java用org.apache.poi包操作excel
一.POI简介
Jakarta POI 是apache的子项目,目标是处理ole2对象。它提供了一组操纵Windows文档的Java API
目前比较成熟的是HSSF接口,处理MS Excel(97-2002)对象。它不象我们仅仅是用csv生成的没有格式的可以由Excel转换的东西,而是真正的Excel对象,你可以控制一些属性如sheet,cell等等。
二.HSSF概况
HSSF 是Horrible SpreadSheet Format的缩写,也即“讨厌的电子表格格式”。也许HSSF的名字有点滑稽,就本质而言它是一个非常严肃、正规的API。通过HSSF,你可以用纯Java代码来读取、写入、修改Excel文件。
HSSF 为读取操作提供了两类API:usermodel和eventusermodel,即“用户模型”和“事件-用户模型”。前者很好理解,后者比较抽象,但操作效率要高得多。
三.开始编码
1 . 准备工作
要求:JDK 1.4+POI开发包
2 . EXCEL 结构
HSSFWorkbook excell 文档对象介绍
HSSFSheet excell的表单
HSSFRow excell的行
HSSFCell excell的格子单元
HSSFFont excell字体
HSSFName 名称
HSSFDataFormat 日期格式
3 .具体用法实例 (采用 usermodel )
如何读Excel
读取Excel文件时,首先生成一个POIFSFileSystem对象,由POIFSFileSystem对象构造一个HSSFWorkbook,该HSSFWorkbook对象就代表了Excel文档。下面代码读取上面生成的Excel文件写入的消息字串:
try{
POIFSFileSystem fs=new POIFSFileSystem(new FileInputStream("d:/workbook.xls"));
HSSFWorkbook wb = new HSSFWorkbook(fs);
HSSFSheet sheet = wb.getSheetAt(0);
HSSFRow row = sheet.getRow(0);
HSSFCell cell = row.getCell((short) 0);
String msg = cell.getStringCellValue();
System.out.println(msg);
}catch(Exception e){
e.printStackTrace();
}
如何写excel,
将excel的第一个表单第一行的第一个单元格的值写成“a test”。
POIFSFileSystem fs =new POIFSFileSystem(new FileInputStream("workbook.xls"));
HSSFWorkbook wb = new HSSFWorkbook(fs);
HSSFSheet sheet = wb.getSheetAt(0);
HSSFRow row = sheet.getRow(0);
HSSFCell cell = row.getCell((short)0);
cell.setCellValue("a test");
// Write the output to a file
FileOutputStream fileOut = new FileOutputStream("workbook.xls");
wb.write(fileOut);
fileOut.close();
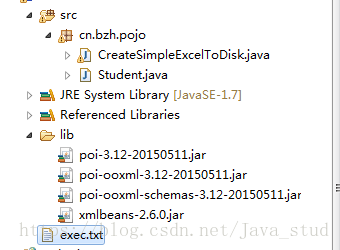
package cn.bzh.pojo;
import java.io.FileOutputStream;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.List;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFCellStyle;
import org.apache.poi.hssf.usermodel.HSSFRow;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
public class CreateSimpleExcelToDisk {
//手工构建一个简单格式的Excel
private static List<Student> getStudent() throws Exception
{
List list = new ArrayList();
SimpleDateFormat df = new SimpleDateFormat("yyyy-mm-dd");
Student user1 = new Student(1, "张三", 16, df.parse("1997-03-12"));
Student user2 = new Student(2, "李四", 17, df.parse("1996-08-12"));
Student user3 = new Student(3, "王五", 26, df.parse("1985-11-12"));
list.add(user1);
list.add(user2);
list.add(user3);
return list;
}
public static void main(String[] args) throws Exception {
// 第一步,创建一个webbook,对应一个Excel文件
HSSFWorkbook wb = new HSSFWorkbook();
// 第二步,在webbook中添加一个sheet,对应Excel文件中的sheet
HSSFSheet sheet = wb.createSheet("学生表一");
// 第三步,在sheet中添加表头第0行,注意老版本poi对Excel的行数列数有限制short
HSSFRow row = sheet.createRow((int) 0);
// 第四步,创建单元格,并设置值表头 设置表头居中
HSSFCellStyle style = wb.createCellStyle();
style.setAlignment(HSSFCellStyle.ALIGN_CENTER); // 创建一个居中格式
HSSFCell cell = row.createCell((short) 0);
cell.setCellValue("学号");
cell.setCellStyle(style);
cell = row.createCell((short) 1);
cell.setCellValue("姓名");
cell.setCellStyle(style);
cell = row.createCell((short) 2);
cell.setCellValue("年龄");
cell.setCellStyle(style);
cell = row.createCell((short) 3);
cell.setCellValue("生日");
cell.setCellStyle(style);
// 第五步,写入实体数据 实际应用中这些数据从数据库得到,
List list = CreateSimpleExcelToDisk.getStudent();
for (int i = 0; i < list.size(); i++){
row = sheet.createRow((int) i + 1);
Student stu = (Student) list.get(i);
// 第四步,创建单元格,并设置值
row.createCell((short) 0).setCellValue((double) stu.getId());
row.createCell((short) 1).setCellValue(stu.getName());
row.createCell((short) 2).setCellValue((double) stu.getAge());
cell = row.createCell((short) 3);
cell.setCellValue(new SimpleDateFormat("yyyy-mm-dd").format(stu
.getBirth()));
}
// 第六步,将文件存到指定位置
try{
FileOutputStream fout = new FileOutputStream("E:/students.xls");
wb.write(fout);
fout.close();
}catch (Exception e) {
e.printStackTrace();
}
}
}
package cn.bzh.pojo;
import java.util.Date;
public class Student {
private int id;
private String name;
private int age;
private Date birth;
public Student() {
super();
}
public Student(int id, String name, int age, Date birth) {
super();
this.id = id;
this.name = name;
this.age = age;
this.birth = birth;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public Date getBirth() {
return birth;
}
public void setBirth(Date birth) {
this.birth = birth;
}
}