题目描述
Given a string, determine if it is a palindrome, considering only alphanumeric characters and ignoring cases.
For example,
"A man, a plan, a canal: Panama"is a palindrome.
"race a car"is not a palindrome.
Note:
Have you consider that the string might be empty? This is a good question to ask during an interview.
For the purpose of this problem, we define empty string as valid palindrome.
解题思路1
将字符串转为char型数组
再将类型为字母和数字类型的值,压入栈
将char型数组中的类型为字母和数字类型的值与栈顶元素比较,若相等,则判断下一个,若不等,则返回false,其中根据题意,需将所有字母值转化为小写
代码示例1
class Solution {
public:
bool isPalindrome(string s) {
char* p = (char*)s.c_str();
stack<char> q;
for (int i = 0; i < strlen(p); i++) {
if (isalnum(p[i]))
q.push(p[i]);
}
for (int i = 0; i < strlen(p); i++) {
if (isalnum(p[i])) {
if (tolower(q.top()) != tolower(p[i])) return false;
q.pop();
}
}
return true;
}
};
缺点:使用了额外空间栈,且存在两个for循环,看起来就很复杂
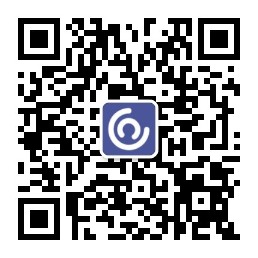
解题思路2
从字符串两头开始判断每一对类型为字母或数字的值是否相等
代码示例2
class Solution {
public:
bool isPalindrome(string s){
for (int i = 0, j = s.length() - 1; i < j;i++, j--) {
while (i < j && !isalnum(s[i])) i++;
while (i < j && !isalnum(s[j])) j--;
if (i < j && tolower(s[i]) != tolower(s[j])) return false;
}
return true;
}
};