按键响应事件
KeyEvent
Rectangle
{
width: 300;
height: 200;
color: "#c0c0c0";
focus: true;
Keys.enab1ed:true;
Keys.onEscapePressed:Qt.quit();
Keys.onBackPressed: Qt.quit();
Keys.opPressed:
{
switch(event.key)
{
case Qt.Key_0:
case Qt.Key_1:
case Qt.Key_2:
case Qt.Key_3:
case Qt.Key_4:
case Qt.Key_5:
case Qt.Key_6:
case Qt.Key_7:
case Qt.Key_8:
case Qt.Key_9:
event.accepted = true;
keyview.text = event.key - Qt.Key_0;
break;
}
}
Text
{
id: keyView;
font.bold: true;
font. pixelIze: 24;
text: qsTr("text");
anchors.centerIn: parent; //显示在中间
}
}
结果:
Text
属性:font、text、color、elide、textFormat、wrapMode、horizontalAlignment、verticalAlignment等属性
wrapMode:
Text.NoWrap // 默认,不换行
Text: WordWrap // 在单词边界进行换行
Text.WrapAnywhere // 任意位置换行,不管单词
Text.Wrap // 尽可能在单词边界换行,否则任意点换行
style属性下的几种文字风格:Text.Raised、Text.Outline、Text.Sunken;// 使用方法style: Text.Sunken;
Button
类似于QPushButton
isDefault
:设置按钮是否为默认,如果为默认的话,按下Enter键就会触发clicked()信号
pressed
:保存按钮按下状态
menu
:允许给按钮设置一个菜单,单击弹出。默认null
action
:设置按钮的action。
activeFocusOnPress
:按钮按下是否获取焦点,默认false
ButtonStyle
有三种属性:background、control、label三个属性
Component
可以对同一类控件设置样式,使用时直接使用Component的ID即可,使用方法:style:Component_ID
image
Qt支持JPG、PNG、BMP、GIF、SVG。。。
fillMode
(图片填充方式):
Image.Stretch // 拉伸
Image.PreserveAspectFit // 等比缩放
Image.PreserveAspectCrop // 等比缩放,最大化填充 Image,必要时裁剪图片
Image.Tile // 在水平和垂直两个方向平铺,就像贴瓷砖那样
Image.TileVertically // 垂直平铺
Image.TileHorizontally // 水平平铺
Image.Pad // 保持图片原样不做变换
asynchronous
设置为true时,将开启异步加载模式,将开一个线程加载图片
Source
加载图片,根据URL,可以加载httpftp类型图片,此时自动开启异步模式
下面是一个网络加载的示例
Image
{
id: imageviewer;
asynchronous: true; // 异步加载
cache: false; // 不用缓存,反之亦然
anchors.fill: parent;
fillMode: Image.PreserveAspectFit;
onStatusChanged: // 信号处理器
{
if (imageViewer.status == Image.Loading)
{
busy.running = true;
statelabel.visible = false;
}
else if(imageViewer.status == Image.Ready)
busy.running = false;
else if(imageViewer.status == Image.Error)
{
busy.running = false;
statelabel.visible = true;
statelabel.text = "ERROR";
}
}
Component.unCompleted:
{
imageviewer.source = "http://image.cuncunle.com/images/editoriMages/2013/01/01/19/20130001194920468.JPG";
}
}
BusyIndicator显示等待图元,主要用于耗时操作
一个非常简单的图片浏览器
import QtQuick 2.2
import QtQuick.Window 2.1
import QtQuick.Controls 1.2
import QtQuick.Controls.Styles 1.2
import QtQuick.Dialogs 1.1
Window
{
visible: true;
width: 600;
height: 480;
minimumWidth: 480;
minimumHeight: 380;
BusyIndicator
{
id: busy;
running: false;
anchors.centerIn: parent;
z: 2;
}
Text
{
id: stateLabel;
visible: false;
anchors.centerIn: parent;
z: 3;
}
Image
{
id: imageViewer;
asynchronous: true;
cache: false;
anchors.fill: parent;
fillMode: Image.PreserveAspectFit;
onStatusChanged:
{
if (imageViewer.status == Image.Loading)
{
busy.running = true;
statelabel.visible = false;
}
else if(imageViewer.status == Image.Ready)
busy.running = false;
else if(imageViewer.status == Image.Error)
{
busy.running = false;
statelabel.visible = true;
statelabel.text = "ERROR";
}
}
}
Button
{
id: openFile;
text: "OPEN";
anchors.left: parent.left;
anchors.leftMargin: 8;
anchors.bottom: parent.bottom;
anchors.bottomMargin: 8;
style: ButtonStyle
{
background: Rectangle
{
implicitWidth:70;
implicitHeight: 25;
color: control.hovered ? "#c0c0c0":"#a0a0a0";
border.width: control.pressed ? 2 : 1;
border.color: (control.hovered || control.pressed) ? "green" : "#888888";
}
}
onClicked: fileDialog.open();
z: 4;
}
Text
{
id: imagePath;
anchors.left: openFile.right;
anchors.leftMargin: 8;
anchors.verticalCenter: openFile.verticalCenter;
font.pixelSize: 18;
}
FileDialog
{
id: fileDialog;
title: "Please choose a file";
nameFilters: ["Image Files(*.jpg *.png *.gif)"];
onAccepted:
{
imageViewer.source = fileDialog.fileUrl;
var imageFile = new String(fileDialog.fileUrl);
//remove file://
imagePath.text = imageFile.slice(8);
}
}
}
FilDialog 文件对话框,可选择已有文件、文件夹,支持单选、多选和Qt 普通工程下的作用一下
属性介绍:
visible
默认为false, 如果需要显示对话框,需要调用open方法或者设置为true;
selectExisting
默认true,表示选择已有文件或文件夹,当设置为false时为用户创建文件或者文件夹文字
selectFolder
默认false, 表示选择文件;设置为true时表示选择文件夹
selectMultiple
默认为false,表示单选,反之多选,当selectExisting为false时该属性也应为false
其他:
nameFilters
用于设定一个过滤列表,用来名字过滤。
selectNameFilter
用来保存选择的过滤器或者用来初始的过滤器
fileUrl
属性保存文件的路径。如果是多个文件时,fileUrls属性是一个列表,保存用户选择的所有文件路径
folder
属性存放的是用户选的(文件所在的)文件夹的位置
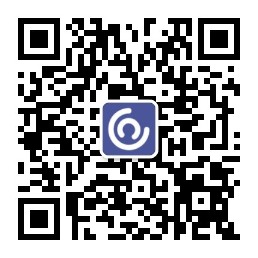