前言:由于之前是做的支付相关的工作,因此对于密码支付的安全比较重视,在工作中经常要接触到随机密码键盘的需求。加上明天就要放假(公司给了一周的假期,很开心~)了,今天没什么工作,就编写一个随机键盘正好回一下曾经的工作吧。
首先,先上图片(界面较粗糙,不喜勿喷):
从上面的图片中,我们大致可以看出功能很简单就是一个数字密码键盘,支持删除,接下来一起来实现:
Step1,界面设计
整个界面是一个竖立的线性布局,由三部分组成,头部标题、密码TextView以及数字键盘,实现代码如下:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:layout_weight="1"
android:background="#000066"
>
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:textColor="#ffffff"
android:textSize="24sp"
android:text="密码键盘"
/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:gravity="center"
android:layout_weight="4"
>
<TextView
android:id="@+id/tv_pwd"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:textSize="36sp"
android:password="true"
android:maxLength="6"
android:text=""
/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:layout_weight="6"
>
<LinearLayout
android:id="@+id/table_num"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="6"
android:background="#c8cbcc"
android:orientation="vertical">
<LinearLayout style="@style/layout_input_amount_style">
<Button
android:id="@+id/btn_1"
style="@style/btn_input_amount_style"
android:layout_marginRight="1dp"
android:text="1" />
<Button
android:id="@+id/btn_2"
style="@style/btn_input_amount_style"
android:layout_marginRight="1dp"
android:text="2" />
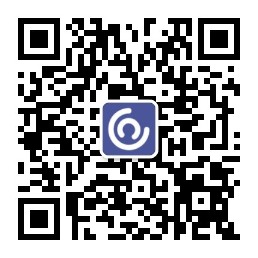
<Button
android:id="@+id/btn_3"
style="@style/btn_input_amount_style"
android:text="3" />
</LinearLayout>
<LinearLayout style="@style/layout_input_amount_style">
<Button
android:id="@+id/btn_4"
style="@style/btn_input_amount_style"
android:layout_marginRight="1dp"
android:text="4" />
<Button
android:id="@+id/btn_5"
style="@style/btn_input_amount_style"
android:layout_marginRight="1dp"
android:text="5" />
<Button
android:id="@+id/btn_6"
style="@style/btn_input_amount_style"
android:text="6" />
</LinearLayout>
<LinearLayout style="@style/layout_input_amount_style">
<Button
android:id="@+id/btn_7"
style="@style/btn_input_amount_style"
android:layout_marginRight="1dp"
android:text="7" />
<Button
android:id="@+id/btn_8"
style="@style/btn_input_amount_style"
android:layout_marginRight="1dp"
android:text="8" />
<Button
android:id="@+id/btn_9"
style="@style/btn_input_amount_style"
android:text="9" />
</LinearLayout>
<LinearLayout style="@style/layout_input_amount_style">
<Button
android:id="@+id/btn_e"
style="@style/btn_input_amount_style"
android:layout_marginRight="1dp"
android:background="#00ff00"
android:text="确认" />
<Button
android:id="@+id/btn_0"
style="@style/btn_input_amount_style"
android:layout_marginRight="1dp"
android:text="0" />
<ImageButton
android:id="@+id/btn_d"
style="@style/btn_input_amount_style"
android:src="@drawable/key_back" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
</LinearLayout>
Step2,生成10个互不相同的0-9的数字
设计思路是利用for循环中嵌套while循环生成0-9组成的字符串,代码如下:
//产生n个互不相同的数(0~9)
private String getRandom(int n) {
// TODO Auto-generated method stub
int x = 10;
String result="";
Random rand = new Random();//新建一个随机类
boolean[] bool = new boolean[x];
int randInt = 0;
for(int i = 0; i < n ; i++) {
do {
randInt = rand.nextInt(x);//产生一个随机数
}while(bool[randInt]);
bool[randInt] = true;
result+=randInt;
}
return result;
}
Step3,初始化密码键盘并添加点击事件
private void initData() {
// TODO Auto-generated method stub
String ran=getRandom(10);
ids=new int[]{R.id.btn_0,R.id.btn_1,R.id.btn_2,R.id.btn_3,R.id.btn_4,R.id.btn_5,R.id.btn_6,R.id.btn_7,R.id.btn_8,R.id.btn_9};
pwd="";
for(int i=0;i<ran.length();i++){
((Button)findViewById(ids[i])).setText(ran.charAt(i)+"");
((Button)findViewById(ids[i])).setOnClickListener(new OnClickListener() {
@Override
public void onClick(View view) {
// TODO Auto-generated method stub
Button btn = (Button)view;
if(tv_pwd.getText().toString().length()<6){
tv_pwd.setText(tv_pwd.getText().toString()+btn.getText());
}else{
Toast.makeText(getBaseContext(), "密码长度最长为6", Toast.LENGTH_SHORT).show();
}
}
});
}
}
Step4,处理确认与删除事件
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
switch (arg0.getId()) {
case R.id.btn_e:
if(tv_pwd.getText().equals("211314")){
Toast.makeText(getBaseContext(), "验证成功", Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(getBaseContext(), "验证失败", Toast.LENGTH_SHORT).show();
}
break;
case R.id.btn_d:
if(tv_pwd.getText().length()>0){
String str = tv_pwd.getText().toString();
tv_pwd.setText(str.substring(0, str.length()-1));
}
break;
default:
break;
}
}
Step5,运行~