1.编码问题
# -*- coding:utf-8 -*-
PY文件当中是不支持中文的,即使你输入的注释是中文也不行,为了解决这个问题,就需要把文件编码类型改为UTF-8的类型,输入这个代码就可以让PY源文件里面有中文了。
建议你写代码之前都把这句话加上,因为不管是注释还是弹出消息提示,免不了的要输入中文,所以这个基本是必须的。
2.numpy
NumPy is the fundamental package for scientific computing with Python. It contains among other things:
*a powerful N-dimensional array object
*sophisticated (broadcasting) functions
*tools for integrating C/C++ and Fortran code
*useful linear algebra, Fourier transform, and random number capabilities
Besides its obvious scientific uses, NumPy can also be used as an efficient multi-dimensional container of generic data. Arbitrary data-types can be defined. This allows NumPy to seamlessly and speedily integrate with a wide variety of databases. [1]
Numpy 和 scikit-learn 都是python常用的第三方库。numpy库可以用来存储和处理大型矩阵,并且在一定程度上弥补了python在运算效率上的不足,正是因为numpy的存在使得python成为数值计算领域的一大利器;sklearn是python著名的机器学习库,它其中封装了大量的机器学习算法,内置了大量的公开数据集,并且拥有完善的文档,因此成为目前最受欢迎的机器学习学习与实践的工具 [2]。
3.python open 文件
f=open(‘/tmp/hello’,’w’)
#open(路径+文件名,读写模式)
#读写模式:r只读,r+读写,w新建(会覆盖原有文件),a追加,b二进制文件.常用模式
rU 或 Ua 以读方式打开, 同时提供通用换行符支持 (PEP 278)
w 以写方式打开,
a 以追加模式打开 (从 EOF 开始, 必要时创建新文件)
r+ 以读写模式打开
w+ 以读写模式打开 (参见 w )
a+ 以读写模式打开 (参见 a )
rb 以二进制读模式打开
wb 以二进制写模式打开 (参见 w )
ab 以二进制追加模式打开 (参见 a )
rb+ 以二进制读写模式打开 (参见 r+ )
wb+ 以二进制读写模式打开 (参见 w+ )
ab+ 以二进制读写模式打开 (参见 a+ ) [3]
注意:使用’W’,文件若存在,首先要清空,然后(重新)创建。
当你用二进制模式将带有换行符的字符串写入txt文件时,数据存储是正确的,但是当用windows平台的记事本程序打开时,你看到的换行符确是一个个的小黑块,但是,用文本模式,就不存在这样的问题。
4.numpy函数
mat函数可以将目标数据的类型转换为矩阵(matrix)
zeros函数是生成指定维数的全0数组
ones函数是用于生成一个全1的数组
.T作用于矩阵,用作球矩阵的转置
tolist函数用于把一个矩阵转化成为list列表
.I用作求矩阵的逆矩阵。逆矩阵在计算中是经常需要用到的。例如一个矩阵A,求A的逆矩阵B,即存在矩阵B是的AB=I(I为单位) [4]
Vectorize函数:对numpy数组中的每一个元素应用一个函数 [6], [7]
np.random.rand(3,2)则产生 3×2的数组,里面的数是0~1的浮点随机数
np.dot(A, B):对于二维矩阵,计算真正意义上的矩阵乘积,同线性代数中矩阵乘法的定义。对于一维矩阵,计算两者的内积。
实现对应元素相乘,有2种方式,一个是np.multiply(),另外一个是*。
np.loadtxt
作用是把文本文件(*.txt)读入并以矩阵或向量的形式输出。它有几个参数:skiprows,delimiter,usecoles和unpack。skiprows作用是跳过头行。在默认情况下是通过空格来分割列的,如果想通过其他来分割列则需要通过delimiter来设置。如果你想跳过几列来读取则需要用usecoles来设置。
json.loads()是用来读取字符串的,即,可以把文件打开,用readline()读取一行,然后json.loads()一行。
json.load()是用来读取文件的,即,将文件打开然后就可以直接读取。
在python中,要输出json格式,需要对json数据进行编码,要用到函数:json.dumps
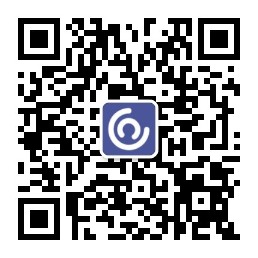
json.dumps() :是对数据进行编码
#coding=gbk
import json
dicts={"name":"lucy","sex":"boy"}
json_dicts=json.dumps(dicts)
print(json_dicts)
5.python类函数
私有方法 : 小写和一个前导下划线
def _secrete(self):
print “don’t test me.”
