handleRRException(CustomException e){
ResultUtil r = new ResultUtil();
r.setCode(e.getCode());
r.setMsg(e.getMessage());
return r;
}
二、使用 @ControllerAdvice+ @ ExceptionHandler 注解
import org.apache.shiro.authz.AuthorizationException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.dao.DuplicateKeyException;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestController;
import common.utils.ResultUtil;
/**
*
* @Desc: 异常处理器
* 请确保此类能被扫描到并装载进Spring容器中
*/
@RestController
@ControllerAdvice
public class RRExceptionHandler {
private Logger logger = LoggerFactory.getLogger(getClass());
/**
* 自定义异常
*/
@ExceptionHandler(RRException.class)
public ResultUtil handleRRException(RRException e){
ResultUtil r = new ResultUtil();
r.setCode(e.getCode());
r.setMsg(e.getMessage());
return r;
}
@ExceptionHandler(DuplicateKeyException.class)
public ResultUtil handleDuplicateKeyException(DuplicateKeyException e){
logger.error(e.getMessage(), e);
return ResultUtil.error("数据库中已存在该记录");
}
@ExceptionHandler(AuthorizationException.class)
public ResultUtil handleAuthorizationException(AuthorizationException e){
logger.error(e.getMessage(), e);
return ResultUtil.error("没有权限,请联系管理员授权");
}
@ExceptionHandler(Exception.class)
public ResultUtil handleException(Exception e){
logger.error(e.getMessage(), e);
return ResultUtil.error();
}
}
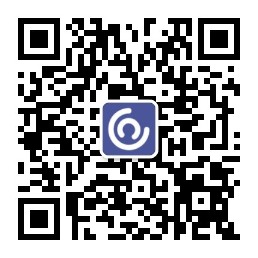
三、实现 HandlerExceptionResolver 接口
import java.io.IOException;import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.stereotype.Component;
import org.springframework.web.servlet.HandlerExceptionResolver;
import org.springframework.web.servlet.ModelAndView;
import com.yuanguo.common.utils.CustomException;
/**
* 全局异常捕获
* @author Mingchenchen
*
*/
@Component
public class GlobleExceptionHandler implements HandlerExceptionResolver {
private Logger logger = LoggerFactory.getLogger(getClass());
@Override
public ModelAndView resolveException(HttpServletRequest request, HttpServletResponse response, Object handler,
Exception ex) {
if (ex instanceof CustomException) {
logger.info("GlobleExceptionHandler catch exception : InvalidParamException");
ModelAndView mv = new ModelAndView();
/* 使用response返回 */
response.setStatus(HttpStatus.OK.value()); // 设置状态码
response.setContentType(MediaType.APPLICATION_JSON_VALUE); // 设置ContentType
response.setCharacterEncoding("UTF-8"); // 避免乱码
try {
response.getWriter().write("你想返回的JSON字符串");
} catch (IOException e) {
e.printStackTrace();
}
return mv;
}
return null;
}
}
/**
* 自定义异常类
*/
public class CustomException extends RuntimeException {
private static final long serialVersionUID = 1L;
private String msg;
private int code = 500;
public CustomException(String msg) {
super(msg);
this.msg = msg;
}
public CustomException(String msg, Throwable e) {
super(msg, e);
this.msg = msg;
}
public CustomException(String msg, int code) {
super(msg);
this.msg = msg;
this.code = code;
}
public CustomException(String msg, int code, Throwable e) {
super(msg, e);
this.msg = msg;
this.code = code;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
public int getCode() {
return code;
}
public void setCode(int code) {
this.code = code;
}
}
/**
* 自定义返回结果封装类
*/
public class ResultUtil implements Serializable {
private static final long serialVersionUID = 1L;
private Integer code;
private String msg;
private Long count;
private Object data;
public ResultUtil() {
super();
}
public ResultUtil(Integer code) {
super();
this.code = code;
}
public ResultUtil(Integer code, String msg) {
super();
this.code = code;
this.msg = msg;
}
public Integer getCode() {
return code;
}
public void setCode(Integer code) {
this.code = code;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
public Long getCount() {
return count;
}
public void setCount(Long count) {
this.count = count;
}
public Object getData() {
return data;
}
public void setData(Object data) {
this.data = data;
}
public static ResultUtil ok(){
return new ResultUtil(0);
}
public static ResultUtil ok(Object list){
ResultUtil result = new ResultUtil();
result.setCode(0);
result.setData(list);
return result;
}
public static ResultUtil ok(String msg){
ResultUtil result = new ResultUtil();
result.setCode(0);
result.setMsg(msg);
return result;
}
public static ResultUtil ok(Integer code,Object list,String msg){
ResultUtil result = new ResultUtil();
result.setCode(0);
result.setData(list);
result.setMsg(msg);
return result;
}
public static ResultUtil error(){
return new ResultUtil(500,"出错啦!");
}
public static ResultUtil error(String msg){
return new ResultUtil(500,msg);
}
}