ListView是Android开发中最常用到的高级控件,ListView与其他View最不寻常的是可以动态的显示数据,即可以在内存中动态的开辟空间!
下面以一个小的项目为例:
步骤一:
首先,创建一个ListData类,存放你即将在ListView显示的内容这里以存放id和“保养项”为属性名,代码如下:
/** * Created by Boy Baby on 2018/4/9. */ public class ListData { //保养项目编号 private String id; public String getId() { return id; } public void setId(String id) { this.id = id; } //项目名 private String repaire_project_name; public String getRepaire_project_name() { return repaire_project_name; } public void setRepaire_project_name(String repaire_project_name) { this.repaire_project_name = repaire_project_name; } }
步骤二:
新建一个xml页面做插件,ListView中的每一行就是一个xml页面,我们起名listview.xml 代码如下:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="horizontal" > <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <TextView android:layout_width="50dp" android:layout_height="40dp" android:id="@+id/tv_id" android:layout_weight="1" android:paddingLeft="20dp"/> <TextView android:layout_width="50dp" android:layout_height="40dp" android:id="@+id/tv_project" android:layout_weight="10"/> <Button android:layout_width="60dp" android:layout_height="wrap_content" android:text="完成" android:id="@+id/btn_succeed"/> <Button android:layout_width="60dp" android:layout_height="wrap_content" android:text="报修" android:id="@+id/btn_repaire"/> </LinearLayout> </LinearLayout>
视图(如下图):
步骤三:
我们配置一个BaseAdapterl类名:ListViewAdaper.java适配器(用来将listview.xml适配到MainActivity.XML页面进行动态显示)代码如下:
/** * Created by apple on 2018/4/4. */ public class ListViewAdapter extends BaseAdapter { //上下文 private Context context; //数据集合 private List<ListData> list; LayoutInflater inflater; /** * 功能:构造函数 * @param c 上下文对象 * @param list 值集合 */ public ListViewAdapter(Context c,List<ListData> list){ //创建时传递过来的窗口 context=c; //要显示的数据集 this.list=list; //inflater用来获取listView.xml中定义的控件,通过java代码来访问这两个控件(调用布局文件) inflater=(LayoutInflater) c.getSystemService(Context.LAYOUT_INFLATER_SERVICE); } @Override public int getCount() { // TODO Auto-generated method stub return list.size(); } @Override public Object getItem(int arg0) { // TODO Auto-generated method stub return list.get(arg0); } @Override public long getItemId(int arg0) { // TODO Auto-generated method stub return 0; } public List<ListData> getlistdata() { //返回listview列表相关数据集合 return list; } /** * * @param position1 listView行号 * @param arg1 listview.xml * @param arg2 * @return */ @Override public View getView(final int position1, View arg1, ViewGroup arg2) { // TODO Auto-generated method stub if(arg1==null) { //view对象如果为空,通过xml文件创建view对象 arg1=inflater.inflate(R.layout.listview, null); } //关联显示保养项目的TextView控件 TextView tv_id=(TextView) arg1.findViewById(R.id.tv_id); TextView tv_project=(TextView) arg1.findViewById(R.id.tv_project); //显示保养项目序号 tv_id.setText(list.get(position1).getId()); //显示保养项目内容 tv_project.setText(list.get(position1).getRepaire_project_name()); //完成提交和保修提交按钮 Button btn_succeed=(Button)arg1.findViewById(R.id.btn_succeed); Button btn_repaire=(Button)arg1.findViewById(R.id.btn_repaire); //完成保养监听事件(广播发送在主页面进行上传服务器) btn_succeed.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { //将用户点击的ListView的按钮广播到MaintenanceActivity Intent intent = new Intent(); intent.setAction("sendUpMsgb"); intent.putExtra("tv_project", list.get(position1).getId()); context.sendBroadcast(intent); } }); //报修监听事件 btn_repaire.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { //广播该按钮一行的保养项 Intent intent = new Intent(); intent.setAction("sendUpMsgx"); intent.putExtra("tv_project", list.get(position1).getRepaire_project_name()); context.sendBroadcast(intent); } }); return arg1; }
步骤四:
在MainActivity.xml中的布局代码如下:
扫描二维码关注公众号,回复:
2456232 查看本文章
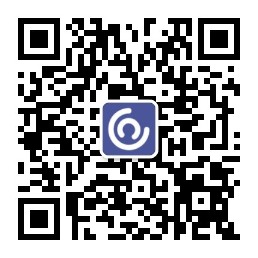
<!--ListView--> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="horizontal"> <ListView android:layout_width="match_parent" android:layout_height="match_parent" android:layout_marginEnd="8dp" android:id="@+id/listview"/> </LinearLayout>
步骤五:
在MainActivity.java中做BaseAdapter的关联,代码如下:
public class MaintenanceActivity extends Activity {
private ListView listView;
protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
listView =(ListView) findViewById(R.id.listview); //创建ListViewAdapter对象并初始化 ListViewAdapter listViewAdapter = new ListViewAdapter(MaintenanceActivity.this,data); //在listView控件设置ListViewAdapter对象 listView.setAdapter(listViewAdapter);}}
我们可以通过动态接收服务器回传的json数据进行动态解析,存放到ListData中去,即可在页面进行动态显示了!
代码如下:
/** * 功能:解析JSON保养项保存到ListData类中 并存到List中取出逐条显示 */ public void Display_Maintenance(){ //接受Intent页面传值(解析JSON) Bundle b = getIntent().getExtras(); String str = b.getString("equitement_Maintenance"); JSONArray jsonArray=null; //动态填充数据 try { JSONObject jsonObject=new JSONObject(str); String content=jsonObject.getString("contents"); jsonArray=new JSONArray(content); }catch (Exception e){ e.printStackTrace(); } for (int i = 0; i <jsonArray.length() ; i++) { try { JSONObject temp=(JSONObject) jsonArray.get(i); ListData listData=new ListData(); listData.setId(Integer.toString(i+1)); listData.setRepaire_project_name(temp.getString("content")); data.add(listData); } catch (JSONException e) { e.printStackTrace(); } } }