//对象初始化列表 对类内调用类(构造函数有参) 在后面直接赋值
#include <iostream>
#include <windows.h>
#pragma warning(disable:4996)
using namespace std;
class Birthday
{
private:
int year;
int month;
int day;
public:
Birthday(int y,int m ,int d);
~Birthday();
void print();
};
Birthday::Birthday(int y, int m, int d)
{
cout << " birthday constuctor" << endl;
year = y;
month = m;
day = d;
}
Birthday::~Birthday()
{
cout << "destuctor birthday " << endl;
}
void Birthday::print()
{
cout << year << "年 " << month << "月 " << day << "日 " << endl;
}
class Student
{
private:
Birthday m_birth;
char name[10];
const int age;
public:
Student(char *n, int y, int m, int d);
//把参数加入我们 后面的 ‘类构造函数里’,最前面 ‘类构造函数’ 使用里面的最后几个值
~Student();
void print();
};
Student::Student(char *n, int y, int m, int d) :m_birth(1000, 12, 3), age(10)
//赋值过去用 “:” + “创建的类对象名” 对于本身的常变量 也这样赋值
{
cout << " student constuctor" << endl;
strcpy(name,n);
}
Student::~Student()
{
cout << " student destuctor" << endl;
}
void Student::print()
{
cout << " 名字:" << name << endl;
cout << " 年龄:" << age << endl;
m_birth.print();
}
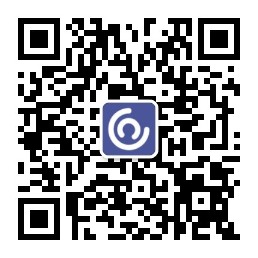
int main()
{
Student s1("aaa",1000,12,3);//初始化列表就是 把调用的 另一个类的参数 放在我们创建的类参数里
s1.print();
system("Pause");
return 0;
}
/***********************************************************************************************************************************************/
/*
//手动调用构造函数 匿名对象 在这行代码结束 就析构了
//但是匿名对象创建了可以复制给新对象 并不等价于 拷贝构造函数
#include <iostream>
#include <windows.h>
using namespace std;
class Test
{
private:
int m_a;
int m_b;
public:
void setab(int _a, int _b);
Test(int a,int b);
~Test();
};
void Test::setab(int _a, int _b)
{
m_a = _a;
m_b = _b;
}
Test::Test(int a,int b)
{
cout << "test constuctor " << endl;
}
Test::~Test()
{
cout << "~test destuct " << endl;
}
int main()
{
Test t1(1,2);
Test(1,2);
Test t3 = Test(2,3);//第一个和第三个是等价的
system("pause");
return 0;
}
*/
/***********************************************************************************************************************************************/
#include <iostream>
#include <windows.h>
using namespace std;
class Test
{
private:
int m_a;
int m_b;
public:
void setab(int _a, int _b);
Test(int a, int b);
Test(int a);
~Test();
};
void Test::setab(int _a, int _b)
{
m_a = _a;
m_b = _b;
}
Test::Test(int a, int b)
{
cout << "test constuctor2 " << endl;
}
Test::Test(int a)
{
cout << "test constuctor1 " << endl;
Test(1,2);//单个参数的构造函数 里创建匿名对象 不会改变此对象中 赋值过的变量值
//因为 这个匿名对象 在遇到自己的分号的时候就结束了
}
Test::~Test()
{
cout << "~test destuct " << endl;
}
int main()
{
Test t1(1, 2);
Test(1, 2);
Test t3 = Test(2, 3);//第一个和第三个是等价的
Test t4(1);
system("pause");
return 0;
}
/***********************************************************************************************************************************************/
//有关 new delete
#include <iostream>
#include <windows.h>
using namespace std;
int main()
{
//以前用的malloc
/*
int *p1 = (int *)malloc( sizeof( int ) );
*p1 = 1;
free( p1 );
int *p2 = (int *)malloc(sizeof(int) * 10);
for (int i = 0; i < 10; i++)
{
*(p2 + i) = i;
}
free(p2);
*/
int *p3 = new int(10);//int *p3 = new int(100);
cout << "p3 = " << *p3 << endl;
delete p3;
int *p4 = new int[10];
cout << "p4 = " << endl;
for (int i = 0; i < 10; i++)
{
cout << << endl;
}
delete p4;
system("pause");
return 0;
}
