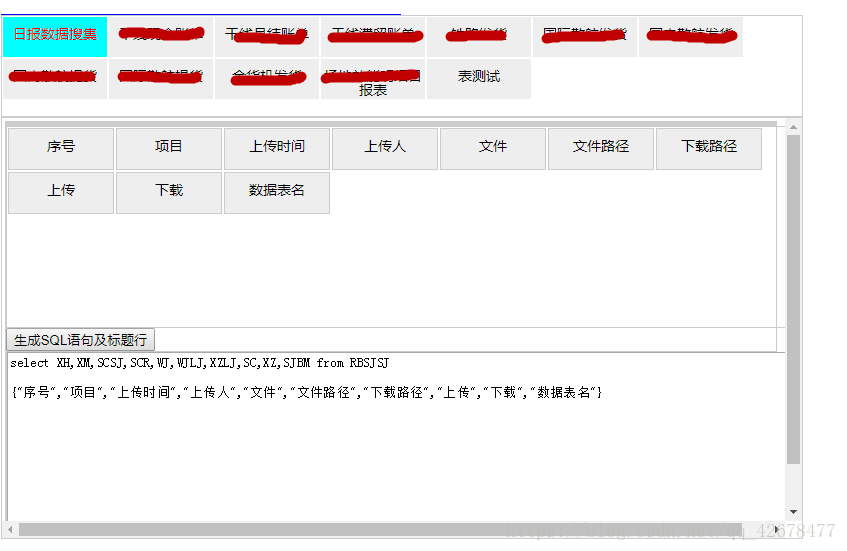
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="GenSqlByMdb.aspx.cs" Inherits="Rjkbb_Other_GenSqlByMdb" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>生成SQL语句工具</title>
<style>
.element {
width: 100px;
height: 30px;
float: left;
margin: 1px;
background-color: #eee;
text-align: center;
font-size: 14px;
padding: 10px 2px 0;
}
.highlight {
color: red;
/*font-weight:bold;*/
background-color: aqua;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div>
<div>
<asp:TextBox ID="txtFilter" runat="server" Style="width: 400px; border: none; border-bottom: 1px solid blue;"></asp:TextBox>
</div>
<div id="divTables" runat="server" style="width: 800px; height: 100px; border: 1px solid #ccc;">
</div>
<iframe id="iFields" style="border: 1px solid #ccc; width: 800px; height: 420px;"></iframe>
<div style="visibility: hidden">
<asp:TextBox runat="server" ID="txtResult" TextMode="MultiLine" Height="200px" Width="800px" />
</div>
</div>
</form>
</body>
<script>
$(function () {
$("#divTables").find("div").click(function () {
$(this).addClass("highlight").siblings().removeClass('highlight');
var tableName = $(this).attr('propname');
var url = _akUrl("GetSqlFieldsByMdb.aspx?ele=" + tableName);
//alert(url);
$("#iFields").attr("src", url);
});
});
</script>
</html>
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.HtmlControls;
using Appkit.Common;
using Appkit.Web.Management;
using Appkit.Web.UI;
using CpmBigDataImportService;
using System.Data;
public partial class Rjkbb_Other_GenSqlByMdb : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
AkPageService.SetCommonStyle(this);
Script.IncludeCommon(this);
if (!IsPostBack)
{
string appid = AkContext.AppId;
AccessHelper acc = new AccessHelper(appid);
string sql = "select * from md_sys_element";
//List<string> strs = new List<string>();
DataTable dt = acc.ExecuteDataSet(acc.ConnectionString, sql).Tables[0];
for (int i = 0; i < dt.Rows.Count; i++)
{
HtmlGenericControl div;
div = new HtmlGenericControl("div");
div.InnerText = dt.Rows[i]["ELE_DISPNAME"].ToString();
div.Attributes["class"] = "element";
div.Attributes["propName"] = dt.Rows[i]["ELE_NAME"].ToString();
divTables.Controls.Add(div);
//ddlTables.Items.Add(dt.Rows[i]["ELE_DISPNAME"].ToString());
//strs.Add(string.Format("<div propname=\"{1}\">{0}</div>]"
// , dt.Rows[i]["ELE_DISPNAME"].ToString()
// , dt.Rows[i]["ELE_NAME"].ToString()));
}
}
//txtResult.Text = string.Join(",", strs.ToArray());
}
}
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="GetSqlFieldsByMdb.aspx.cs" Inherits="Rjkbb_Other_GetSqlFieldsByMdb" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>字段列表</title>
<style>
.element {
width: 100px;
height: 30px;
float: left;
margin: 1px;
background-color: #eee;
text-align: center;
font-size: 14px;
padding: 10px 2px 0;
}
div {
border: 1px solid #ccc;
}
</style>
<script>
function allowDrop(ev) {
