1. 链队列含头结点模型示意图如下:
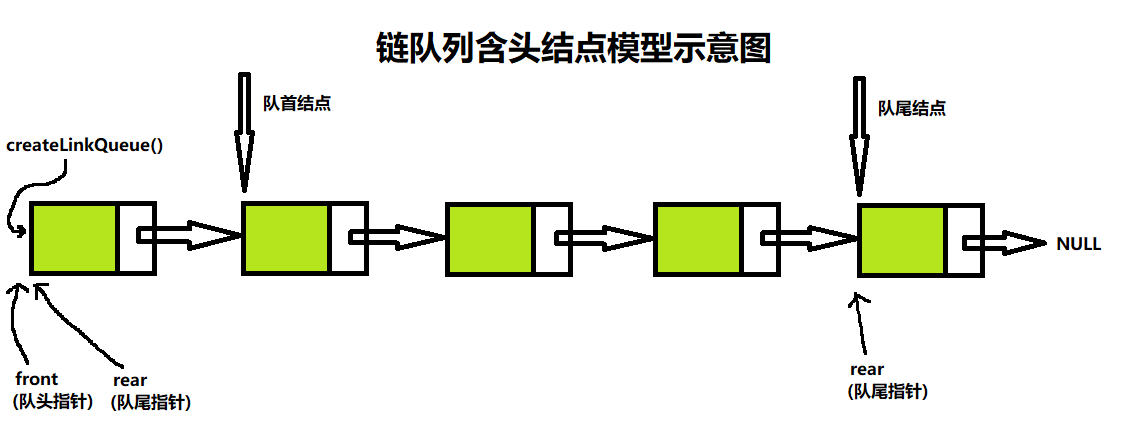
2. 链队列结构定义如下:
// 结点类型
struct QueueNode {
int data;
QueueNode* next;
};
// 链队列结构
struct LinkQueue{
QueueNode* front;
QueueNode* rear;
};
3. 链队列的基本操作函数如下:
- LinkQueue* createLinkQueue(); // 创建队列头结点
- Insert(LinkQueue* head, int item); // 插入
- Delete(LinkQueue* head); // 出队,并返回数据
4. 具体代码实现如下:
#include <iostream>
using namespace std;
// 结点类型
struct QueueNode {
int data;
QueueNode* next;
};
// 链队列结构
struct LinkQueue{
QueueNode* front;
QueueNode* rear;
};
// 创建队列头结点
LinkQueue* createLinkQueue() {
LinkQueue* head = (LinkQueue*)malloc(sizeof(LinkQueue));
head->front = (QueueNode*)malloc(sizeof(QueueNode));
head->front->data = 0;
head->front->next = NULL;
head->rear = head->front;
return head;
}
// 插入
void Insert(LinkQueue* head, int item) {
if (head == NULL) {
head = createLinkQueue();
}
QueueNode* qnode = (QueueNode*)malloc(sizeof(QueueNode));
qnode->data = item;
qnode->next = NULL;
head->rear->next = qnode;
head->rear = qnode;
}
// 删除
int Delete(LinkQueue* head) {
if (head == NULL || head->front == head->rear) {
cout << "The linkqueue is empty." << endl;
return 0;
}
QueueNode* temp = head->front->next;
int val = temp->data;
head->front->next = temp->next;
if (temp == head->rear) {
head->rear = head->front;
}
free(temp);
return val;
}
int main() {
LinkQueue* head = NULL;
head = createLinkQueue();
Insert(head, 1);
Insert(head, 2);
Insert(head, 3);
Insert(head, 4);
cout << Delete(head) << endl;
cout << Delete(head) << endl;
cout << Delete(head) << endl;
cout << Delete(head) << endl;
cout << Delete(head) << endl;
system("pause");
return 0;
}
5. 运行结果截图如下:
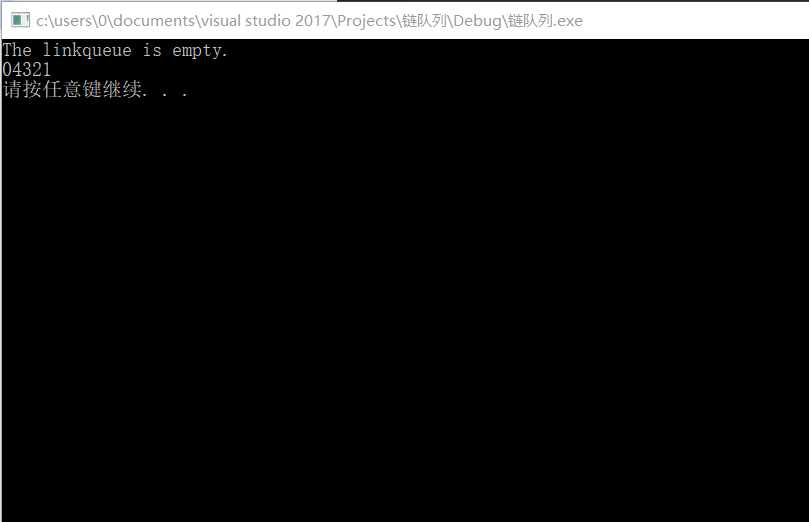