angular directive 入门
“控制器应该尽可能保持短小精悍,而在控制器中进行DOM操作和数据操作则是一个不好的实践。”
“设计良好的应用会将复杂的逻辑放到指令和服务中。通过使用指令和服务,我们可以将控制器重构成一个轻量且更易维护的形式”
《AngularJs权威教程》
Hello Directive
<body ng-app="myApp">
<my-student></my-student>
<script type="text/javascript">
var app = angular.module('myApp',[]);
app.directive("myStudent",function(){
return {
restrict:"EA",
template:"Hello Directive"
}
})
</script>
</body>
是的,创建一个指令就是这么简单,但是仅仅如此可是不行的,比如:如何让指令的html更丰富呢,如何让指令也能进行数据绑定呢,如何向指令中传递参数、方法呢,是否可以指令嵌套指令呢…请继续往后看
Directive结构分析
restrict:属性名称,取”EACM”中的任意一个或多个字母,它是用来限制指令的声明格式
E element 元素名称
<my-student></my-student>
A attribute 属性
<div my-student></div>
C class 类名
<div class="my-student"></div>
M 注释
<!-- directive:my-student -->
template:’指令的内容(html代码)’
template:'<div><p>{{name}}</p></div>'
app.directive("myStudent",function(){
return {
restrict:"EA",
template:"<div><p>{{name}}</p></div>",
controller:function($scope){
$scope.name="CONST_Q"
}
}
})
templateUrl:’指令的内容(引用html文件内容作为指令内容)’
<!-- child1.html-->
<div>
<p>您好 {{name}}</p>
</div>
app.directive("myStudent",function(){
return {
restrict:"EA",
templateUrl:"child1.html",
controller:function($scope){
$scope.name="CONST_Q"
}
}
})
controller:指令的控制器,可以是自己的,也可以继承其他的
<body ng-app="myApp">
<div ng-controller="myCtrl">
<p>Hello {{name}}</p>
</div>
<my-student></my-student>
<script type="text/javascript">
var app = angular.module('myApp',[]);
app.controller("myCtrl",function($scope){
$scope.name = "XiaoMing"
})
app.directive("myStudent",function(){
return {
restrict:"EA",
templateUrl:"child1.html",
controller:function($scope){
$scope.name="小明"
}
}
})
</script>
</body>
或
app.directive("myStudent",function(){
return {
restrict:"EA",
templateUrl:"child1.html",
controller:'myCtrl'
}
})
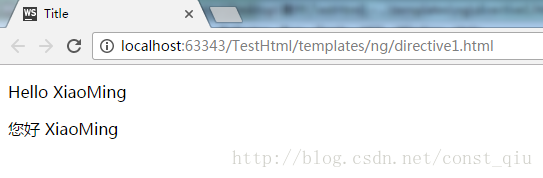
scope:指令的作用域对象,当你写上这个属性时,就表示这个directive不会从他的controller里承$scope对象,而是会重新创建一个。(封闭自定义的scope,希望自己的directive用在多处,一共三种状态形式)
@:外部scope赋值不影响scope的变量
<!-- child1.html-->
<div>
<p>您好 {{name2}}</p>
<button ng-click="updateName2()">修改用户名</button>
</div>
<!--directive1.html-->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script type="text/javascript" src="../../lib/angular-1.4.1/angular.min.js"></script>
</head>
<body ng-app="myApp" ng-controller="myCtrl">
<div style="background-color: burlywood">
<p>Hello {{name1}}</p>
<button ng-click="updateName1()">Update Name</button>
</div>
<div my-student name3="{{name1}}"></div>
<script type="text/javascript">
var app = angular.module('myApp',[]);
app.controller("myCtrl",function($scope){
$scope.name1 = "XiaoMing";
var i=1;
$scope.updateName1 = function(){
$scope.name1 = $scope.name1 + (i++);
}
})
app.directive("myStudent",function(){
return {
restrict:"EA",
templateUrl:"child1.html",
scope:{
name2:'@name3'
},
controller:function($scope){
var i=1;
$scope.updateName2 = function(){
$scope.name2 = $scope.name2 + (i++);
}
}
}
})
</script>
</body>
</html>
=:外部scope赋值会影响封闭scope变量
<div my-student name3="name1"></div>
scope:{
name2:'=name3'
},
&:允许外部scope传递一个函数、方法给封闭scope
传递无参函数(需要注意变量的命名规则和书写规则–骆驼峰)
<!-- child1.html-->
<div>
<p>您好 {{name2}}</p>
