模板是一个包含响应文本的文件,其中包含用占位变量表示的动态部分。使用真实值替换变量,再返回最终得到的响应字符串,叫渲染。为了渲染模板,Flask使用Jinja2的模板引擎。
3.1 Jinja2模板引擎
最简单的Jinja2模板是一个包含响应文本的文件
index.html
<h1>Hello World!</h1>
user.html
<h1>Hello,{{ name }}!</h1>
3.1.1 渲染模板
Flask默认在程序文件夹中的templates子文件夹中找模板。
C:\Windows\System32\flasky\templates
hello.py
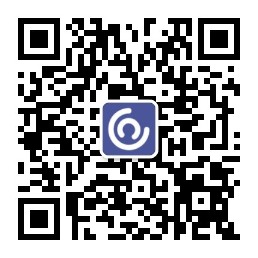
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/user/<name>')
def user(name):
return render_template('user.html', name=name)
Flask提供的render_template函数第一个参数为模板的文件名,第二个参数为键值对(模板中变量对应的真实值)
3.1.2 变量
{{ name }}结构表示一个变量,是一种特殊的占位符。Jinja2支持所有类型的变量。
<p>A value from a dictionary:{{ mydict[‘key’] }}.</p>
<p>A value from a list:{{ mylist[‘3’] }}.</p>
<p>A value from an object’s method:{{ myobj.somemethod() }}.</p>
可以使用过滤器修改变量,过滤器名添加在变量名后,中间竖线分隔
Hello,{{ name|capitalize }}
以首字母大写形式显示变量name的值
常用过滤器
safe:渲染值时不转义,注意:不可在不可信的值上使用safe过滤器
capitalize:值的首字母转换成大写,其他小写
lower:值转换成小写
upper:值转换成大写
title:值中每个单词的首字母转换成大写
trim:把值的首尾空格去掉
striptags:渲染之前把值中所有的HTML标签删掉
3.1.3 控制结构
3.1.3.1 条件控制语句
{% if user %}
Hello,{{ user }}!
{% else %}
Hello,Stranger!
{% endif %}
3.1.3.2 for循环
<ul>
{% for comment in comments %}
<li>{{ comment }}</li>
{% endfor %}
</ul>
3.1.3.3 宏
{% macro render_comment(comment) %}
<li>{{ comment }}</li>
{% end macro %}
<ul>
{% for comment in comments %}
{{ render_comment(comment) }}
{% endfor %}}
</ul>
为了重复使用宏,可单独保存在文件中,使用时在模板中导入
{% import ‘macro.html’ as macros %}
<ul>
{% for comment in comments %}
{{ macros.render_comment(comment) }}
{% endfor %}
</ul>
3.1.3.4 重复使用的模板代码片段
写入单独的文件,再包含再所有模板中
{% include ‘common.html’ %}
3.1.3.5 模板继承
基模板
base.html
<html>
<head>
{% block head %}
<title>{% block title %}{% endblock %} - My Application</title>
{% endblock %}
</head>
<body>
{% block body %}
{% endblock %}
</body>
</html>
基模板的衍生模板
{% extends “base.html” %}
{% block title %}Index{% endblock %} //title包含在head所以要先声明
{% block head %}
{{ super() }}
<style>
</style>
{% endblock %}
{% block body %}
<h1>Hello,World!</h1>
{% endblock %}
extends指令声明模板衍生自base.html。基模板的3个块被重新定义,其中head块的内容使用super()获取原来的内容。
3.2 使用Flask-Bootstrap集成Twitter Bootstrap
Bootstrap是Twitter开发的一个开源客户端框架,不涉及服务器。服务器需要提供引用了Bootstrap层叠样式表(CSS)和JavaScript文件的HTML响应。
我们使用Flask-Bootstrap的Flask扩展集成Bootstrap
pip stall flask-bootstrap
3.2.1 Flask扩展的初始化
from flask_bootstrap import Bootstrap
bootstrap = Bootstrap(app)
templates/user.html
{% extends "bootstrap/base.html" %}
{% block title %}Flasky{% endblock %}
{% block navbar %}
<div class="navbar navbar-inverse" role="navigation">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle" data-toggle="collapse" data-target=".navbar-collapse">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<a class="navbar-brand" href="/">Flasky</a>
</div>
<div class="navbar-collapse collapse">
<ul class="nav navbar-nav">
<li><a href="/">Home</a></li>
</ul>
</div>
</div>
</div>
{% endblock %}
{% block content %}
<div class="container">
<div class="page-header">
<h1>Hello, {{ name }}!</h1>
</div>
</div>
{% endblock %}
3.3 自定义错误页面
Flask允许程序使用给予模板的自定义错误页面。常见错误代码:404,客户端请求未知页面或路由时显示;500,有未处理的异常时显示。
@app.errorhandler(404)
def page_not_found(e):
return render_template('404.html'),404
@app.errorhandler(500)
def internal_server_error(e):
return render_template('500.html'),500
1.templates/user.html
{% extends "base.html" %}
{% block title %}Flasky{% endblock %}
{% block page_content %}
<div class="page-header">
<h1>Hello, {{ name }}!</h1>
</div>
{% endblock %}
2. .templates/404.html
{% extends "base.html" %}
{% block title %}Flasky - Page Not Found{% endblock %}
{% block page_content %}
<div class="page-header">
<h1>Not Found</h1>
</div>
{% endblock %}
3.4 链接
具有多个路由的程序都需要链接来连接不同页面。直接编写简单路由的URL链接,在包含可变部分的动态路由会很困难,而且对路由产生不必要的依赖关系。
Flask提供了url_for()辅助函数,可以使用程序URL映射中保存的信息生成URL。
url_for()函数最简单的用法是以视图函数名作为参数,返回URL。例如:url_for(‘index’)得到的结果/;url_for(‘index’,_external=True)返回绝对地址,http://localhost:5000/。
url_for()生成动态地址时,将动态部分作为关键字参数传入。url_for(‘user’,name=’john’,_external=True)返回http://localhost:5000/user/john。还可以将额外参数添加到查询字符串,url_for(‘user’,page=2)返回/?page=2
3.5 静态文件
Flask默认到程序根目录中名为static的子目录中寻找静态文件
在基模板中放置favicon.ico图标
{% block head %}
{{ super() }}
<link rel="shortcut icon" href="{{ url_for('static', filename='favicon.ico') }}" type="image/x-icon">
<link rel="icon" href="{{ url_for('static', filename='favicon.ico') }}" type="image/x-icon">
{% endblock %}
3.6 Flask-Moment本地化日期和时间
安装pip install flask-moment
1.hello.py
from datetime import datetime
from flask_moment import Moment
moment = Moment(app)
@app.route('/')
def index():
return render_template('index.html', current_time=datetime.utcnow())
2.templates/base.html
{% block scripts %}
{{ super() }}
{{ moment.include_moment() }}
{% endblock %}
3.templates/index.html
<p>The local date and time is {{ moment(current_time).format('LLL') }}.</p>
<p>That was {{ moment(current_time).fromNow(refresh=True) }}.</p>