封装:
public class student {
private String name;
private int age;
private char sex;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public char getSex() {
return sex;
}
public void setSex(char sex) {
this.sex = sex;
}
}
继承:
1,写父类:person
public class person {
String name;
char sex;
int age;
String birthday;
public void walk(){
System.out.println("我会走路");
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public char getSex() {
return sex;
}
public void setSex(char sex) {
this.sex = sex;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getBirthday() {
return birthday;
}
public void setBirthday(String birthday) {
this.birthday = birthday;
}
}
2,写子类 student
public class student extends person {
public void study(){
System.out.println("学习");
}
}
3,写子类teacher
public class teacher extends person {
public void teach(){
System.out.println("我会教书");
}
}
4,写main主类
public class test {
public static void main(String[] args) {
student stu1 = new student();
stu1.setName("yangliwei");
System.out.println(stu1.getName());
stu1.walk();
}
}
多态:
允许不同类的对象对同一消息做出响应。即同一操作作用于不同的对象,可以有不同的解释,产生不同的执行结果。这也意味着一个对象有着多重特征,可以在特定的情况下,表现不同的状态,从而对应着不同的属性和方法。
多态的实现方式:接口实现,继承父类进行方法重写,同一个类中进行方法重载。
方法重载(Overloading):一个类中有多个方法(函数),它们具有相同的名字,但方法传递的参数或参数的个数不同,返回类型可以相同。
例子:
扫描二维码关注公众号,回复:
2395247 查看本文章
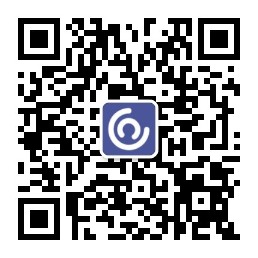
public class Person
{
int children;
public void st(){
System.out.println("123");
}
public Person()//构造函数,这个会在new的时候执行
{
this.getChildren(1);// 赋值children为1
this.getChildren();// 返回true
System.out.println(this.getChildren());
}
public Person(int a){
this.getChildren(a);
}
public void getChildren(int children)
{
this.children = children;
}
public boolean getChildren()
{
if (children != 0)
return true;
else
return false;
}
}
方法重写(Override): 子类对父类的方法做一定的修改,返回值和形参都不能改变。又称方法覆盖。