特殊方法 : 小写和两个前导下划线,两个后置下划线
def add(self, other):
return int.add(other)
这种风格只应用于特殊函数,比如操作符重载等。
函数参数 : 小写和下划线,缺省值等号两边无空格
def connect(self, user=None):
self._user = user
除特殊模块 init 之外,模块名称都使用不带下划线的小写字母。
变量:
1. 前带_的变量: 标明是一个私有变量, 只用于标明, 外部类还是可以访问到这个变量
2. 前带两个_ ,后带两个_ 的变量: 标明是内置变量,
3. 大写加下划线的变量: 标明是 不会发生改变的全局变量
函数:
1. 前带_的变量: 标明是一个私有函数, 只用于标明,
2. 前带两个_ ,后带两个_ 的函数: 标明是特殊函数 [5]
6. POST GET的区别
HTTP的底层是TCP/IP。所以GET和POST的底层也是TCP/IP,也就是说,GET/POST都是TCP链接。GET和POST能做的事情是一样一样的。你要给GET加上request body,给POST带上url参数,技术上是完全行的通的。
在我大万维网世界中,TCP就像汽车,我们用TCP来运输数据,它很可靠,从来不会发生丢件少件的现象。但是如果路上跑的全是看起来一模一样的汽车,那这个世界看起来是一团混乱,送急件的汽车可能被前面满载货物的汽车拦堵在路上,整个交通系统一定会瘫痪。为了避免这种情况发生,交通规则HTTP诞生了。HTTP给汽车运输设定了好几个服务类别,有GET, POST, PUT, DELETE等等,HTTP规定,当执行GET请求的时候,要给汽车贴上GET的标签(设置method为GET),而且要求把传送的数据放在车顶上(url中)以方便记录。如果是POST请求,就要在车上贴上POST的标签,并把货物放在车厢里。当然,你也可以在GET的时候往车厢内偷偷藏点货物,但是这是很不光彩;也可以在POST的时候在车顶上也放一些数据,让人觉得傻乎乎的。HTTP只是个行为准则,而TCP才是GET和POST怎么实现的基本。
在我大万维网世界中,还有另一个重要的角色:运输公司。不同的浏览器(发起http请求)和服务器(接受http请求)就是不同的运输公司。 虽然理论上,你可以在车顶上无限的堆货物(url中无限加参数)。但是运输公司可不傻,装货和卸货也是有很大成本的,他们会限制单次运输量来控制风险,数据量太大对浏览器和服务器都是很大负担。业界不成文的规定是,(大多数)浏览器通常都会限制url长度在2K个字节,而(大多数)服务器最多处理64K大小的url。超过的部分,恕不处理。如果你用GET服务,在request body偷偷藏了数据,不同服务器的处理方式也是不同的,有些服务器会帮你卸货,读出数据,有些服务器直接忽略,所以,虽然GET可以带request body,也不能保证一定能被接收到哦。
对于GET方式的请求,浏览器会把http header和data一并发送出去,服务器响应200(返回数据);
而对于POST,浏览器先发送header,服务器响应100 continue,浏览器再发送data,服务器响应200 ok(返回数据)。
也就是说,GET只需要汽车跑一趟就把货送到了,而POST得跑两趟,第一趟,先去和服务器打个招呼“嗨,我等下要送一批货来,你们打开门迎接我”,然后再回头把货送过去。
因为POST需要两步,时间上消耗的要多一点,看起来GET比POST更有效。因此Yahoo团队有推荐用GET替换POST来优化网站性能。但这是一个坑!跳入需谨慎。为什么?[8]
7. BaseHTTPServer
class BaseHTTPServer.HTTPServer(server_address, RequestHandlerClass)
This class builds on the TCPServer class by storing the server address as instance variables named server_name and server_port. The server is accessible by the handler, typically through the handler’s server instance variable.
class BaseHTTPServer.BaseHTTPRequestHandler(request, client_address, server)
This class is used to handle the HTTP requests that arrive at the server. By itself, it cannot respond to any actual HTTP requests; it must be subclassed to handle each request method (e.g. GET or POST). BaseHTTPRequestHandler provides a number of class and instance variables, and methods for use by subclasses.
The handler will parse the request and the headers, then call a method specific to the request type. The method name is constructed from the request. For example, for the request method SPAM, the do_SPAM() method will be called with no arguments. All of the relevant information is stored in instance variables of the handler. Subclasses should not need to override or extend the init() method.
A BaseHTTPRequestHandler instance has the following methods:
1)send_response(code[, message])
Sends a response header and logs the accepted request. The HTTP response line is sent, followed by Server and Date headers. The values for these two headers are picked up from the version_string() and date_time_string() methods, respectively.
2)send_header(keyword, value)
Writes a specific HTTP header to the output stream. keyword should specify the header keyword, with value specifying its value.
3)end_headers()
Sends a blank line, indicating the end of the HTTP headers in the response.
To create a server that doesn’t run forever, but until some condition is fulfilled:
def run_while_true(server_class=BaseHTTPServer.HTTPServer,
handler_class=BaseHTTPServer.BaseHTTPRequestHandler):
“””
This assumes that keep_running() is a function of no arguments which
is tested initially and after each request. If its return value
is true, the server continues.
“””
server_address = (”, 8000)
httpd = server_class(server_address, handler_class)
while keep_running():
httpd.handle_request()
[8]
参考文献
[1] NumPy. http://www.numpy.org/
[2] python常用库 - NumPy 和 sklearn入门. https://www.cnblogs.com/lianyingteng/p/7749609.html
[3] python open 文件操作. https://www.cnblogs.com/happySyk/p/8287976.html
[4] Python的numpy库下的几个小函数的用法. https://www.cnblogs.com/itdyb/p/5773404.html
[5] 关于python中带下划线的变量和函数的意义. https://www.cnblogs.com/wangshuyi/p/6096362.html
[6] Routines. https://docs.scipy.org/doc/numpy/reference/routines.html
[7] numpy教程:函数库和ufunc函数. https://blog.csdn.net/pipisorry/article/details/39235753
[8] 99%的人都理解错了HTTP中GET与POST的区别. http://www.techweb.com.cn/network/system/2016-10-11/2407736.shtml
[9] BaseHTTPServer — Basic HTTP server. https://docs.python.org/2/library/basehttpserver.html