Spring框架总结(3)
其他
2018-07-30 18:30:26
阅读次数: 0
AOP&代理模式
- AOP:面向切面编程
- 将分散在各个方法中的相同的功能(日志处理,权限控制)提取出来,并在运行时动态的将切面注入到各方法中;
- 它是OOP的有力补充,OOP是纵向的抽象,AOP是横向的抽象
- 业务代码变得简洁
- 便于维护
- 代理模式
- 解决的问题:无法直接访问某个目标时(没有权限;找不到目标对象),可以使用代理模式
- 与装饰模式相似,但解决的问题(初衷)不一样
- 题外话:装饰模式
- 给一个已知的类动态的增加一个功能,这个类能直接访问;按照以前的方式,我们可以创建一个子类就可以解决了,但是这样做会使类的数量急剧膨胀
- 最典型的用法:流(流量),一个流没有这个功能,我们可以再套一层流,从而增加了一个功能;前提是这个流是可以访问到的
- 三个角色:
- 抽象角色:
- 真实角色
- 代理角色
- 三者关系(类图)
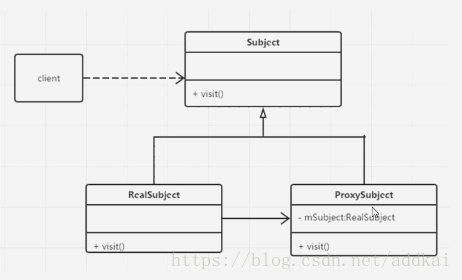
- 存在的问题:每一个真实角色都得有一个代理角色与之对应
- 解决的方法:动态代理
- 动态代理
- 为每个真实对象动态生成一个与之匹配的代理对象
- 相同接口
- Proxy类:根据接口,真实对象和调用处理器来生成代理对象
- InvocationHandler接口:调用处理器(重难点)
- JDK动态代理的缺点:
- 必须提供一个接口
- 若不想使用接口,可使用cglib通过创建子类实现动态代理(Spring两者都用)
- AOP的术语
- 增强(advice):也叫通知;新增的功能(代码段)
- 连接点(Joinpoint):程序执行的某个特定的位置;Spring只支持方法(method)
- 切点(pointcut):具体的连接点;通常用一个表达式表示切点
- 目标对象(Target)
- 织入(weaving):将增强添加到目标对象切点的过程
- 编译期
- 加载期
- 运行期
- 代理对象(Proxy):向目标对象中加入增强后产生的新对象
- 切面(Aspect):增强 + 切点
- AOP的提供者
- AspectJ:开源的AOP框架,支持在三个时期加入增强
- SpringAOP:Spring自带的AOP,在运行期加入增强(JDB动态代理,Cglib)
- JBossAOP
- AspectJ的实现
- 加入aspect依赖包
- 加入<aop:aspectj-autoproxy>
- 创建切面 @Component,@Aspect
- 增强(通知):@Before,@After,@Around,@AfterReturning,@AfterThrowing
- 切点:execution(* com.oracle.proxy.*.*(..)) [ 详见下面代码 ]
- 大量繁琐代码来袭(注意:test 中创建houser的方法有变化 [ houser上转型为接口对象 ],因为JDK动态代理必须提供接口的限制)
-
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:util="http://www.springframework.org/schema/util"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-4.3.xsd">
<context:component-scan base-package="com.oracle.proxy"></context:component-scan>
<aop:aspectj-autoproxy></aop:aspectj-autoproxy>
</beans>
-----------------------------------------------------------------------------------------
Houser.java
package com.oracle.proxy;
import org.springframework.stereotype.Component;
@Component
public class Houser implements Rentable{
@Override
public void rent(int money) {
System.out.println("我是房东,收取房费:"+money);
}
@Override
public int sum(int a) {
return (1+a)*a/2;
}
}
-----------------------------------------------------------------------------------------
LogAspect.java
package com.oracle.proxy;
import java.util.Arrays;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.springframework.stereotype.Component;
@Component
@Aspect
public class LogAspect {
@Before("execution(* com.oracle.proxy.*.rent(..))")
public void before(JoinPoint point) {
System.out.println("记录日志开始");
System.out.println("目标对象:"+point.getTarget());
System.out.println("参数:"+Arrays.toString(point.getArgs()));
System.out.println("方法名:"+point.getSignature().getName());
}
@After("execution(* com.oracle.proxy.*.rent(..))")
public void after() {
System.out.println("记录日志结束");
}
}
-----------------------------------------------------------------------------------------
Rentable.java
package com.oracle.proxy;
public interface Rentable {
public void rent(int money);
public int sum(int a);
}
-----------------------------------------------------------------------------------------
Test.java
package com.oracle.test;
import org.junit.After;
import org.junit.Before;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.oracle.proxy.Rentable;
public class Test {
ApplicationContext factory = new ClassPathXmlApplicationContext("applicationContext.xml");
@Before
public void setUp() throws Exception {
}
@After
public void tearDown() throws Exception {
}
@org.junit.Test
public void test() {
Rentable houser = factory.getBean(Rentable.class);
houser.rent(100);
houser.sum(100);
}
}
转载自blog.csdn.net/addkai/article/details/81202332