3.3 Putting Strings Together with StringBuilder
StringBuilderDemo.java
package String;
/**shows three ways of concatenating strings*/
public class StringBuilderDemo {
public static void main(String[] args) {
// TODO Auto-generated method stub
String s1 = "Hello"+", "+"World";
System.out.println(s1);
//Build a StringBuilder,and append some things to it.
StringBuilder sb2 = new StringBuilder();
sb2.append("Hello");
sb2.append(',');
sb2.append(' ');
sb2.append("World");
//Get the StringBuilder's value as s String, and print it.
String s2 = sb2.toString();
System.out.println(s2);
//Now do the above all over again, but in a more
//concise (and typical "real-world" Java) fashion.
System.out.println(
new StringBuilder()
.append("Hello")
.append(',')
.append(' ')
.append("World")
);
}
}
result
StringBuilderDemo.java
...convert a list of items into a comma-separated list, while avoiding getting an extra comma after the last element of the list.
package String;
import java.awt.image.SampleModel;
import java.util.StringTokenizer;
public class StringBuilderCommaList {
public static void main(String[] args) {
// TODO Auto-generated method stub
//法1:StringBuilder.length()
String SAMPLE_STRING = new String("Hello World!");
StringBuilder sb1 = new StringBuilder();
for(String word : SAMPLE_STRING.split(" ")) {
if (sb1.length()>0) {
sb1.append(", ");
}
sb1.append(word);
}
System.out.println(sb1);
//Method using a StringTokenizer
//法2:hasMoreElements()
StringTokenizer st = new StringTokenizer(SAMPLE_STRING);
StringBuilder sb2 = new StringBuilder();
while(st.hasMoreElements()) {
sb2.append(st.nextToken());
if (st.hasMoreElements()) {
sb2.append(", ");
}
}
System.out.println(sb2);
}
}
result
3.4 Processing a String One Character at a Time
StrCharAt.java
...process the contents of a string, one character at a time.
package String;
public class StrCharAt {
public static void main(String[] args) {
// TODO Auto-generated method stub
String a = "A quick bronze fox lept a lazy bovine";
for (int i = 0; i < a.length(); i++) { //Don't use foreach
System.out.println("Char" + i + " is " + a.charAt(i));
}
}
}
result
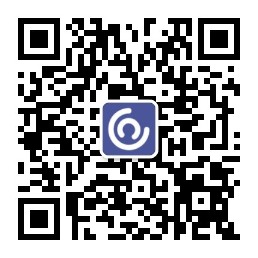
ForEachChar.java
package String;
public class ForEachChar {
public static void main(String[] args) {
// TODO Auto-generated method stub
String s = "Hello world";
//for (char ch : s){...} Does not work, in Java 7
//foreach里面期望的是一个array,不是一个String类型。String.toCharArray()把String转换成array
for (char ch : s.toCharArray()) {
System.out.println(ch);
}
}
}
result
CheckSum.java
A "checksum" is a numeric quantity representing and confirming the contents of a file.
...
below it's (斜体非书中内容,为衔接而加的) the simplest possible checksum, computed just by adding the numberic values of each character. Note that on files, it does not include the values of the newline characters; in order to fix this, retrieve(检索) System.getProperty("line.separator"); and add its character value(s) into the sum at the end of each line. Or give up line mode and read the file a character at a time.
package String;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStreamReader;
import javax.management.RuntimeErrorException;
/**CheckSum one text file, given an open BufferedReader.
* CheckSum does not include line endings, so will give the
* same value for given text on any platform.Do not use
* on binary files!
*/
public class CheckSum {
public static void main(String[] args) throws FileNotFoundException {
BufferedReader is = new BufferedReader(new FileReader(new File("E:\\javaString.txt")));
System.out.println(process(is));
}
public static int process(BufferedReader is) {
int sum = 0;
try {
String inputLine;
while ((inputLine = is.readLine()) != null ) {
int i;
for( i=0; i<inputLine.length(); i++) {
sum += inputLine.charAt(i);
}
}
} catch (IOException e) {
// TODO: handle exception
throw new RuntimeException("IOException: " + e);
}
return sum;
}
}
result