数组的增删改查是指对给定数组的特定位置增加一个数据,删除一个数据,改正数据或者查找数据。
本博只提供对数组增删改查的方法。
①增:在给定数组的固定位置插入一个数。
第一种方法:
public static int[] insert1(int[] a) {
System.out.println("请输入要插入的位置:");
Scanner sca=new Scanner(System.in);
int n=sca.nextInt(); //插入位置为n,数组插入位置下标为n-1
System.out.println("请输入要插入的数字:");
Scanner sc=new Scanner(System.in);
int c=sc.nextInt();
//定义一个长度比数组a长度多1的数组b
int[] b=new int[a.length+1];
//将数组a下标为n-1之前的数值赋给数组b
for(int i=0;i<n-1;i++) {
b[i]=a[i];
}
//下标为n-1处数组b的数值为输入数值c
b[n-1]=c;
//为数组b下标为n-1之后的空间赋值
for(int i=n;i<b.length;i++) {
b[i]=a[i-1];
}
return b;
}
第二种方法
public static int[] insert2(int[] a) {
System.out.println("请输入要插入的位置:");
Scanner sca=new Scanner(System.in);
int n=sca.nextInt(); //插入位置为n,数组插入位置下标为n-1
System.out.println("请输入要插入的数字:");
Scanner sc=new Scanner(System.in);
int c=sc.nextInt();//c接收输入的数字
//定义一个长度比数组a长度多1的数组b
int[] b=new int[a.length+1];
//将数组a的值赋给数组b
for(int i=0;i<a.length;i++) {
b[i]=a[i];
}
//将数组b下标为n-1处的数值向后移动一个位置
for(int j=b.length-1;j>n-1;j--) {
b[j]=b[j-1];
}
//将数值c赋给数组b下标为n-1的空间
b[n-1]=c;
return b;
}
②删:删除给定数组特定位置的数据
第一种方法
public static int[] delete1(int[] a) {
System.out.println("请输入要删除的位置:");
Scanner sca=new Scanner(System.in);
int n=sca.nextInt(); //位置为n,下标为n-1
//定义一个数组b接收删除数据后的数组数值
int[] b=new int[a.length-1];
//将数组a下标为n-1之前的数值赋给数组b
for(int i=0;i<n-1;i++){
b[i]=a[i];
}
//将数组a下标为n-1之后的数值赋给数组b
for(int i=n-1;i<b.length;i++) {
b[i]=a[i+1];
}
return b;
}
第二种方法
public static int[] delete2(int[] a) {
System.out.println("请输入要删除的位置:");
Scanner sca=new Scanner(System.in);
int n=sca.nextInt(); //位置为n,下标为n-1
//定义一个长度比数组a小1的数组b接收删除数据后的数组数值
int[] b=new int[a.length-1];
//将数组a的值赋给数组b
for(int i=0;i<n-1;i++){
b[i]=a[i];
}
//将数组b下标为n-1处的数值向前移动一个位置
for(int i=b.length;i>n-1;i--) {
b[i-1]=a[i];
}
return b;
}
③改:修改给定数组特定位置的数据
public static int[] change(int[] a) {
System.out.println("请输入要修改的位置:");
Scanner sca=new Scanner(System.in);
int n=sca.nextInt(); //位置为n,下标为n-1
System.out.println("第"+n+"个数字为"+a[n-1]);
System.out.println("请输入修改后的数字:");
Scanner sc=new Scanner(System.in);
a[n-1]=sc.nextInt();
return a;
}
④查:查找给定数组特定位置的数据或者查找给定数字是否在给定数组中
public static void search(int[] a) {
System.out.println("请输入要查找的位置:");
Scanner sca=new Scanner(System.in);
int n=sca.nextInt();//查找位置为n,下标为n-1
System.out.println("该位置的数字为:"+a[n-1]);
System.out.println("请输入要查找的数字:");
Scanner sc=new Scanner(System.in);
int m=sc.nextInt();//查找数值为m
//遍历数组,判断查找数值是否在给定数组中
for(int i=0;i<a.length;i++) {
//若数组中有数值等于要查找的数值,则输出该数值位置,终止程序
if(a[i]==m){
System.out.println("查找的数字为第"+(i+1)+"个");
return;
}
}
System.out.println("查找的数字不存在该数组中");
}
好的,数组的增删改查就到这里了。
扫描二维码关注公众号,回复:
2410031 查看本文章
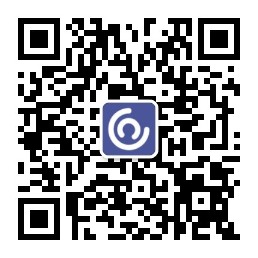
如有疑问,敬请提出。