常见问题:
A.在用jmail发送邮件的时候很多时候会在 Transport.send(message);这里报授权失败的异常:
解决方案:
(1)检查自己用户名或者密码输入错误
(2)是否有配置如下代码
Properties properties = new Properties();
properties.put("mail.smtp.host", serviceAddress);
properties.put("mail.smtp.auth", "true");
(3)对应邮件的STMP服务是否开启,申请的Email默认是关闭的
(4)QQ、163作为发送端还是授权失败,这时候就申请一个另外的Email(例如新浪的Email)
B.用Email开始的时候能发送,在自己测试一段时间后,就不能发送了。
解决方案:
(1)检查是否是代码被自己不小心动了
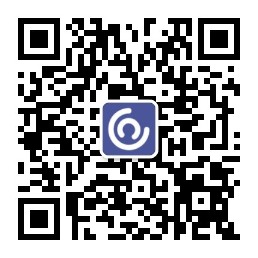
(2)用电脑或者手机端登录下发送邮件试一下,看能否发送;偶尔是因为邮件因为发送过于频繁,而被认为是中毒导致到账没禁止;
C.Jmail要实现给多用户发送邮件
解决方案:
MimeMessage message = new MimeMessage(mailSession);
//设置发送的人数
InternetAddress[] internetAddresss = new InternetAddress[sendAddress.length];
for (int i = 0; i < sendAddress.length; i++) {
internetAddresss[i] = new InternetAddress(sendAddress[i]);
}
message.setRecipients(Message.RecipientType.TO, internetAddresss);
需要的jar包:activation.jar additionnal,jar mail.jar
发送邮件的工具类:
import android.text.TextUtils;
import android.util.Log;
import java.util.Properties;
import javax.activation.DataHandler;
import javax.activation.DataSource;
import javax.activation.FileDataSource;
import javax.mail.Authenticator;
import javax.mail.BodyPart;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
/**
* Created by Relin on 2016/9/6.
* Mail工具类
*/
public class EmailTool extends Authenticator {
//登录的邮件的用户名[xxx.sina.com]
private String userName;
//登录的邮件的密码
private String password;
//发送邮件的服务器
private String serviceAddress;
//邮件主题
private String emailTheme;
//邮件文字内容
private String emailTextContent;
//添加邮件内容的类
private MimeMultipart mimeMultipart;
/**
* @param serviceAddress
* @param userName
* @param password
*/
public EmailTool(String serviceAddress, String userName, String password) {
//初始化配置传递数据的类
mimeMultipart = new MimeMultipart();
this.userName = userName;
this.password = password;
this.serviceAddress = serviceAddress;
}
/**
* 发送邮件之前的授权处理[extends Authenticator]
*
* @return 授权结果
*/
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(userName, password);
}
/**
* 发送Email
*
* @param sendAddress 发送的地址[多地址发送]
* @param emailTheme 发送邮件的主题
* @param emailTextContent 发送邮件的内容
*/
public void sendEmail(final String[] sendAddress, final String emailTheme, final String emailTextContent) {
if (!TextUtils.isEmpty(userName) && !TextUtils.isEmpty(password)
&& !TextUtils.isEmpty(serviceAddress) && !TextUtils.isEmpty(emailTheme)
&& !TextUtils.isEmpty(emailTextContent)) {
new Thread() {//存在网络访问,开线程发送[注意要声明网络权限]
@Override
public void run() {
super.run();
try {
//发送邮件的属性配置
Properties properties = new Properties();
properties.put("mail.smtp.host", serviceAddress);
properties.put("mail.smtp.auth", "true");
properties.put("username", userName);
properties.put("password", password);
//建立发送邮件会话
Session mailSession = Session.getInstance(properties, EmailTool.this);
mailSession.setDebug(true);
MimeMessage message = new MimeMessage(mailSession);
message.setFrom(new InternetAddress(userName));
//设置发送的人数
InternetAddress[] internetAddresss = new InternetAddress[sendAddress.length];
for (int i = 0; i < sendAddress.length; i++) {
internetAddresss[i] = new InternetAddress(sendAddress[i]);
}
message.setRecipients(Message.RecipientType.TO, internetAddresss);
//=====添加发送数据=====
message.setSubject(emailTheme);//邮件主题
BodyPart textBodyPart = new MimeBodyPart();
textBodyPart.setText(emailTextContent);//邮件文字内容
//设置总共需要发送的内容
mimeMultipart.addBodyPart(textBodyPart);
message.setContent(mimeMultipart);
message.saveChanges();
//发送邮件
Transport.send(message);
} catch (MessagingException e) {
e.printStackTrace();
}
}
}.start();
} else {
Log.e("ElveTrees", this.getClass().getSimpleName() + " Parameters of the lack of send Email !");
}
}
/**
* 设置邮件的附件
*
* @param filePath
* @param fileName
*/
public void addEmailAttachment(String filePath, String fileName) {
try {
BodyPart bodyPart = new MimeBodyPart();
DataSource source = new FileDataSource(filePath);
bodyPart.setDataHandler(new DataHandler(source));
bodyPart.setFileName(fileName);
mimeMultipart.addBodyPart(bodyPart);
} catch (MessagingException e) {
e.printStackTrace();
}
}
}