C#网编Console(二)
一、TCP
//服务端
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Net.Sockets; //添加Socket类空间
using System.Net;
namespace Server_test
{
class Program
{
private static byte[] result = new Byte[1024];
private static int myport = 8012;
static Socket serverSocket;
static void Main(string[] args)
{
//服务器IP地址
IPAddress ip = IPAddress.Parse("127.0.0.1");
serverSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
serverSocket.Bind(new IPEndPoint(ip, myport));
serverSocket.Listen(10);
Console.WriteLine("启动监听{0}", serverSocket.LocalEndPoint.ToString());
//通过clientsocket发送数据
string sendMessage = "server send Message Hello";
Socket clientsocket = serverSocket.Accept();
clientsocket.Send(Encoding.ASCII.GetBytes(sendMessage));
Console.WriteLine("向客户端发送消息:{0}", sendMessage);
//通过clientsocket接收数据
int receiveNumber = clientsocket.Receive(result);
Console.WriteLine("接收客户端{0}消息{1}", clientsocket.RemoteEndPoint.ToString(), Encoding.ASCII.GetString(result, 0, receiveNumber));
clientsocket.Shutdown(SocketShutdown.Both);
clientsocket.Close();
Console.ReadLine();
}
}
}
//客户端
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Net.Sockets; //添加Socket类空间
using System.Net;
namespace Client_test
{
class Program
{
private static byte[] result = new Byte[1024];
static void Main(string[] args)
{
//服务器IP地址
IPAddress ip = IPAddress.Parse("127.0.0.1");
Socket clientSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
try
{
clientSocket.Connect(new IPEndPoint(ip, 8012));
Console.WriteLine("连接服务器成功");
}
catch
{
Console.WriteLine("连接服务失败,请按回车键退出");
return;
}
//通过clientSocket接收数据
int receiveLength = clientSocket.Receive(result);
Console.WriteLine("接收服务器消息:{0}", Encoding.ASCII.GetString(result, 0, receiveLength));
//通过clientSocket发送数据