ev.preventDefault();
}
var srcdiv = null;
function drag(ev, divdom) {
srcdiv = divdom;
ev.dataTransfer.setData("text/html", divdom.innerHTML);
}
function drop(ev, divdom) {
ev.preventDefault();
if (srcdiv != divdom) {
var propSrc = srcdiv.getAttribute("propName");
var propDest = divdom.getAttribute("propName");
srcdiv.innerHTML = divdom.innerHTML;
srcdiv.setAttribute("propName", propDest);
divdom.innerHTML = ev.dataTransfer.getData("text/html");
divdom.setAttribute("propName", propSrc);
}
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<input type="hidden" id="hdnTableName" runat="server" />
<div id="divMain" runat="server" style="width: 800px; height: 200px"></div>
<input type="button" value="生成SQL语句及标题行"
onclick="getFields();" />
<div>
<asp:TextBox ID="txtResult" TextMode="MultiLine" runat="server" Style="width: 800px; height: 200px"></asp:TextBox>
</div>
</div>
</form>
<script>
function getFields() {
var str = 'select ' + $("#divMain").find("div").map(function () {
return $(this).attr("propName");
}).get().join() + ' from ' + $("#hdnTableName").val();
var strTitle = '{' + $("#divMain").find("div").map(function () {
return '"' + $(this).text() + '"';
}).get().join() + '}';
$("#txtResult").val(str + "\r\n" + "\r\n" + strTitle);
//alert(str);
}
</script>
</body>
<script>
$(function () {
$("#divMain").find("div").dblclick(function () {
//alert("dbclick");
$(this).remove();
});
});
</script>
</html>
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using CpmBigDataImportService;
using System.Web.UI.HtmlControls;
using Appkit.Common;
using Appkit.Web.Management;
using Appkit.Web.UI;
using System.Data;
public partial class Rjkbb_Other_GetSqlFieldsByMdb : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
AkPageService.SetCommonStyle(this);
Script.IncludeCommon(this);
string tableName = AkCommon.GetQueryString("ele");
if (!IsPostBack)
{
string appid = AkContext.AppId;
hdnTableName.Value = tableName;
AccessHelper acc = new AccessHelper(appid);
string sql = string.Format("select prop_name,prop_dispname from md_sys_property where ele_id in ( select ele_id from md_sys_element where ele_name='{0}')", tableName);
DataTable dt = acc.ExecuteDataSet(acc.ConnectionString, sql).Tables[0];
for (int i = 0; i < dt.Rows.Count; i++)
{
HtmlGenericControl div;
div = new HtmlGenericControl("div");
div.InnerText = dt.Rows[i][1].ToString();
div.Attributes["class"] = "element";
div.Attributes["propName"] = dt.Rows[i][0].ToString();
div.Attributes["ondrop"] = "drop(event,this)";
div.Attributes["ondragover"] = "allowDrop(event)";
div.Attributes["draggable"] = "true";
div.Attributes["ondragstart"] = "drag(event, this)";
divMain.Controls.Add(div);
// .Controls.Add(div);
//ddlTables.Items.Add(dt.Rows[i]["ELE_DISPNAME"].ToString());
//strs.Add(string.Format("<div propname=\"{1}\">{0}</div>]"
// , dt.Rows[i]["ELE_DISPNAME"].ToString()
// , dt.Rows[i]["ELE_NAME"].ToString()));
}
}
}
}