/***********************************************************************************************************************************************/
//堆上申请 new/malloc
//调用delete 自动调用析构函数
#include <iostream>
#include <windows.h>
using namespace std;
class Test
{
private:
int m_a;
public:
void print();
Test();
Test(int x);
~Test();
};
Test::Test()
{
cout << "无参构造函数" << endl;
}
Test::Test(int x)
{
m_a = x;
cout << "有参构造函数" << endl;
}
Test::~Test()
{
cout << "~析构函数" << endl;
}
void Test::print()
{
cout << "a = " << m_a << endl;
}
int main()
{
Test *t1 = (Test *)malloc(sizeof(Test));
t1->print();
free(t1);//用 malloc 不会调用构造函数的 只是分配空间 最后需要释放空间
Test *t2 = new Test(1);
t2->print();
delete t2;//new 与delete 对应
system("pause");
return 0;
}
//7-24-下午-自己实现tsl
/**********************************************************************************************************************************************/
main.cpp
#include <iostream>
#include <windows.h>
#include "mylist.h"
using namespace std;
int main()
{
Mylist mylist;
Student *s1 = new Student("aaa", 20 ,'m');
Student *s2 = new Student("bbb", 20, 'm');
Student *s3 = new Student("ccc", 20, 'm');
Student *s4 = new Student("ddd", 20, 'm');
Student *s5 = new Student("eee", 20, 'm');
Student *s6 = new Student("fff", 20, 'm');
mylist.push_back(s1);
mylist.push_back(s2);
mylist.push_back(s3);
mylist.push_back(s4);
mylist.push_back(s5);
mylist.push_back(s6);
mylist.list_traval();
mylist.list_delete(s2);
mylist.list_traval();
system("pause");
return 0;
}
mylist.cpp
#include <iostream>
#include "mylist.h"
#include <windows.h>
using namespace std;
Mylist::Mylist()
{
head = new Student;
}
Mylist::~Mylist()
{
cout << "destuct" << endl;
}
void Mylist::push_back(Student *s)
{
Student *s1 = head;
while (s1->getnext() != NULL)
{
s1 = s1->getnext();
}
s1->setnext(s);
}
void Mylist::list_delete(Student *s)
{
Student *s2 = head->getnext();
while (s2 != NULL)
{
if (s2->getnext() == s)
{
Student *s3 = s2;
s2 = s2->next;
s3->setnext( s2->getnext() );
break;
}
else
s2 = s2->getnext();
}
}
void Mylist::list_traval()
{
Student *s2 = head->getnext();
while (s2 != NULL)
{
s2->printall();
s2 = s2->getnext();
}
cout << endl;
}
student.cpp
#include <string.h>
#include "student.h"
#include <iostream>
#pragma warning(disable:4996)
using namespace std;
Student::Student(char *name, int age, char sex)
{
strcpy(m_name, name);
m_age = age;
m_sex = sex;
next = NULL;
}
int Student::getage()
{
return m_age;
}
char* Student::getname()
{
char *name1;
strcpy(name1, m_name);
return name1;
}
char Student::getsex()
{
return m_sex;
}
void Student::printall()
{
cout << "name = " << m_name << " age = " << m_age << " sex = " << m_sex << endl;
}
void Student::setnext(Student *s)
{
next = s;
}
Student * Student::getnext()
{
return next;
}
mylist.h
#ifndef _MYLIST_H_
#define _MYLIST_H_
#include "student.h"
class Mylist
{
private:
Student *head;
public:
Mylist();
~Mylist();
void push_back(Student *s);
void list_delete(Student *s);
void list_traval();
};
#endif
student.h
#ifndef _STUDENT_H_
#define _STUDENT_H_
class Student
{
private:
int m_age;
char m_name[10];
char m_sex;
Student *next;
public:
Student(char *name = "xxx", int age = 20, char sex = 'm');
~Student();
void setnext(Student *s);
Student * getnext();
void printall();
int getage();
char* getname();
char getsex();
};
#endif