string sendMessage = "client send Message Hello";
clientSocket.Send(Encoding.ASCII.GetBytes(sendMessage));
Console.WriteLine("向服务器发送消息:{0}", sendMessage);
clientSocket.Shutdown(SocketShutdown.Both);
clientSocket.Close();
Console.ReadLine();
}
}
}
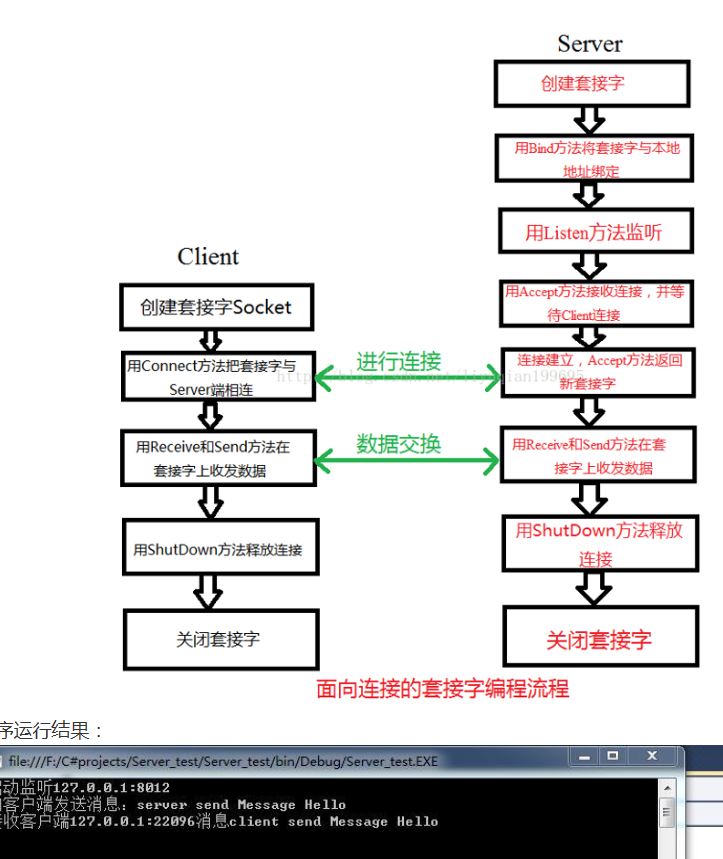
二、UDP
//发送方
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Net; //add net namespace
using System.Net.Sockets;
namespace Send_Test
{
class Program
{
private static int remoteReceivePort = 8012;
static void Main(string[] args)
{
IPAddress ip = IPAddress.Parse("127.0.0.1");
//发送方:发送数据
Socket sendSocket = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp);
sendSocket.SendTo(Encoding.UTF8.GetBytes("测试数据"), new IPEndPoint(ip, remoteReceivePort));
Console.WriteLine("发送测试数据");
sendSocket.Shutdown(SocketShutdown.Send);
sendSocket.Close();
Console.ReadLine();
}
}
}
//接收方
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Net; //add net namespace
using System.Net.Sockets;
namespace Recv_Test
{
class Program
{
private static int receivePort = 8012;
static void Main(string[] args)
{
IPAddress ip = IPAddress.Parse("127.0.0.1");
//接收准备
Socket receiveSocket = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp);
receiveSocket.Bind(new IPEndPoint(ip, receivePort));
//接收数据
byte[] result = new Byte[1024];
EndPoint senderRemote = (EndPoint)(new IPEndPoint(IPAddress.Any, 0));
//引用类型参数为EndPoint类型,用于存放发送方的IP地址和端点
int length = receiveSocket.ReceiveFrom(result, ref senderRemote);
Console.WriteLine("接收到{0}消息:{1}", senderRemote.ToString(), Encoding.UTF8.GetString(result, 0, length).Trim());
receiveSocket.Shutdown(SocketShutdown.Receive);
Console.ReadLine();
}
}
}
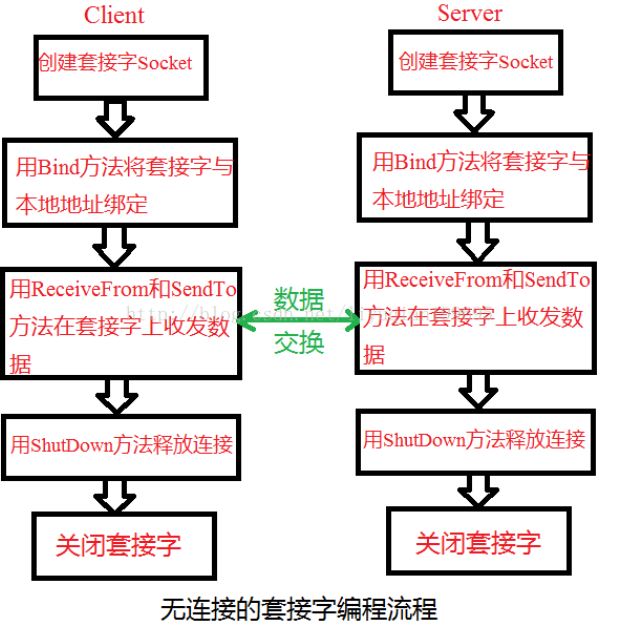
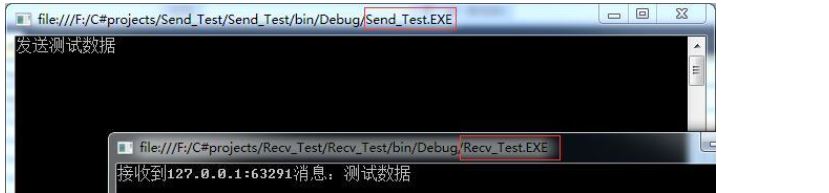