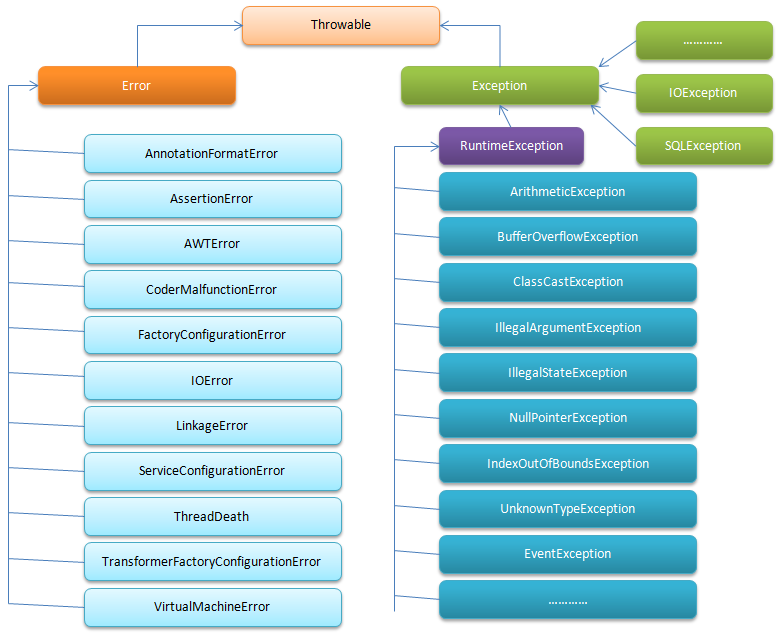
注意事项:
1。 Error仅在java的虚拟机中发生,用户无需在程序中捕捉或者抛出Error。
2。 Exception分为一般的Exception和RuntimeException两类。这里有点让人觉得别扭的是RuntimeException(Unchecked)继承于Exception(Checked)的父类。
PS: checked与unchecked的概念理解:
checked: 一般是指程序不能直接控制的外界情况,是指在编译的时候就需要检查的一类exception,用户程序中必须采用try catch机制处理或者通过throws交由调用者来处理。这类异常,主要指除了Error以及RuntimeException及其子类之外的异常。
unchecked:是指那些不需要在编译的时候就要处理的一类异常。在java体系里,所有的Error以及RuntimeException及其子类都是unchecked异常。再形象直白的理解为不需要try catch等机制处理的异常,可以认为是unchecked的异常。
checked与unchecked在throwable的继承关系中体现为下图:
+-----------+ | Throwable | +-----------+ / \ / \ +-------+ +-----------+ | Error | | Exception | +-------+ +-----------+ / | \ / | \ \ \________/ \______/ \ +------------------+ unchecked checked | RuntimeException | +------------------+ / | | \ \_________________/ unchecked
首先定义一个基本的异常类GenericException,继承于Exception。
package check_unchecked_exceptions; public class GenericException extends Exception{ /** * */ private static final long serialVersionUID = 2778045265121433720L; public GenericException(){ } public GenericException(String msg){ super(msg); } }
下面定义一个测试类VerifyException
package check_unchecked_exceptions; public class VerifyException { public void first() throws GenericException { throw new GenericException("checked exception"); } public void second(String msg){ if(msg == null){ throw new NullPointerException("unchecked exception"); } } public void third() throws GenericException{ first(); } public static void main(String[] args) { VerifyException ve = new VerifyException(); try { ve.first(); } catch (GenericException e) { e.printStackTrace(); } ve.second(null); } }
上面的例子,结合checked以及unchecked的概念,可以看出Exception这个父类是checked类型,但是其子类RuntimeException (子类NullPointerException)却是unchecked的。
https://www.cnblogs.com/shihuc/p/5201905.html