全局异常处理:
定义一个处理类,使用@ControllerAdvice注解。
@ControllerAdvice注解:控制器增强,一个被@Component注册的组件。
配合@ExceptionHandler来增强所有的@requestMapping方法。
例如:@ExceptionHandler(Exception.class) 用来捕获@requestMapping的方法中所有抛出的exception。
代码:
-
-
public class GlobalDefultExceptionHandler {
-
-
//声明要捕获的异常
-
-
-
public String defultExcepitonHandler(HttpServletRequest request,Exception e) {
-
return “error”;
-
}
-
}
这样,全局异常处理类完毕。可以添加自己的逻辑。
然后还有一个问题,有的时候,我们需要业务逻辑时抛出自定义异常,这个时候需要自定义业务异常类。
定义class:BusinessException ,使他继承于RuntimeException.
扫描二维码关注公众号,回复:
2035346 查看本文章
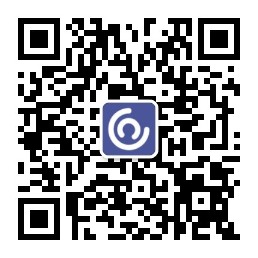
说明:因为某些业务需要进行业务回滚。但spring的事务只针对RuntimeException的进行回滚操作。所以需要回滚就要继承RuntimeException。
-
public class BusinessException extends RuntimeException{
-
-
}
然后,现在来稍微完善一下这个类。
当我们抛出一个业务异常,一般需要错误码和错误信息。有助于我们来定位问题。
所以如下:
-
public class BusinessException extends RuntimeException{
-
//自定义错误码
-
private Integer code;
-
//自定义构造器,只保留一个,让其必须输入错误码及内容
-
public BusinessException(int code,String msg) {
-
super(msg);
-
this.code = code;
-
}
-
-
public Integer getCode() {
-
return code;
-
}
-
-
public void setCode(Integer code) {
-
this.code = code;
-
}
-
}
这时候,我们发现还有一个问题,如果这样写,在代码多起来以后,很难管理这些业务异常和错误码之间的匹配。所以在优化一下。
把错误码及错误信息,组装起来统一管理。
定义一个业务异常的枚举。
-
public enum ResultEnum {
-
UNKONW_ERROR(- 1, "未知错误"),
-
SUCCESS( 0, "成功"),
-
ERROR( 1, "失败"),
-
;
-
-
private Integer code;
-
private String msg;
-
-
ResultEnum(Integer code,String msg) {
-
this.code = code;
-
this.msg = msg;
-
}
-
-
public Integer getCode() {
-
return code;
-
}
-
-
public String getMsg() {
-
return msg;
-
}
-
}
这个时候,业务异常类:
-
public class BusinessException extends RuntimeException{
-
-
private static final long serialVersionUID = 1L;
-
-
private Integer code; //错误码
-
-
public BusinessException() {}
-
-
public BusinessException(ResultEnum resultEnum) {
-
super(resultEnum.getMsg());
-
this.code = resultEnum.getCode();
-
}
-
-
public Integer getCode() {
-
return code;
-
}
-
-
public void setCode(Integer code) {
-
this.code = code;
-
}
-
}
然后再修改一下全局异常处理类:
-
-
public class GlobalDefultExceptionHandler {
-
-
//声明要捕获的异常
-
-
-
public <T> Result<?> defultExcepitonHandler(HttpServletRequest request,Exception e) {
-
e.printStackTrace();
-
if(e instanceof BusinessException) {
-
Log.error( this.getClass(), "业务异常:"+e.getMessage());
-
BusinessException businessException = (BusinessException)e;
-
return ResultUtil.error(businessException.getCode(), businessException.getMessage());
-
}
-
//未知错误
-
return ResultUtil.error(- 1, "系统异常:\\n"+e);
-
}
-
-
}