实验环境是VS 2015、MSSQL Server 2008、windows 10
一、创建项目
通过VS创建一个MVC5项目EntityFrameworkExtension
二、安装Entity Framework
通过nuget添加Entity Framework到项目,当前版本为6.2.0
三、创建实体类Student
public class Student { public string Id { get; set; } public string Name { get; set; } public int Age { get; set; } }
四、添加数据库连接和上下文
在web.config中添加数据库链接
<connectionStrings> <add name="EfDefault" connectionString="Server=.;Database=CodeFirstApp;Integrated Security=SSPI" providerName="System.Data.SqlClient"/> </connectionStrings>
创建上下文EfContext
public class EfContext : DbContext { public EfContext():base("name=EfDefault") { } private DbSet<Student> Students { get; set; } }
在这里,DbContext是所有基于EF的上下文基类,通过它可以访问到数据库中的所有表。上面的代码中调用了父类的构造函数,并且传入了一个键值对,键是name,值是FirstCodeFirstApp
五、启用迁移
在程序包管理器控制台用命令进行开启迁移
PM> Enable-Migrations
将自动生成迁移文件夹Migrations和Configuration.cs
namespace EntityFrameworkExtension.Migrations { using System; using System.Data.Entity; using System.Data.Entity.Migrations; using System.Linq; internal sealed class Configuration : DbMigrationsConfiguration<EntityFrameworkExtension.Entities.EfContext> { public Configuration() { AutomaticMigrationsEnabled = false; } protected override void Seed(EntityFrameworkExtension.Entities.EfContext context) { // This method will be called after migrating to the latest version. // You can use the DbSet<T>.AddOrUpdate() helper extension method // to avoid creating duplicate seed data. } } }
这时自动迁移是被关闭的,需要手动迁移
PM> add-migration InitDb
将自动在Migrations生成迁移代码文件xxxx_迁移名称.cs,如201804160820076_InitDb.cs
使用命令进行手动迁移
扫描二维码关注公众号,回复:
25028 查看本文章
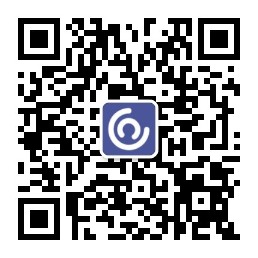
PM> update-database -verbose
verbose可以查看命令的执行过程
Using StartUp project 'EntityFrameworkExtension'. Using NuGet project 'EntityFrameworkExtension'. Specify the '-Verbose' flag to view the SQL statements being applied to the target database. Target database is: 'CodeFirstApp' (DataSource: ., Provider: System.Data.SqlClient, Origin: Configuration). Applying explicit migrations: [201804160820076_InitDb]. Applying explicit migration: 201804160820076_InitDb. CREATE TABLE [dbo].[Students] ( [Id] [nvarchar](128) NOT NULL, [Name] [nvarchar](max), [Age] [int] NOT NULL, CONSTRAINT [PK_dbo.Students] PRIMARY KEY ([Id]) ) CREATE TABLE [dbo].[__MigrationHistory] ( [MigrationId] [nvarchar](150) NOT NULL, [ContextKey] [nvarchar](300) NOT NULL, [Model] [varbinary](max) NOT NULL, [ProductVersion] [nvarchar](32) NOT NULL, CONSTRAINT [PK_dbo.__MigrationHistory] PRIMARY KEY ([MigrationId], [ContextKey]) ) INSERT [dbo].[__MigrationHistory]([MigrationId], [ContextKey], [Model], [ProductVersion]) VALUES (N'201804160820076_InitDb', N'EntityFrameworkExtension.Migrations.Configuration', 此处省略 , N'6.2.0-61023') Running Seed method.
然后刷新数据库即可看到创建的表,其中__MigrationHistory是EF自动生成的迁移日志表
参考文章:http://www.cnblogs.com/farb/p/FirstCodeFirstApp.html