<button ng-click="updateName2()">修改用户名</button>
</div>
<!--directive1.html-->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script type="text/javascript" src="../../lib/angular-1.4.1/angular.min.js"></script>
</head>
<body ng-app="myApp" ng-controller="myCtrl">
<div style="background-color: burlywood">
<p>Hello {{name1}}</p>
<button ng-click="updateName1()">Update Name</button>
</div>
<div my-student name3="name1" update-name3="updateName1()"></div>
<script type="text/javascript">
var app = angular.module('myApp',[]);
app.controller("myCtrl",function($scope){
$scope.name1 = "XiaoMing";
var i=1;
$scope.updateName1 = function(){
$scope.name1 = $scope.name1 + (i++);
}
})
app.directive("myStudent",function(){
return {
restrict:"EA",
templateUrl:"child1.html",
scope:{
name2:'=name3',
updateName2:'&updateName3'
}
}
})
</script>
</body>
</html>
传递有参函数
<!-- child1.html-->
<div>
<p>您好 {{name2}}</p>
<button ng-click="updateName2({name4:name2})">修改用户名</button>
</div>
<body ng-app="myApp" ng-controller="myCtrl">
<div style="background-color: burlywood">
<p>Hello {{name1}}</p>
<button ng-click="updateName1({name4:name1})">Update Name</button>
</div>
<div my-student name3="name1" update-name3="updateName1({name4:name1})"></div>
<script type="text/javascript">
var app = angular.module('myApp',[]);
app.controller("myCtrl",function($scope){
$scope.name1 = "XiaoMing";
var i=1;
$scope.updateName1 = function(name){
$scope.name1 = name.name4 + (i++);
console.log(name)
}
})
app.directive("myStudent",function(){
return {
restrict:"EA",
templateUrl:"child1.html",
scope:{
name2:'=name3',
updateName2:'&updateName3'
}
}
})
</script>
</body>
link:可以理解为directive被angular编译后执行的方法,指令的dom操作就可以写在这里面
link:function(scope,element,attrs,ctrl){
//scope 基本就是上面写到的scope
//element 简单的就是指令html的dom,可用于dom操作,类似$(‘xxx’)
//attrs 是个map对象,内容就是指令上所有的属性
例:<my-student name=”name”></my-student>
即attrs={name:’name’}
}
replace:是否替换掉自定义的指令, 默认是false
可切换true/false,然后打开浏览器的开发者模式查看elements对比效果
transclude:指令中可以加入其它的html内容(可用于指令的嵌套)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<script type="text/javascript"
src="../../lib/angular-1.4.1/angular.js">
</script>
</head>
<body ng-app="myApp" ng-controller="myCtrl">
<div>
<my-student>
</my-student>
</div>
<script>
var app = angular.module("myApp", []);
app.controller("myCtrl",function($scope){
$scope.stu = {
name:'张三',
age:20
}
$scope.ageAdd = function(){
$scope.stu.age+=1;
}
$scope.ageCut = function(){
$scope.stu.age-=1;
}
})
app.directive("myStudent", function () {
return {
restrict: 'EA',
template: '<div><ng-transclude age-add></ng-transclude>姓名:{{stu.name}} 年龄:{{stu.age}}<ng-transclude class="age-cut"></ng-transclude></div>',
replace:true,
transclude:true
}
})
app.directive("ageAdd",function(){
return {
restrict:"EAC",
template:'<button ng-click="ageAdd()">年龄自增</button>'
}
})
app.directive("ageCut",function(){
return {
restrict:"EAC",
template:'<button ng-click="ageCut()">年龄自减</button>'
}
})
</script>
</body>
</html>
转自: https://blog.csdn.net/const_qiu/article/details/55049020