链接:Codeforces 911G - Mass Change Queries
大意:给你n个数,处理q次询问,每次询问要求将l-r之间值为x的数修改为y,最后输出q次询问之后的数列。
扫描二维码关注公众号,回复:
2503747 查看本文章
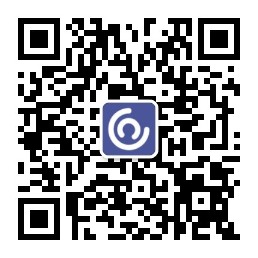
思路:可以利用线段树减少时间复杂度,对于每个节点,储存该节点对应区间内每个数对应的值。
代码:
#include<iostream>
using namespace std;
#define SIZE 200000
inline int lson(int ord) { return ord << 1; }
inline int rson(int ord) { return ord << 1 | 1; }
struct stn
{
int left, right;
int change_to[101];
};
int value[SIZE];
stn nodes[SIZE << 2];
void build(int ord, int l, int r)
{
nodes[ord].left = l, nodes[ord].right = r;
for (int i = 0; i < 101; i++)
nodes[ord].change_to[i] = i;
if (l == r)
return;
int mid = (l + r) >> 1;
build(lson(ord), l, mid);
build(rson(ord), mid + 1, r);
}
inline void push_down(int ord)
{
for (int i = 1; i <= 100; i++)
{
nodes[lson(ord)].change_to[i] = nodes[ord].change_to[nodes[lson(ord)].change_to[i]];
nodes[rson(ord)].change_to[i] = nodes[ord].change_to[nodes[rson(ord)].change_to[i]];
}
for (int i = 1; i <= 100; i++)
nodes[ord].change_to[i] = i;
}
void change_between(int ord, int l, int r, int& x, int& y)
{
if (l == nodes[ord].left && nodes[ord].right == r)
{
for (int i = 1; i <= 100; i++)
if (nodes[ord].change_to[i] == x)
nodes[ord].change_to[i] = y;
return;
}
int mid = (nodes[ord].left + nodes[ord].right) >> 1;
push_down(ord);
if (r <= mid)
change_between(lson(ord), l, r, x, y);
else if (mid < l)
change_between(rson(ord), l, r, x, y);
else
{
change_between(lson(ord), l, mid, x, y);
change_between(rson(ord), mid + 1, r, x, y);
}
}
int get_val(int ord, int pos)
{
if (nodes[ord].left == pos && pos == nodes[ord].right)
return nodes[ord].change_to[value[nodes[ord].left]];
if (pos <= (nodes[ord].left + nodes[ord].right) >> 1)
return nodes[ord].change_to[get_val(lson(ord), pos)];
else
return nodes[ord].change_to[get_val(rson(ord), pos)];
}
int main()
{
int n, q, l, r, x, y;
while (scanf("%d", &n) != EOF)
{
for (int i = 1; i <= n; i++)
scanf("%d", &value[i]);
memset(nodes, 0, sizeof(nodes));
build(1, 1, n);
scanf("%d", &q);
for (int i = 0; i < q; i++)
{
scanf("%d %d %d %d", &l, &r, &x, &y);
change_between(1, l, r, x, y);
}
for (int i = 1; i <= n; i++)
printf("%d%c", get_val(1, i), i == n ? '\n' : ' ');
}
return 0;
}